java zip 中文文件名乱码_java使用zip压缩中文文件名乱码的解决办法
时间: 2023-09-12 09:08:19 浏览: 176
在Java中使用Zip压缩中文文件名可能会出现乱码的问题,这是因为Zip文件格式默认使用的是ASCII编码,而中文字符需要使用其他编码方式进行处理。解决这个问题的方法有两种:
1. 使用Apache Commons Compress库
可以使用Apache Commons Compress库来压缩文件,这个库支持多种编码方式,可以解决中文文件名乱码的问题。示例代码如下:
```java
import org.apache.commons.compress.archivers.ArchiveEntry;
import org.apache.commons.compress.archivers.zip.ZipArchiveOutputStream;
import org.apache.commons.compress.utils.IOUtils;
import java.io.*;
public class ZipUtil {
public static void zip(String sourcePath, String destPath) throws IOException {
FileOutputStream fos = new FileOutputStream(destPath);
ZipArchiveOutputStream zos = new ZipArchiveOutputStream(fos);
File file = new File(sourcePath);
String basePath = file.getParent();
compress(file, zos, basePath);
zos.close();
fos.close();
}
private static void compress(File file, ZipArchiveOutputStream zos, String basePath) throws IOException {
if (file.isDirectory()) {
File[] files = file.listFiles();
if (files.length == 0) {
String path = file.getPath().substring(basePath.length() + 1) + "/";
ArchiveEntry entry = zos.createArchiveEntry(new File(file.getPath()), path);
zos.putArchiveEntry(entry);
zos.closeArchiveEntry();
} else {
for (File f : files) {
compress(f, zos, basePath);
}
}
} else {
String path = file.getPath().substring(basePath.length() + 1);
ArchiveEntry entry = zos.createArchiveEntry(new File(file.getPath()), path);
zos.putArchiveEntry(entry);
InputStream is = new FileInputStream(file);
IOUtils.copy(is, zos);
is.close();
zos.closeArchiveEntry();
}
}
}
```
2. 使用Java的ZipOutputStream类
Java中自带的ZipOutputStream类也可以用于压缩文件,但是需要手动设置编码方式,示例代码如下:
```java
import java.io.*;
import java.nio.charset.Charset;
import java.util.zip.ZipEntry;
import java.util.zip.ZipOutputStream;
public class ZipUtil {
private static final int BUFFER_SIZE = 2 * 1024;
public static void zip(String sourcePath, String destPath) throws IOException {
FileOutputStream fos = new FileOutputStream(destPath);
ZipOutputStream zos = new ZipOutputStream(fos, Charset.forName("GBK"));
File file = new File(sourcePath);
String basePath = file.getParent();
compress(file, zos, basePath);
zos.close();
fos.close();
}
private static void compress(File file, ZipOutputStream zos, String basePath) throws IOException {
if (file.isDirectory()) {
File[] files = file.listFiles();
if (files.length == 0) {
String path = file.getPath().substring(basePath.length() + 1) + "/";
ZipEntry entry = new ZipEntry(path);
zos.putNextEntry(entry);
zos.closeEntry();
} else {
for (File f : files) {
compress(f, zos, basePath);
}
}
} else {
String path = file.getPath().substring(basePath.length() + 1);
ZipEntry entry = new ZipEntry(path);
zos.putNextEntry(entry);
InputStream is = new FileInputStream(file);
byte[] buffer = new byte[BUFFER_SIZE];
int len;
while ((len = is.read(buffer)) != -1) {
zos.write(buffer, 0, len);
}
is.close();
zos.closeEntry();
}
}
}
```
以上两种方法都可以解决中文文件名乱码的问题,具体使用哪一种方法可以根据实际需要选择。
阅读全文
相关推荐
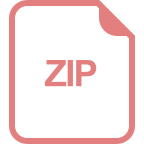

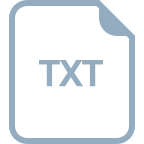
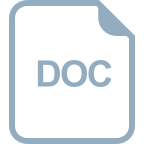

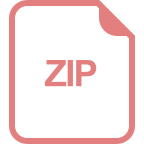
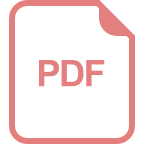


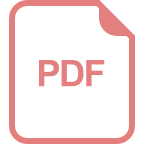
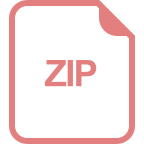
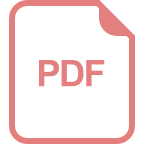



