python五子棋人机对战源码IDLE
时间: 2023-06-18 16:03:16 浏览: 150
以下是使用Python编写的简单五子棋人机对战的源码,可以在IDLE中运行:
```python
import random
def draw_board(board):
# 绘制棋盘
HLINE = ' +---+---+---+---+---+---+---+'
VLINE = ' | | | | | | | |'
print(' 1 2 3 4 5 6 7')
print(HLINE)
for y in range(6):
print(VLINE)
print(y+1, end=' ')
for x in range(7):
print('| %s' % board[x][y], end=' ')
print('|')
print(VLINE)
print(HLINE)
def get_new_board():
# 创建新的棋盘
board = []
for x in range(7):
board.append([' ', ' ', ' ', ' ', ' ', ' '])
return board
def get_human_move(board):
# 获取玩家下棋的位置
while True:
move = input('请您输入下棋的位置(如:3,4):')
if move.isdigit() and len(move) == 3 and int(move[0]) in range(1, 8) and int(move[2]) in range(1, 7):
x = int(move[0]) - 1
y = int(move[2]) - 1
if board[x][y] == ' ':
return (x, y)
print('输入不合法,请重新输入!')
def get_computer_move(board, computer_tile):
# 获取电脑下棋的位置
possible_moves = []
for x in range(7):
for y in range(6):
if board[x][y] == ' ' and is_valid_move(board, x, y, computer_tile):
possible_moves.append((x, y))
if possible_moves:
return random.choice(possible_moves)
else:
return None
def is_valid_move(board, xstart, ystart, tile):
# 判断下棋的位置是否合法
if board[xstart][ystart] != ' ' or not is_on_board(xstart, ystart):
return False
board[xstart][ystart] = tile
if tile == 'X':
other_tile = 'O'
else:
other_tile = 'X'
tiles_to_flip = []
for xdir, ydir in [[0, 1], [1, 1], [1, 0], [1, -1], [0, -1], [-1, -1], [-1, 0], [-1, 1]]:
x, y = xstart, ystart
x += xdir
y += ydir
if is_on_board(x, y) and board[x][y] == other_tile:
x += xdir
y += ydir
if not is_on_board(x, y):
continue
while board[x][y] == other_tile:
x += xdir
y += ydir
if not is_on_board(x, y):
break
if not is_on_board(x, y):
continue
if board[x][y] == tile:
while True:
x -= xdir
y -= ydir
if x == xstart and y == ystart:
break
tiles_to_flip.append((x, y))
board[xstart][ystart] = ' '
if len(tiles_to_flip) == 0:
return False
return tiles_to_flip
def is_on_board(x, y):
# 判断位置是否在棋盘内
return x >= 0 and x <= 6 and y >= 0 and y <= 5
def make_move(board, tile, xstart, ystart, real_move=False):
# 下棋
tiles_to_flip = is_valid_move(board, xstart, ystart, tile)
if not tiles_to_flip:
return False
board[xstart][ystart] = tile
if real_move:
animate_tile_change(tiles_to_flip, tile, (xstart, ystart))
for x, y in tiles_to_flip:
board[x][y] = tile
if real_move:
animate_tile_change([(x, y)], tile, (x, y))
return True
def animate_tile_change(tiles_to_flip, tile, direction):
# 绘制下棋的动画效果
pass
def get_score_of_board(board):
# 计算棋盘的得分
xscore = 0
oscore = 0
for x in range(7):
for y in range(6):
if board[x][y] == 'X':
xscore += 1
if board[x][y] == 'O':
oscore += 1
return {'X': xscore, 'O': oscore}
def print_score(board, player_tile, computer_tile):
# 打印得分
scores = get_score_of_board(board)
print('您的得分:%s。电脑的得分:%s。' % (scores[player_tile], scores[computer_tile]))
def play_game(player_tile, computer_tile):
# 开始游戏
print('五子棋游戏开始了!')
board = get_new_board()
draw_board(board)
while True:
if player_tile == 'X':
x, y = get_human_move(board)
make_move(board, player_tile, x, y, True)
if is_game_over(board):
break
x, y = get_computer_move(board, computer_tile)
make_move(board, computer_tile, x, y, True)
if is_game_over(board):
break
else:
x, y = get_computer_move(board, computer_tile)
make_move(board, computer_tile, x, y, True)
if is_game_over(board):
break
x, y = get_human_move(board)
make_move(board, player_tile, x, y, True)
if is_game_over(board):
break
draw_board(board)
scores = get_score_of_board(board)
if scores[player_tile] > scores[computer_tile]:
print('恭喜您,您赢了!')
elif scores[player_tile] < scores[computer_tile]:
print('很遗憾,您输了!')
else:
print('平局!')
print_score(board, player_tile, computer_tile)
def is_game_over(board):
# 判断游戏是否结束
for x in range(7):
for y in range(6):
if board[x][y] == ' ':
return False
return True
def main():
# 主函数
player_tile = ' '
computer_tile = ' '
while player_tile not in ['X', 'O']:
player_tile = input('请选择您要使用的棋子(X或O):').upper()
if player_tile == 'X':
computer_tile = 'O'
else:
computer_tile = 'X'
play_game(player_tile, computer_tile)
if __name__ == '__main__':
main()
```
注意:该代码只是一个简单的实现,对于游戏的交互、AI策略等方面还有很多可以改进的地方。
阅读全文
相关推荐
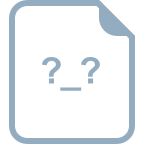
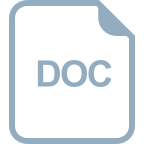

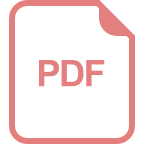
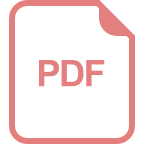
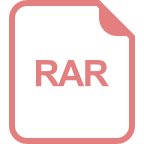
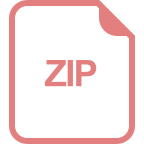
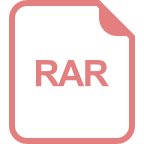
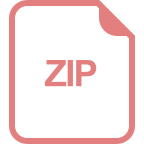
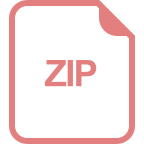
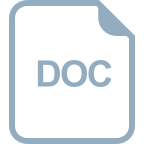
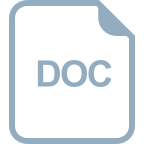
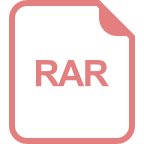
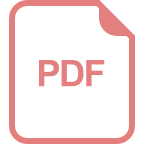
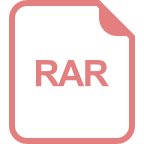