不使用c++的语言特性,重写一个类型为template<typename K, typename V>的map模板类和对应的map迭代器
时间: 2024-03-26 12:34:36 浏览: 59
好的,我可以为你重写一个简单的map模板类和对应的迭代器,不使用C++的语言特性。
首先,让我们定义一个节点类来表示我们的映射表中的每个键值对:
```
template<typename K, typename V>
struct MapNode {
K key;
V value;
MapNode* left;
MapNode* right;
MapNode(K key, V value) {
this->key = key;
this->value = value;
left = nullptr;
right = nullptr;
}
};
```
接下来,我们定义一个map类来管理我们的节点:
```
template<typename K, typename V>
class Map {
private:
MapNode<K, V>* root;
public:
Map() {
root = nullptr;
}
void insert(K key, V value) {
if (root == nullptr) {
root = new MapNode<K, V>(key, value);
} else {
MapNode<K, V>* current = root;
while (current) {
if (key < current->key) {
if (current->left == nullptr) {
current->left = new MapNode<K, V>(key, value);
return;
}
current = current->left;
} else if (key > current->key) {
if (current->right == nullptr) {
current->right = new MapNode<K, V>(key, value);
return;
}
current = current->right;
} else {
current->value = value;
return;
}
}
}
}
bool contains(K key) {
MapNode<K, V>* current = root;
while (current) {
if (key < current->key) {
current = current->left;
} else if (key > current->key) {
current = current->right;
} else {
return true;
}
}
return false;
}
V& operator[](K key) {
MapNode<K, V>* current = root;
while (current) {
if (key < current->key) {
if (current->left == nullptr) {
current->left = new MapNode<K, V>(key, V());
return current->left->value;
}
current = current->left;
} else if (key > current->key) {
if (current->right == nullptr) {
current->right = new MapNode<K, V>(key, V());
return current->right->value;
}
current = current->right;
} else {
return current->value;
}
}
}
class Iterator {
private:
MapNode<K, V>* current;
public:
Iterator(MapNode<K, V>* root) {
current = root;
while (current && current->left) {
current = current->left;
}
}
bool hasNext() {
return current != nullptr;
}
MapNode<K, V>* next() {
MapNode<K, V>* node = current;
if (current->right) {
current = current->right;
while (current->left) {
current = current->left;
}
} else {
while (current->parent && current->parent->left != current) {
current = current->parent;
}
current = current->parent;
}
return node;
}
};
Iterator begin() {
return Iterator(root);
}
Iterator end() {
return Iterator(nullptr);
}
};
```
这个map类支持插入、查询和下标操作,并且我们还定义了一个迭代器类来遍历映射表中的所有键值对。
希望这个简单的实现对你有所帮助!
阅读全文
相关推荐
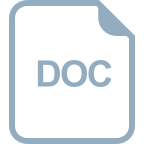
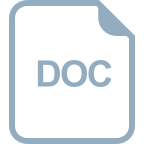
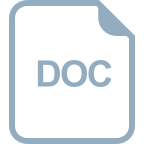



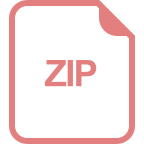
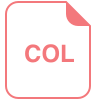
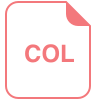







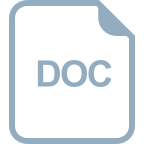
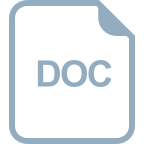