python五子棋人机对战 pygame
时间: 2023-11-21 21:55:55 浏览: 156
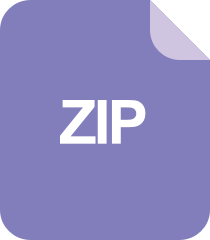
python 五子棋人机对战
以下是基于Python和pygame实现的五子棋人机对战游戏的介绍:
该游戏支持人机对战和人人对战两种模式,游戏初始界面会有5秒的规则介绍,五秒后自动进入人机对战模式。玩家可根据游戏中的提示按下Q键切换为初始人人对战对接,按下E键切换为初始人机对战界面。在游戏进行过程中,程序会记录并显示黑白两子的累计获胜局数,提高玩家体验。
如果您想了解更多关于该游戏的信息,可以参考以下两个引用:
引用:【源码】【G1】基于Python+pygame实现的人机AI对战五子棋游戏.zip-其他文档类资源-CSDN下载
引用:通过python的pygame库,实现一款支持人机对战和人人对战的五子棋小游戏。
```python
# 以下是一个简单的五子棋人机对战游戏的代码示例
import pygame
import sys
import random
# 初始化pygame
pygame.init()
# 设置游戏窗口大小
size = width, height = 640, 640
# 设置游戏窗口标题
pygame.display.set_caption("五子棋人机对战")
# 设置游戏窗口
screen = pygame.display.set_mode(size)
# 设置棋盘大小
board_size = 600
# 设置棋盘边距
margin = 20
# 设置棋盘格子大小
grid_size = board_size // 15
# 设置棋子半径
piece_radius = grid_size // 2 - 2
# 设置棋子颜色
black = (0, 0, 0)
white = (255, 255, 255)
# 设置棋盘背景颜色
board_color = (200, 150, 50)
# 设置棋盘线条颜色
line_color = (0, 0, 0)
# 设置字体
font = pygame.font.Font(None, 30)
# 设置游戏状态
game_over = False
# 设置棋盘数组
board = [[0] * 15 for i in range(15)]
# 设置棋子数量
piece_count = 0
# 设置玩家颜色
player_color = black
# 设置AI颜色
ai_color = white
# 设置AI难度
ai_level = 2
# 设置AI下棋时间间隔
ai_think_time = 1000
# 设置AI下棋位置
ai_pos = (0, 0)
# 设置获胜状态
win = 0
# 绘制棋盘
def draw_board():
# 绘制棋盘背景
screen.fill(board_color)
# 绘制棋盘线条
for i in range(15):
pygame.draw.line(screen, line_color, (margin, margin + i * grid_size), (margin + board_size, margin + i * grid_size), 2)
pygame.draw.line(screen, line_color, (margin + i * grid_size, margin), (margin + i * grid_size, margin + board_size), 2)
# 绘制星位
for i in [3, 7, 11]:
for j in [3, 7, 11]:
pygame.draw.circle(screen, line_color, (margin + i * grid_size, margin + j * grid_size), 5, 0)
# 绘制棋子
def draw_piece(x, y, color):
pygame.draw.circle(screen, color, (margin + x * grid_size, margin + y * grid_size), piece_radius, 0)
# 判断是否获胜
def check_win(x, y, color):
count = 0
# 判断横向是否获胜
for i in range(15):
if board[x][i] == color:
count += 1
else:
count = 0
if count == 5:
return True
count = 0
# 判断纵向是否获胜
for i in range(15):
if board[i][y] == color:
count += 1
else:
count = 0
if count == 5:
return True
count = 0
# 判断左上到右下是否获胜
for i in range(-4, 5):
if x + i < 0 or x + i > 14 or y + i < 0 or y + i > 14:
continue
if board[x + i][y + i] == color:
count += 1
else:
count = 0
if count == 5:
return True
count = 0
# 判断左下到右上是否获胜
for i in range(-4, 5):
if x + i < 0 or x + i > 14 or y - i < 0 or y - i > 14:
continue
if board[x + i][y - i] == color:
count += 1
else:
count = 0
if count == 5:
return True
return False
# 判断是否平局
def check_draw():
for i in range(15):
for j in range(15):
if board[i][j] == 0:
return False
return True
# AI下棋
def ai_move():
global ai_pos
max_score = -1
for i in range(15):
for j in range(15):
if board[i][j] == 0:
score = get_score(i, j, ai_color)
if score > max_score:
max_score = score
ai_pos = (i, j)
board[ai_pos[0]][ai_pos[1]] = ai_color
draw_piece(ai_pos[0], ai_pos[1], ai_color)
# 获取得分
def get_score(x, y, color):
score = 0
# 判断横向得分
count = 0
empty = 0
for i in range(15):
if board[x][i] == color:
count += 1
elif board[x][i] == 0:
empty += 1
else:
break
if count >= 5:
score += 10000
elif count == 4 and empty == 1:
score += 1000
elif count == 3 and empty == 2:
score += 100
elif count == 2 and empty == 3:
score += 10
# 判断纵向得分
count = 0
empty = 0
for i in range(15):
if board[i][y] == color:
count += 1
elif board[i][y] == 0:
empty += 1
else:
break
if count >= 5:
score += 10000
elif count == 4 and empty == 1:
score += 1000
elif count == 3 and empty == 2:
score += 100
elif count == 2 and empty == 3:
score += 10
# 判断左上到右下得分
count = 0
empty = 0
for i in range(-4, 5):
if x + i < 0 or x + i > 14 or y + i < 0 or y + i > 14:
continue
if board[x + i][y + i] == color:
count += 1
elif board[x + i][y + i] == 0:
empty += 1
else:
break
if count >= 5:
score += 10000
elif count == 4 and empty == 1:
score += 1000
elif count == 3 and empty == 2:
score += 100
elif count == 2 and empty == 3:
score += 10
# 判断左下到右上得分
count = 0
empty = 0
for i in range(-4, 5):
if x + i < 0 or x + i > 14 or y - i < 0 or y - i > 14:
continue
if board[x + i][y - i] == color:
count += 1
elif board[x + i][y - i] == 0:
empty += 1
else:
break
if count >= 5:
score += 10000
elif count == 4 and empty == 1:
score += 1000
elif count == 3 and empty == 2:
score += 100
elif count == 2 and empty == 3:
score += 10
return score
# 主循环
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN and event.button == 1:
if win != 0:
continue
x, y = event.pos
if x < margin or x > margin + board_size or y < margin or y > margin + board_size:
continue
i = (x - margin) // grid_size
j = (y - margin) // grid_size
if board[i][j] != 0:
continue
board[i][j] = player_color
draw_piece(i, j, player_color)
piece_count += 1
if check_win(i, j, player_color):
win = 1
text = font.render("黑棋获胜!", True, black)
screen.blit(text, (margin, margin + board_size + 10))
pygame.display.update()
continue
if check_draw():
win = 2
text = font.render("平局!", True, black)
screen.blit(text, (margin, margin + board_size + 10))
pygame.display.update()
continue
player_color, ai_color = ai_color, player_color
if ai_level > 0:
pygame.time.set_timer(pygame.USEREVENT, ai_think_time)
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
player_color, ai_color = ai_color, player_color
ai_level = 0
draw_board()
for i in range(15):
for j in range(15):
if board[i][j] == black:
draw_piece(i, j, black)
elif board[i][j] == white:
draw_piece(i, j, white)
pygame.display.update()
elif event.key == pygame.K_e:
player_color, ai_color = ai_color, player_color
ai_level = 2
pygame.time.set_timer(pygame.USEREVENT, ai_think_time)
draw_board()
for i in range(15):
for j in range(15):
if board[i][j] == black:
draw_piece(i, j, black)
elif board[i][j] == white:
draw_piece(i, j, white)
pygame.display.update()
elif event.type == pygame.USEREVENT:
ai_move()
piece_count += 1
if check_win(ai_pos[0], ai_pos[1], ai_color):
win = -1
text = font.render("白棋获胜!", True, black)
screen.blit(text, (margin, margin + board_size + 10))
pygame.display.update()
continue
if check_draw():
win = 2
text = font.render("平局!", True, black)
screen.blit(text, (margin, margin + board_size + 10))
pygame.display.update()
continue
player_color, ai_color = ai_color, player_color
pygame.time.set_timer(pygame.USEREVENT, 0)
# 绘制棋盘
draw_board()
# 绘制棋子
for i in range(15):
for j in range(15):
if board[i][j] == black:
draw_piece(i, j, black)
elif board[i][j] == white:
draw_piece(i, j, white)
# 判断游戏是否结束
if win != 0:
continue
# 更新屏幕
pygame.display.update()
# 相关问题:
--相关问题--:
阅读全文
相关推荐
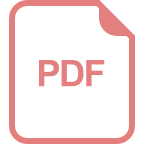
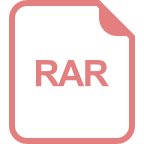
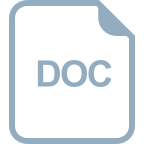

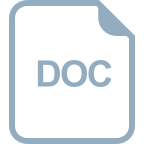
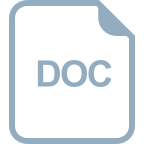
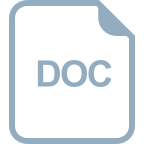
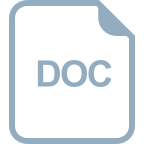
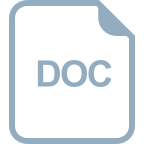







