model = resnet18().cpu() model.load_state_dict(torch.load(file_path))
时间: 2024-06-02 17:13:04 浏览: 10
这段代码的作用是从指定路径 `file_path` 加载预训练的 ResNet-18 模型的权重参数,并将模型放到 CPU 上运行。其中 `resnet18()` 是一个函数,用于创建一个预训练的 ResNet-18 模型。`load_state_dict()` 方法是 PyTorch 中用于加载模型权重的函数。
相关问题
weights_path = "./resNet50.pth" assert os.path.exists(weights_path), f"file: '{weights_path}' dose not exist." model.load_state_dict(torch.load(weights_path, map_location=device))
这段代码加载了预训练的ResNet-50模型的权重,并将这些权重应用到之前创建的模型`model`中。具体地,这段代码首先定义了一个变量`weights_path`,表示预训练的模型权重文件的路径。然后,代码使用`assert`语句来判断该文件是否存在,如果文件不存在,则会抛出一个异常,异常信息为`file: '{weights_path}' dose not exist.`。
如果文件存在,则程序会继续执行后面的代码。这段代码使用了`torch.load`函数从`weights_path`中加载预训练的模型权重,并使用`model.load_state_dict`函数将这些权重应用到`model`中。需要注意的是,`map_location`参数用于指定模型应该被加载到哪个设备上,如果不指定该参数,则模型会被加载到CPU上,而不是GPU上。
需要注意的是,预训练模型的权重文件通常非常大,下载和加载这些文件可能会消耗大量的时间和计算资源。因此,在使用这段代码之前,需要确保已经下载了预训练模型的权重文件,并将其保存在`weights_path`所表示的路径中。
为以下每句代码做注释:device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu") data_transform = transforms.Compose( [transforms.Resize(256), transforms.CenterCrop(224), transforms.ToTensor(), transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])]) img = Image.open("./huanglongbing.JPG") plt.imshow(img) img = data_transform(img) img = torch.unsqueeze(img, dim=0) try: json_file = open('./class_indices.json', 'r') class_indict = json.load(json_file) except Exception as e: print(e) exit(-1) model = resnet152(num_classes=38) model_weight_path = "./resNet152.pth" model.load_state_dict(torch.load(model_weight_path, map_location=device)) model.eval() with torch.no_grad(): output = torch.squeeze(model(img)) predict = torch.softmax(output, dim=0) predict_cla = torch.argmax(predict).numpy() print(class_indict[str(predict_cla)], predict[predict_cla].numpy()) plt.show()
# 设备选择:如果有可用的cuda设备,则使用cuda:0,否则使用cpu
device = torch.device("cuda:0" if torch.cuda.is_available() else "cpu")
# 数据变换操作,包括图像大小调整、中心裁剪、转换为张量、归一化等
data_transform = transforms.Compose(
[transforms.Resize(256),
transforms.CenterCrop(224),
transforms.ToTensor(),
transforms.Normalize([0.485, 0.456, 0.406], [0.229, 0.224, 0.225])]
)
# 打开图像文件,显示图像
img = Image.open("./huanglongbing.JPG")
plt.imshow(img)
# 对图像进行数据变换
img = data_transform(img)
img = torch.unsqueeze(img, dim=0)
# 读取类别标签与索引的对应关系
try:
json_file = open('./class_indices.json', 'r')
class_indict = json.load(json_file)
except Exception as e:
print(e)
exit(-1)
# 加载预训练的resnet152模型,并载入预训练权重
model = resnet152(num_classes=38)
model_weight_path = "./resNet152.pth"
model.load_state_dict(torch.load(model_weight_path, map_location=device))
model.eval()
# 使用载入的模型进行推理,并输出预测结果
with torch.no_grad():
output = torch.squeeze(model(img))
predict = torch.softmax(output, dim=0)
predict_cla = torch.argmax(predict).numpy()
print(class_indict[str(predict_cla)], predict[predict_cla].numpy())
plt.show()
相关推荐









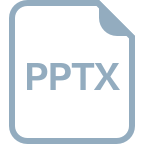
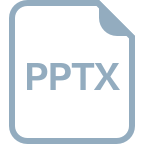