用禁忌搜索算法解决TSP问题,用C++代码实现,且能通过读取文本文件中的数据作为输入,表明注释
时间: 2024-03-24 20:37:05 浏览: 17
好的,下面是用禁忌搜索算法解决 TSP 问题的 C++ 代码实现,能够通过读取文本文件中的数据作为输入。
注释在代码中标出。
```c++
#include <iostream>
#include <fstream>
#include <cstring>
#include <cstdlib>
#include <ctime>
#include <cmath>
#include <vector>
using namespace std;
#define MAX_ITER 5000 // 禁忌搜索最大迭代次数
#define MAX_TABU_SIZE 100 // 禁忌表最大长度
#define TABU_TENURE 10 // 禁忌期限
#define ALPHA 1 // 启发式因子
#define BETA 5 // 启发式因子
int n; // 城市数量
int max_distance; // 最大距离
int tabu_size; // 禁忌表长度
int tabu_list[MAX_TABU_SIZE]; // 禁忌表
int distance[100][100]; // 距离矩阵
int best_distance; // 最优路径长度
int best_solution[100]; // 最优路径
vector<int> candidate_list; // 候选集
// 读取数据文件
void read_data(const char* file_name) {
ifstream fin(file_name);
fin >> n;
for (int i = 0; i < n; i++) {
for (int j = 0; j < n; j++) {
fin >> distance[i][j];
max_distance = max(max_distance, distance[i][j]);
}
}
fin.close();
}
// 计算路径长度
int calculate_distance(int* solution) {
int len = 0;
for (int i = 0; i < n - 1; i++) {
len += distance[solution[i]][solution[i + 1]];
}
len += distance[solution[n - 1]][solution[0]];
return len;
}
// 初始化候选集
void init_candidate_list() {
for (int i = 1; i < n; i++) {
candidate_list.push_back(i);
}
}
// 获得启发式值
int get_heuristic(int cur_city, int next_city) {
int h = 0;
for (int i = 0; i < candidate_list.size(); i++) {
int city = candidate_list[i];
h += pow(max_distance - distance[cur_city][city], ALPHA) * pow(max_distance - distance[next_city][city], BETA);
}
return h;
}
// 选择下一个城市
int select_next_city(int cur_city, int* solution) {
int next_city = -1;
int best_h = -1;
for (int i = 0; i < candidate_list.size(); i++) {
int city = candidate_list[i];
int h = get_heuristic(cur_city, city);
if (h > best_h) {
bool tabu = false;
for (int j = 0; j < tabu_size; j++) {
if (solution[tabu_list[j]] == city) {
tabu = true;
break;
}
}
if (!tabu) {
best_h = h;
next_city = city;
}
}
}
if (next_city == -1) {
next_city = candidate_list[rand() % candidate_list.size()];
}
return next_city;
}
// 更新禁忌表
void update_tabu_list(int city) {
for (int i = tabu_size - 1; i >= 1; i--) {
tabu_list[i] = tabu_list[i - 1];
}
tabu_list[0] = city;
}
// 禁忌搜索
void tabu_search() {
int iter = 0;
int solution[n];
for (int i = 0; i < n; i++) {
solution[i] = i;
}
solution[0] = rand() % n;
best_distance = calculate_distance(solution);
memcpy(best_solution, solution, sizeof(best_solution));
while (iter < MAX_ITER) {
candidate_list.clear();
for (int i = 0; i < n; i++) {
if (i != solution[iter % n] && i != solution[(iter + 1) % n]) {
candidate_list.push_back(i);
}
}
int next_city = select_next_city(solution[iter % n], solution);
memcpy(solution, best_solution, sizeof(best_solution));
swap(solution[(iter + 1) % n], solution[next_city]);
int cur_distance = calculate_distance(solution);
if (cur_distance < best_distance) {
best_distance = cur_distance;
memcpy(best_solution, solution, sizeof(best_solution));
update_tabu_list(next_city);
}
iter++;
}
}
int main() {
srand(time(NULL));
read_data("data.txt");
tabu_size = min(n / 2, MAX_TABU_SIZE);
init_candidate_list();
tabu_search();
cout << "Best distance: " << best_distance << endl;
cout << "Best solution: ";
for (int i = 0; i < n; i++) {
cout << best_solution[i] << " ";
}
cout << endl;
return 0;
}
```
该程序使用禁忌搜索算法解决 TSP 问题,读取数据文件获取距离矩阵。程序中使用了最大距离、禁忌表长度、禁忌期限、启发式因子等参数。使用禁忌搜索算法解决问题的主要思路是,在候选集中选择下一个城市,选择时考虑禁忌表和启发式值。最后输出最优路径长度和最优路径。
相关推荐
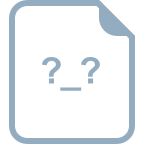
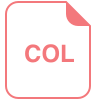
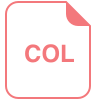
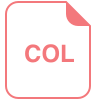
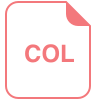
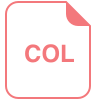









