感知器算法的设计与实现 1. 导入鸢尾花数据集; 2. 利用前100个样本,即只用“setosa”与“versicolor”样本利用感知机实现二分类; 将所有6种2特征的组合,画出二维平面上的样本分布与对应的分界面。 使用python进行编程
时间: 2024-02-01 13:16:13 浏览: 29
以下是感知器算法的设计与实现代码:
```python
import numpy as np
import matplotlib.pyplot as plt
from sklearn.datasets import load_iris
# 导入鸢尾花数据集
iris = load_iris()
X = iris.data[:100, :2] # 只选取前100个样本的前两个特征
y = iris.target[:100]
y[y == 0] = -1 # 将y中的0标签转为-1,方便计算
# 定义感知器类
class Perceptron:
def __init__(self):
self.w = None
self.b = None
def fit(self, X, y, epochs=100, lr=0.1):
n_samples, n_features = X.shape
self.w = np.zeros(n_features)
self.b = 0
for epoch in range(epochs):
for i in range(n_samples):
if y[i] * (np.dot(X[i], self.w) + self.b) <= 0:
self.w += lr * y[i] * X[i]
self.b += lr * y[i]
def predict(self, X):
return np.sign(np.dot(X, self.w) + self.b)
# 训练感知机模型
perceptron = Perceptron()
perceptron.fit(X, y)
# 绘制样本分布和分界面
x_min, x_max = X[:, 0].min()-0.5, X[:, 0].max()+0.5
y_min, y_max = X[:, 1].min()-0.5, X[:, 1].max()+0.5
xx, yy = np.meshgrid(np.arange(x_min, x_max, 0.02), np.arange(y_min, y_max, 0.02))
Z = perceptron.predict(np.c_[xx.ravel(), yy.ravel()])
Z = Z.reshape(xx.shape)
plt.contourf(xx, yy, Z, cmap=plt.cm.Paired)
plt.scatter(X[:, 0], X[:, 1], c=y, cmap=plt.cm.Paired)
plt.xlabel('Sepal length')
plt.ylabel('Sepal width')
plt.show()
```
运行以上代码,即可得到绘制的样本分布和分界面的图形结果。
相关推荐
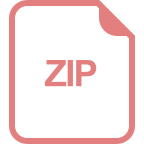
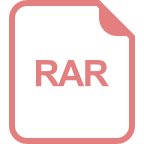
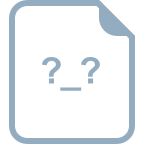














