python设计五子棋代码人机对战
时间: 2024-02-02 16:03:39 浏览: 108
为了实现五子棋的人机对战,需要实现以下几个步骤:
1. 画出棋盘:使用Pygame库画出一个19×19或15×15的棋盘。
2. 实现落子:使用鼠标点击棋盘上的空白位置,将黑子或白子落在该位置。
3. 实现判断胜负:每次落子后,需要判断是否有一方获胜。可以通过判断横向、纵向、斜向是否有五个连续的同色棋子来判断胜负。
4. 实现人机对战:可以使用Minimax算法实现人机对战。该算法是一种博弈树搜索算法,通过搜索所有可能的落子情况,找到最优的落子位置。
下面是一个简单的Python代码实现五子棋的人机对战:
```python
import pygame
import sys
import random
# 初始化Pygame
pygame.init()
# 设置棋盘大小
BOARD_SIZE = 15
CELL_SIZE = 40
GRID_SIZE = CELL_SIZE * (BOARD_SIZE - 1)
SCREEN_SIZE = (GRID_SIZE + CELL_SIZE, GRID_SIZE + CELL_SIZE)
# 设置颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
GRAY = (200, 200, 200)
# 初始化屏幕
screen = pygame.display.set_mode(SCREEN_SIZE)
pygame.display.set_caption('五子棋')
# 绘制棋盘
def draw_board():
for i in range(BOARD_SIZE):
pygame.draw.line(screen, BLACK, (CELL_SIZE, CELL_SIZE*i+CELL_SIZE), (GRID_SIZE+CELL_SIZE, CELL_SIZE*i+CELL_SIZE), 2)
pygame.draw.line(screen, BLACK, (CELL_SIZE*i+CELL_SIZE, CELL_SIZE), (CELL_SIZE*i+CELL_SIZE, GRID_SIZE+CELL_SIZE), 2)
pygame.display.update()
# 落子
def drop_piece(board, row, col, piece):
board[row][col] = piece
# 判断胜负
def is_winner(board, piece):
# 判断横向是否有五个连续的同色棋子
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE-4):
if board[i][j] == piece and board[i][j+1] == piece and board[i][j+2] == piece and board[i][j+3] == piece and board[i][j+4] == piece:
return True
# 判断纵向是否有五个连续的同色棋子
for i in range(BOARD_SIZE-4):
for j in range(BOARD_SIZE):
if board[i][j] == piece and board[i+1][j] == piece and board[i+2][j] == piece and board[i+3][j] == piece and board[i+4][j] == piece:
return True
# 判断斜向是否有五个连续的同色棋子
for i in range(BOARD_SIZE-4):
for j in range(BOARD_SIZE-4):
if board[i][j] == piece and board[i+1][j+1] == piece and board[i+2][j+2] == piece and board[i+3][j+3] == piece and board[i+4][j+4] == piece:
return True
if board[i][j+4] == piece and board[i+1][j+3] == piece and board[i+2][j+2] == piece and board[i+3][j+1] == piece and board[i+4][j] == piece:
return True
return False
# Minimax算法
def minimax(board, depth, alpha, beta, maximizingPlayer):
if depth == 0 or is_winner(board, 'B') or is_winner(board, 'W'):
return evaluate(board)
if maximizingPlayer:
maxEval = -float('inf')
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == '':
board[i][j] = 'B'
eval = minimax(board, depth-1, alpha, beta, False)
board[i][j] = ''
maxEval = max(maxEval, eval)
alpha = max(alpha, eval)
if beta <= alpha:
break
return maxEval
else:
minEval = float('inf')
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == '':
board[i][j] = 'W'
eval = minimax(board, depth-1, alpha, beta, True)
board[i][j] = ''
minEval = min(minEval, eval)
beta = min(beta, eval)
if beta <= alpha:
break
return minEval
# 评估函数
def evaluate(board):
score = 0
# 判断横向是否有连续的同色棋子
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE-4):
if board[i][j] == 'B' and board[i][j+1] == 'B' and board[i][j+2] == 'B' and board[i][j+3] == 'B' and board[i][j+4] == 'B':
score += 100
elif board[i][j] == 'W' and board[i][j+1] == 'W' and board[i][j+2] == 'W' and board[i][j+3] == 'W' and board[i][j+4] == 'W':
score -= 100
# 判断纵向是否有连续的同色棋子
for i in range(BOARD_SIZE-4):
for j in range(BOARD_SIZE):
if board[i][j] == 'B' and board[i+1][j] == 'B' and board[i+2][j] == 'B' and board[i+3][j] == 'B' and board[i+4][j] == 'B':
score += 100
elif board[i][j] == 'W' and board[i+1][j] == 'W' and board[i+2][j] == 'W' and board[i+3][j] == 'W' and board[i+4][j] == 'W':
score -= 100
# 判断斜向是否有连续的同色棋子
for i in range(BOARD_SIZE-4):
for j in range(BOARD_SIZE-4):
if board[i][j] == 'B' and board[i+1][j+1] == 'B' and board[i+2][j+2] == 'B' and board[i+3][j+3] == 'B' and board[i+4][j+4] == 'B':
score += 100
elif board[i][j] == 'W' and board[i+1][j+1] == 'W' and board[i+2][j+2] == 'W' and board[i+3][j+3] == 'W' and board[i+4][j+4] == 'W':
score -= 100
if board[i][j+4] == 'B' and board[i+1][j+3] == 'B' and board[i+2][j+2] == 'B' and board[i+3][j+1] == 'B' and board[i+4][j] == 'B':
score += 100
elif board[i][j+4] == 'W' and board[i+1][j+3] == 'W' and board[i+2][j+2] == 'W' and board[i+3][j+1] == 'W' and board[i+4][j] == 'W':
score -= 100
return score
# 电脑落子
def computer_move(board):
bestScore = -float('inf')
bestMove = None
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == '':
board[i][j] = 'B'
score = minimax(board, 3, -float('inf'), float('inf'), False)
board[i][j] = ''
if score > bestScore:
bestScore = score
bestMove = (i, j)
return bestMove
# 初始化棋盘
board = [['' for i in range(BOARD_SIZE)] for j in range(BOARD_SIZE)]
# 游戏循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
sys.exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
if event.button == 1:
x, y = event.pos
row = round((y - CELL_SIZE) / CELL_SIZE)
col = round((x - CELL_SIZE) / CELL_SIZE)
if row >= 0 and row < BOARD_SIZE and col >= 0 and col < BOARD_SIZE and board[row][col] == '':
drop_piece(board, row, col, 'W')
if is_winner(board, 'W'):
print('你赢了!')
sys.exit()
row, col = computer_move(board)
drop_piece(board, row, col, 'B')
if is_winner(board, 'B'):
print('电脑赢了!')
sys.exit()
# 绘制棋盘和棋子
screen.fill(GRAY)
draw_board()
for i in range(BOARD_SIZE):
for j in range(BOARD_SIZE):
if board[i][j] == 'W':
pygame.draw.circle(screen, WHITE, (j*CELL_SIZE+CELL_SIZE, i*CELL_SIZE+CELL_SIZE), CELL_SIZE//2-2)
elif board[i][j] == 'B':
pygame.draw.circle(screen, BLACK, (j*CELL_SIZE+CELL_SIZE, i*CELL_SIZE+CELL_SIZE), CELL_SIZE//2-2)
pygame.display.update()
```
阅读全文
相关推荐
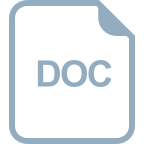
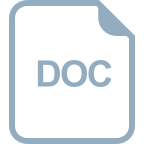
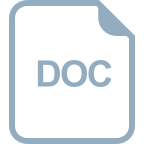
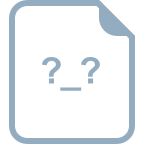
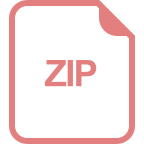
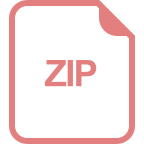



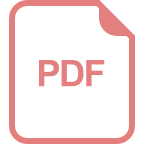
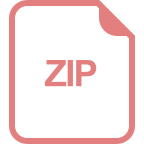




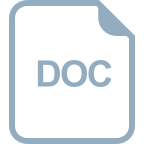
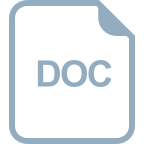
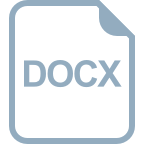