python五子棋人机代码
时间: 2024-06-11 16:02:30 浏览: 24
Python五子棋的人机对战代码可以分为以下几个步骤:
1. 画出棋盘,确定棋子大小和棋盘大小。
2. 编写落子函数,包括判断胜负、判断落子是否合法、绘制棋子等。
3. 编写人类玩家和AI玩家,使得人类玩家可以通过鼠标点击棋盘进行落子,AI玩家根据算法自动落子。
4. 编写算法,实现AI玩家的自动落子。常用的算法有极大值算法(Minimax)、Alpha-Beta剪枝算法、蒙特卡罗树搜索等。
以下是一个简单的五子棋人机对战代码示例:
```python
import pygame
import random
# 棋盘参数
BOARD_SIZE = 15
CHESS_SIZE = 30
MARGIN = 40
WIDTH = CHESS_SIZE * BOARD_SIZE + MARGIN * 2
HEIGHT = CHESS_SIZE * BOARD_SIZE + MARGIN * 2
# 颜色定义
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
BLUE = (0, 0, 255)
GREEN = (0, 255, 0)
# 初始化Pygame
pygame.init()
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption("五子棋")
# 绘制棋盘
def draw_board():
for i in range(BOARD_SIZE):
pygame.draw.line(screen, BLACK, (MARGIN, MARGIN + i * CHESS_SIZE), (WIDTH - MARGIN, MARGIN + i * CHESS_SIZE), 1)
pygame.draw.line(screen, BLACK, (MARGIN + i * CHESS_SIZE, MARGIN), (MARGIN + i * CHESS_SIZE, HEIGHT - MARGIN), 1)
# 落子函数
def drop_chess(row, col, color):
x = MARGIN + col * CHESS_SIZE
y = MARGIN + row * CHESS_SIZE
pygame.draw.circle(screen, color, (x, y), CHESS_SIZE // 2)
# 判断胜负
def is_win(board, row, col):
# 判断横向是否有5个棋子相连
count = 1
left = col - 1
right = col + 1
while left >= 0 and board[row][left] == board[row][col]:
count += 1
left -= 1
while right < BOARD_SIZE and board[row][right] == board[row][col]:
count += 1
right += 1
if count >= 5:
return True
# 判断纵向是否有5个棋子相连
count = 1
top = row - 1
bottom = row + 1
while top >= 0 and board[top][col] == board[row][col]:
count += 1
top -= 1
while bottom < BOARD_SIZE and board[bottom][col] == board[row][col]:
count += 1
bottom += 1
if count >= 5:
return True
# 判断左上到右下斜线是否有5个棋子相连
count = 1
top = row - 1
left = col - 1
bottom = row + 1
right = col + 1
while top >= 0 and left >= 0 and board[top][left] == board[row][col]:
count += 1
top -= 1
left -= 1
while bottom < BOARD_SIZE and right < BOARD_SIZE and board[bottom][right] == board[row][col]:
count += 1
bottom += 1
right += 1
if count >= 5:
return True
# 判断左下到右上斜线是否有5个棋子相连
count = 1
top = row - 1
left = col + 1
bottom = row + 1
right = col - 1
while top >= 0 and right >= 0 and board[top][right] == board[row][col]:
count += 1
top -= 1
right -= 1
while bottom < BOARD_SIZE and left < BOARD_SIZE and board[bottom][left] == board[row][col]:
count += 1
bottom += 1
left += 1
if count >= 5:
return True
return False
# 判断落子是否合法
def is_valid_move(board, row, col):
if row < 0 or row >= BOARD_SIZE or col < 0 or col >= BOARD_SIZE:
return False
if board[row][col] != -1:
return False
return True
# AI玩家落子函数,随机落子一步棋
def ai_play(board):
while True:
row = random.randint(0, BOARD_SIZE - 1)
if is_valid_move(board, row, col):
return row, col
# 主程序循环
def main_loop():
# 初始化棋盘状态,-1表示空位,0表示黑方,1表示白方
board = [[-1] * BOARD_SIZE for _ in range(BOARD_SIZE)]
# 当前下棋方,0表示黑方,1表示白方
cur_player = random.randint(0, 1)
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
# 玩家下棋部分,通过鼠标点击实现落子操作
if event.type == pygame.MOUSEBUTTONDOWN and cur_player == 0:
x, y = event.pos, event.pos
if x < MARGIN or x > WIDTH - MARGIN or y < MARGIN or y > HEIGHT - MARGIN:
continue
col = (x - MARGIN) // CHESS_SIZE
row = (y - MARGIN) // CHESS_SIZE
if not is_valid_move(board, row, col):
continue
drop_chess(row, col, BLACK)
board[row][col] = cur_player
if is_win(board, row, col):
print("黑方获胜!")
return
cur_player = (cur_player + 1) % 2
# AI下棋部分,通过算法实现自动落子操作
if cur_player == 1:
row, col = ai_play(board)
drop_chess(row, col, WHITE)
board[row][col] = cur_player
if is_win(board, row, col):
print("白方获胜!")
return
cur_player = (cur_player + 1) % 2
# 绘制棋盘和棋子状态
screen.fill(WHITE)
draw_board()
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == 0:
drop_chess(i, j, BLACK)
elif board[i][j] == 1:
drop_chess(i, j, WHITE)
pygame.display.update()
if __name__ == '__main__':
main_loop()
```
相关推荐
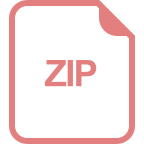
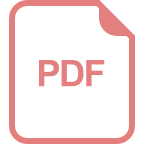
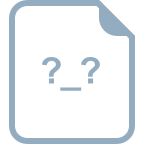












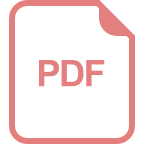