springboot+vue将html格式的数据转为docx文档并将html样式转化为对应的docx样式的代码
时间: 2024-04-08 18:33:01 浏览: 254
可以使用Apache POI库来实现将HTML格式的数据转为docx文档,并使用Apache POI的XWPFStyles来实现将HTML样式转化为对应的docx样式。以下是一个示例代码:
```java
import org.apache.poi.xwpf.usermodel.*;
import org.jsoup.Jsoup;
import org.jsoup.nodes.Document;
import org.jsoup.nodes.Element;
import org.jsoup.select.Elements;
import java.io.FileOutputStream;
import java.io.IOException;
public class HtmlToDocxConverter {
public static void convertHtmlToDocx(String html, String outputPath) throws IOException {
// 解析HTML
Document doc = Jsoup.parse(html);
// 创建文档对象
XWPFDocument document = new XWPFDocument();
// 获取所有段落
Elements paragraphs = doc.body().select("p");
for (Element paragraph : paragraphs) {
// 创建段落对象
XWPFParagraph xwpfParagraph = document.createParagraph();
// 设置段落样式
String style = paragraph.attr("style");
if (!style.isEmpty()) {
XWPFStyles styles = document.createStyles();
XWPFStyle xwpfStyle = styles.getStyle(paragraph.tagName());
if (xwpfStyle == null) {
xwpfStyle = styles.createStyle(paragraph.tagName());
}
xwpfStyle.setType(STStyleType.PARAGRAPH);
xwpfStyle.setQFormat(true);
xwpfParagraph.setStyle(paragraph.tagName());
}
// 设置段落内容
xwpfParagraph.createRun().setText(paragraph.text());
}
// 保存文档
FileOutputStream out = new FileOutputStream(outputPath);
document.write(out);
out.close();
}
public static void main(String[] args) throws IOException {
String html = "<html><body><p style=\"font-size: 14px; color: red;\">Hello, World!</p></body></html>";
String outputPath = "output.docx";
convertHtmlToDocx(html, outputPath);
}
}
```
以上代码使用Jsoup库来解析HTML,然后使用Apache POI库将解析后的内容转为docx文档。在转化过程中,会根据HTML样式设置对应的docx样式。最后,将生成的docx文档保存到指定路径。
请注意,为了使代码简洁,以上示例省略了异常处理部分,实际应用中应根据需要进行异常处理。另外,需要在项目中引入Apache POI和Jsoup的依赖。
阅读全文
相关推荐
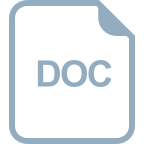
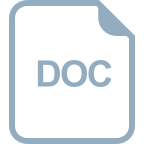
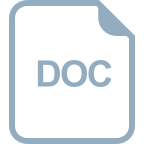
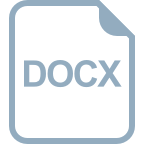
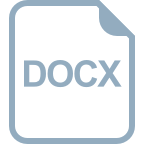
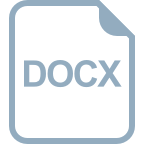
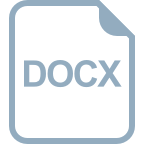
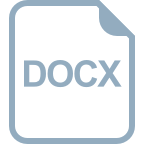
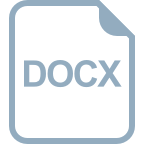