使用java swing 编写一款贪吃蛇游戏
时间: 2023-07-20 22:31:52 浏览: 120
好的,这是一个基础版的贪吃蛇游戏的代码,使用Java Swing编写,其中包括游戏面板、蛇的移动控制、食物的生成等功能。你可以在此基础上进行改进,添加更多的功能。
```java
import java.awt.Color;
import java.awt.Dimension;
import java.awt.Graphics;
import java.awt.Point;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.awt.event.KeyEvent;
import java.awt.event.KeyListener;
import java.util.LinkedList;
import java.util.Random;
import javax.swing.JFrame;
import javax.swing.JPanel;
import javax.swing.Timer;
public class SnakeGame extends JPanel implements ActionListener, KeyListener {
private static final long serialVersionUID = 1L;
private static final int WIDTH = 500;
private static final int HEIGHT = 500;
private static final int SIZE = 10;
private static final int MAX_LENGTH = (WIDTH / SIZE) * (HEIGHT / SIZE) - 1;
private static final int DELAY = 100;
private static final int UP = 0, DOWN = 1, LEFT = 2, RIGHT = 3;
private LinkedList<Point> snake;
private Point food;
private int direction;
private boolean gameOver;
private Timer timer;
private Random random;
public SnakeGame() {
setPreferredSize(new Dimension(WIDTH, HEIGHT));
setBackground(Color.BLACK);
setFocusable(true);
addKeyListener(this);
snake = new LinkedList<Point>();
snake.add(new Point(WIDTH / 2, HEIGHT / 2));
food = new Point();
random = new Random();
newFood();
direction = -1;
gameOver = false;
timer = new Timer(DELAY, this);
timer.start();
}
public static void main(String[] args) {
JFrame frame = new JFrame("Snake Game");
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
frame.setResizable(false);
frame.add(new SnakeGame());
frame.pack();
frame.setLocationRelativeTo(null);
frame.setVisible(true);
}
@Override
public void paintComponent(Graphics g) {
super.paintComponent(g);
if (gameOver) {
g.setColor(Color.WHITE);
g.drawString("Game Over", WIDTH / 2 - 25, HEIGHT / 2);
return;
}
g.setColor(Color.RED);
g.fillOval(food.x, food.y, SIZE, SIZE);
g.setColor(Color.GREEN);
for (Point p : snake) {
g.fillOval(p.x, p.y, SIZE, SIZE);
}
}
@Override
public void actionPerformed(ActionEvent e) {
if (gameOver) {
return;
}
move();
checkCollision();
checkFood();
repaint();
}
private void move() {
Point head = snake.getFirst();
Point next = new Point();
switch (direction) {
case UP:
next.setLocation(head.x, head.y - SIZE);
break;
case DOWN:
next.setLocation(head.x, head.y + SIZE);
break;
case LEFT:
next.setLocation(head.x - SIZE, head.y);
break;
case RIGHT:
next.setLocation(head.x + SIZE, head.y);
break;
}
snake.addFirst(next);
snake.removeLast();
}
private void checkCollision() {
Point head = snake.getFirst();
if (head.x < 0 || head.x >= WIDTH || head.y < 0 || head.y >= HEIGHT) {
gameOver = true;
return;
}
for (Point p : snake.subList(1, snake.size())) {
if (head.equals(p)) {
gameOver = true;
return;
}
}
}
private void checkFood() {
if (snake.getFirst().equals(food)) {
newFood();
if (snake.size() == MAX_LENGTH) {
gameOver = true;
return;
}
} else {
snake.removeLast();
}
}
private void newFood() {
int x = random.nextInt(WIDTH / SIZE) * SIZE;
int y = random.nextInt(HEIGHT / SIZE) * SIZE;
food.setLocation(x, y);
for (Point p : snake) {
if (food.equals(p)) {
newFood();
return;
}
}
}
@Override
public void keyPressed(KeyEvent e) {
int code = e.getKeyCode();
if (code == KeyEvent.VK_UP && direction != DOWN) {
direction = UP;
} else if (code == KeyEvent.VK_DOWN && direction != UP) {
direction = DOWN;
} else if (code == KeyEvent.VK_LEFT && direction != RIGHT) {
direction = LEFT;
} else if (code == KeyEvent.VK_RIGHT && direction != LEFT) {
direction = RIGHT;
}
}
@Override
public void keyTyped(KeyEvent e) {
}
@Override
public void keyReleased(KeyEvent e) {
}
}
```
阅读全文
相关推荐
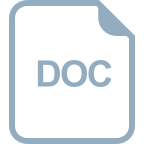
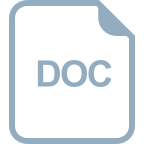
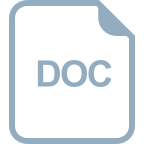

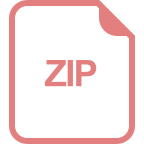
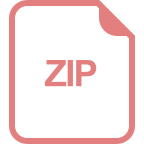



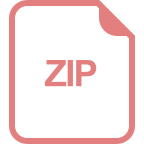
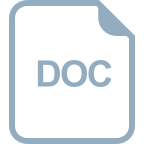
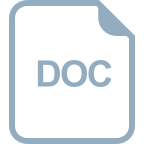
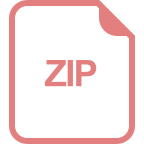
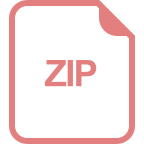
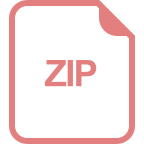
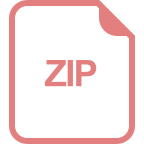
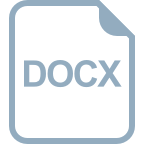
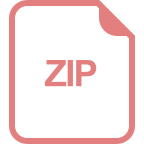