C++函数指针用来在数据格式fp_t为float时用cublasSaxpy,为double时用cublasDaxpy
时间: 2023-06-13 19:08:53 浏览: 95
可以使用函数指针来根据数据类型的不同来调用不同的函数。下面是一个示例代码:
```c++
#include <cublas_v2.h>
// 声明函数指针类型
typedef cublasStatus_t (*axpy_func_t)(cublasHandle_t handle, int n, const float* alpha,
const float* x, int incx, float* y, int incy);
// float 版本
cublasStatus_t saxpy(cublasHandle_t handle, int n, const float* alpha,
const float* x, int incx, float* y, int incy) {
return cublasSaxpy(handle, n, alpha, x, incx, y, incy);
}
// double 版本
cublasStatus_t daxpy(cublasHandle_t handle, int n, const float* alpha,
const float* x, int incx, float* y, int incy) {
return cublasDaxpy(handle, n, alpha, x, incx, y, incy);
}
// 根据数据类型选择使用的函数
void axpy(axpy_func_t func, cublasHandle_t handle, int n, const void* alpha,
const void* x, int incx, void* y, int incy) {
if (sizeof(*alpha) == sizeof(float)) {
func(handle, n, (const float*)alpha, (const float*)x, incx, (float*)y, incy);
} else if (sizeof(*alpha) == sizeof(double)) {
func(handle, n, (const float*)alpha, (const float*)x, incx, (float*)y, incy);
} else {
// 处理其他类型的数据
}
}
int main() {
cublasHandle_t handle;
cublasCreate(&handle);
float alpha = 2.0f;
float x[] = {1.0f, 2.0f, 3.0f};
float y[] = {4.0f, 5.0f, 6.0f};
// 调用 float 版本的 cublasSaxpy
axpy(saxpy, handle, 3, &alpha, x, 1, y, 1);
double dalpha = 2.0;
double dx[] = {1.0, 2.0, 3.0};
double dy[] = {4.0, 5.0, 6.0};
// 调用 double 版本的 cublasDaxpy
axpy(daxpy, handle, 3, &dalpha, dx, 1, dy, 1);
cublasDestroy(handle);
return 0;
}
```
在上面的代码中,`axpy` 函数接受一个函数指针 `func` 和其他参数,根据 `alpha` 的数据类型来选择使用 `saxpy` 或 `daxpy` 函数。这里的 `axpy_func_t` 类型是定义的函数指针类型,它是一个指向 `cublasSaxpy` 或 `cublasDaxpy` 函数的指针类型。
相关推荐
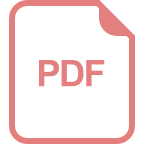
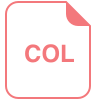
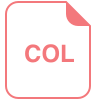
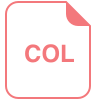
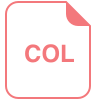
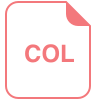









