Python的类和对象实例
时间: 2024-12-08 19:11:41 浏览: 10
在Python中,类和对象实例是面向对象编程的核心概念。类是一种抽象的模板,用于定义对象的属性和方法,而对象实例则是类的具体实现。以下是一些关键点:
1. **定义类**:
使用`class`关键字定义一个类。类中可以包含属性和方法。
```python
class Person:
def __init__(self, name, age):
self.name = name
self.age = age
def greet(self):
print(f"Hello, my name is {self.name} and I am {self.age} years old.")
```
2. **创建对象实例**:
通过调用类名并传入必要的参数来创建对象实例。
```python
person1 = Person("Alice", 30)
person2 = Person("Bob", 25)
```
3. **访问属性和方法**:
可以通过对象实例访问类的属性和方法。
```python
print(person1.name) # 输出: Alice
person1.greet() # 输出: Hello, my name is Alice and I am 30 years old.
```
4. **继承**:
一个类可以继承另一个类的属性和方法。
```python
class Employee(Person):
def __init__(self, name, age, employee_id):
super().__init__(name, age)
self.employee_id = employee_id
def work(self):
print(f"{self.name} is working with employee id {self.employee_id}.")
employee1 = Employee("Charlie", 40, 12345)
employee1.greet() # 输出: Hello, my name is Charlie and I am 40 years old.
employee1.work() # 输出: Charlie is working with employee id 12345.
```
5. **多态**:
通过继承和方法重写实现多态。
```python
class Animal:
def speak(self):
pass
class Dog(Animal):
def speak(self):
print("Woof!")
class Cat(Animal):
def speak(self):
print("Meow!")
def animal_speak(animal):
animal.speak()
dog = Dog()
cat = Cat()
animal_speak(dog) # 输出: Woof!
animal_speak(cat) # 输出: Meow!
```
通过这些例子,可以看到Python中类和对象实例的强大功能和灵活性。
阅读全文
相关推荐
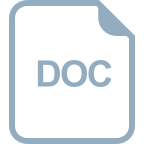
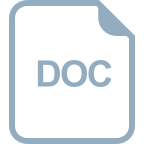
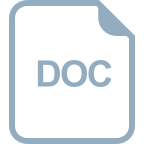
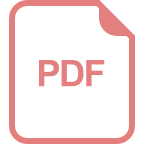
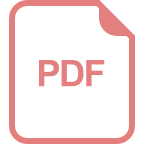
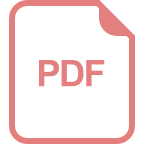
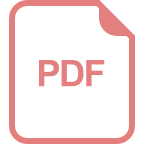
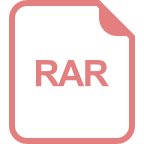
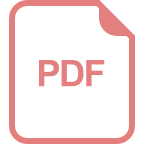
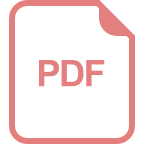
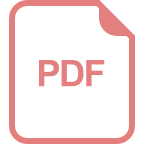
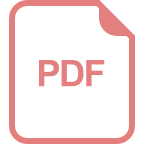
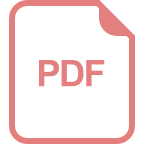
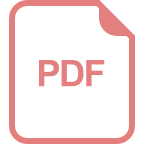
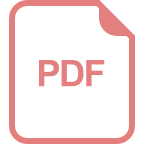
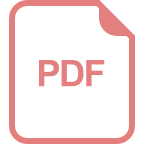
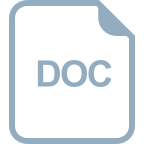
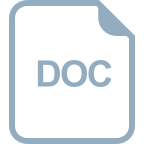