从文件中读取各学生成绩,计算所有学生成绩的平均值,将成绩的最大值、最小值及平均值并存入文本文件中。
时间: 2024-02-13 07:59:41 浏览: 61
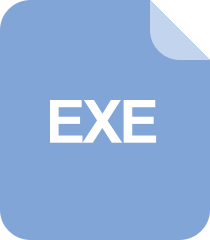
读取考试成绩文件,求最大值、最小值,并排序
好的,这个问题涉及到文件的读写和数据处理,需要一步一步来实现。
首先,我们需要读取学生成绩文件,可以使用 Python 的内置库 `open()` 函数打开文件,并使用 `readlines()` 函数读取每一行的数据:
```python
with open('scores.txt', 'r') as f:
lines = f.readlines()
```
假设学生成绩文件 `scores.txt` 中每一行的格式为 `name score1 score2 score3`,我们可以通过字符串分割将每一行的数据转换为列表:
```python
scores = []
for line in lines:
data = line.strip().split()
name = data[0]
score = list(map(float, data[1:]))
scores.append((name, score))
```
这样我们就得到了一个包含每个学生的姓名和成绩列表的列表 `scores`。
接下来,我们可以计算所有学生成绩的平均值:
```python
total_score = 0
num_students = 0
for name, score in scores:
total_score += sum(score)
num_students += 1
average_score = total_score / num_students
```
然后,我们可以计算成绩的最大值和最小值:
```python
max_score = max([max(s) for _, s in scores])
min_score = min([min(s) for _, s in scores])
```
最后,我们可以将成绩的最大值、最小值及平均值存入文本文件中:
```python
with open('result.txt', 'w') as f:
f.write('Max score: {:.2f}\n'.format(max_score))
f.write('Min score: {:.2f}\n'.format(min_score))
f.write('Average score: {:.2f}\n'.format(average_score))
```
完整代码如下:
```python
with open('scores.txt', 'r') as f:
lines = f.readlines()
scores = []
for line in lines:
data = line.strip().split()
name = data[0]
score = list(map(float, data[1:]))
scores.append((name, score))
total_score = 0
num_students = 0
for name, score in scores:
total_score += sum(score)
num_students += 1
average_score = total_score / num_students
max_score = max([max(s) for _, s in scores])
min_score = min([min(s) for _, s in scores])
with open('result.txt', 'w') as f:
f.write('Max score: {:.2f}\n'.format(max_score))
f.write('Min score: {:.2f}\n'.format(min_score))
f.write('Average score: {:.2f}\n'.format(average_score))
```
阅读全文
相关推荐




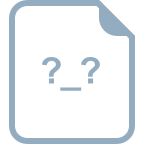
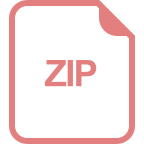
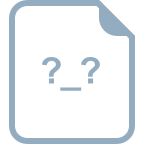
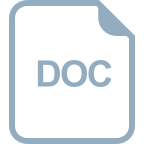
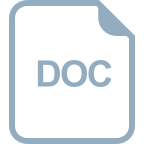






