编写以下代码 用java swing做一款贪吃蛇游戏,要求有不同关卡
时间: 2024-03-08 07:51:05 浏览: 110
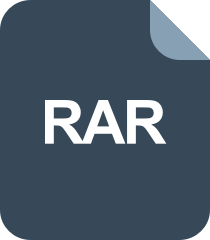
基于java-swing的贪吃蛇游戏
好的,下面是一个简单的Java Swing贪吃蛇游戏代码,其中包含有三个不同的关卡。这里提供的代码只是一个简单的示例,您可以根据自己的需要进行修改和扩展。
```java
import java.awt.*;
import java.awt.event.*;
import javax.swing.*;
public class SnakeGame extends JFrame implements KeyListener {
private static final long serialVersionUID = 1L;
private final int ROWS = 20;
private final int COLS = 20;
private final int BLOCK_SIZE = 20;
private final int MAX_LEVEL = 3;
private int[][][] levels = {
// level 1
{
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0}
},
// level 2
{
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 1, 1, 1, 1, 1, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 1, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 1, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 1, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 1, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 1, 0, 0, 0}
},
// level 3
{
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 1, 1, 1, 1, 1, 1, 1, 0},
{0, 0, 1, 0, 0, 0, 0, 0, 1, 0},
{0, 0, 1, 0, 0, 0, 0, 0, 1, 0},
{0, 0, 1, 0, 0, 0, 0, 0, 1, 0},
{0, 0, 1, 0, 0, 0, 0, 0, 1, 0},
{0, 0, 1, 1, 1, 1, 1, 1, 1, 0}
}
};
private int[][] level = levels[0];
private boolean[][] blocks = new boolean[ROWS][COLS];
private int[] snakeX = new int[ROWS * COLS];
private int[] snakeY = new int[ROWS * COLS];
private int snakeLength = 0;
private int foodX = 0;
private int foodY = 0;
private int direction = 0;
private int levelIndex = 0;
private int score = 0;
public static void main(String[] args) {
new SnakeGame();
}
public SnakeGame() {
setTitle("Snake Game");
setSize(COLS * BLOCK_SIZE + 20, ROWS * BLOCK_SIZE + 70);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setResizable(false);
setLayout(null);
JButton levelButton = new JButton("Level 1");
levelButton.setBounds(10, 10, 80, 20);
levelButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
levelIndex = 0;
level = levels[levelIndex];
resetGame();
}
});
add(levelButton);
JButton levelButton2 = new JButton("Level 2");
levelButton2.setBounds(100, 10, 80, 20);
levelButton2.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
levelIndex = 1;
level = levels[levelIndex];
resetGame();
}
});
add(levelButton2);
JButton levelButton3 = new JButton("Level 3");
levelButton3.setBounds(190, 10, 80, 20);
levelButton3.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
levelIndex = 2;
level = levels[levelIndex];
resetGame();
}
});
add(levelButton3);
setVisible(true);
addKeyListener(this);
setFocusable(true);
resetGame();
startGame();
}
private void resetGame() {
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
blocks[i][j] = (level[i][j] == 0);
}
}
snakeX[0] = ROWS / 2;
snakeY[0] = COLS / 2;
snakeLength = 1;
generateFood();
direction = 0;
score = 0;
}
private void generateFood() {
while (true) {
foodX = (int) (Math.random() * ROWS);
foodY = (int) (Math.random() * COLS);
if (blocks[foodX][foodY]) {
break;
}
}
}
private void startGame() {
Timer timer = new Timer(200 - levelIndex * 50, new ActionListener() {
public void actionPerformed(ActionEvent e) {
move();
repaint();
}
});
timer.start();
}
private void move() {
int dx = (direction == 1) ? -1 : (direction == 3 ? 1 : 0);
int dy = (direction == 0) ? -1 : (direction == 2 ? 1 : 0);
int x = snakeX[0] + dx;
int y = snakeY[0] + dy;
if (x < 0 || x >= ROWS || y < 0 || y >= COLS || !blocks[x][y]) {
JOptionPane.showMessageDialog(null, "Game Over!");
resetGame();
return;
}
for (int i = snakeLength - 1; i > 0; i--) {
snakeX[i] = snakeX[i - 1];
snakeY[i] = snakeY[i - 1];
}
snakeX[0] = x;
snakeY[0] = y;
if (x == foodX && y == foodY) {
snakeLength++;
score++;
generateFood();
}
}
public void paint(Graphics g) {
super.paint(g);
for (int i = 0; i < ROWS; i++) {
for (int j = 0; j < COLS; j++) {
if (blocks[i][j]) {
g.setColor(Color.GRAY);
g.fillRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
} else {
g.setColor(Color.WHITE);
g.fillRect(j * BLOCK_SIZE, i * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
}
}
for (int i = 0; i < snakeLength; i++) {
g.setColor(Color.GREEN);
g.fillRect(snakeY[i] * BLOCK_SIZE, snakeX[i] * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
}
g.setColor(Color.RED);
g.fillOval(foodY * BLOCK_SIZE, foodX * BLOCK_SIZE, BLOCK_SIZE, BLOCK_SIZE);
g.setColor(Color.BLACK);
g.drawString("Score: " + score, 10, ROWS * BLOCK_SIZE + 20);
g.drawString("Level: " + (levelIndex + 1), 100, ROWS * BLOCK_SIZE + 20);
}
public void keyPressed(KeyEvent e) {
int code = e.getKeyCode();
if (code == KeyEvent.VK_UP && direction != 2) {
direction = 0;
} else if (code == KeyEvent.VK_DOWN && direction != 0) {
direction = 2;
} else if (code == KeyEvent.VK_LEFT && direction != 3) {
direction = 1;
} else if (code == KeyEvent.VK_RIGHT && direction != 1) {
direction = 3;
}
}
public void keyReleased(KeyEvent e) {
}
public void keyTyped(KeyEvent e) {
}
}
```
这个代码实现了一个贪吃蛇游戏,包含有三个不同的关卡。在窗口中,有三个按钮,分别对应于不同的关卡。当用户点击某个按钮时,游戏将切换到相应的关卡。在游戏界面中,用户可以通过上下左右键来控制蛇的移动。当蛇吃到食物时,用户的得分将增加。如果蛇碰到墙壁或自己的身体,游戏将结束。
希望这个代码对您有所帮助。
阅读全文
相关推荐
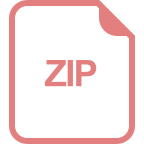

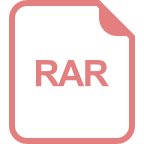
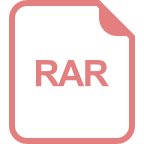
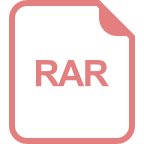
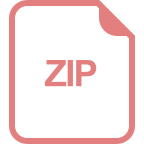
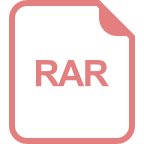
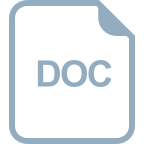
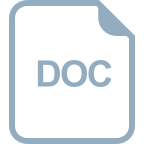


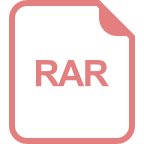
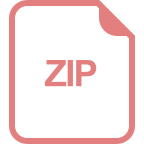
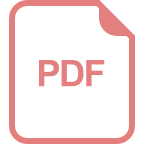