C语言集合的交并差运算
时间: 2024-09-11 17:15:20 浏览: 74
在C语言中实现集合的交、并、差运算通常需要手动编写相应的函数,因为C语言标准库中并没有直接提供集合操作的函数。集合可以用数组来表示,其中每个元素都是唯一的。为了简化问题,我们假设集合中的元素已经排序且没有重复。
交集(Intersection):是指属于两个集合的共同元素组成的集合。例如,集合A和集合B的交集包含了所有同时属于A和B的元素。
并集(Union):是指将两个集合合并在一起,不包含重复元素的集合。例如,集合A和集合B的并集包含了所有属于A或B的元素,但每个元素只出现一次。
差集(Difference):是指属于一个集合而不属于另一个集合的元素组成的集合。例如,集合A和集合B的差集包含了所有属于A但不属于B的元素。
下面是一个简单的示例代码,展示了如何在C语言中实现集合的交、并、差运算:
```c
#include <stdio.h>
// 假设集合已经按照升序排列
void printSet(int set[], int size) {
for (int i = 0; i < size; i++) {
printf("%d ", set[i]);
}
printf("\n");
}
// 集合的交集
int* intersection(int a[], int aSize, int b[], int bSize, int* resultSize) {
*resultSize = 0;
int *result = (int*)malloc((aSize < bSize ? aSize : bSize) * sizeof(int));
for (int i = 0, j = 0; i < aSize && j < bSize;) {
if (a[i] < b[j]) {
i++;
} else if (a[i] > b[j]) {
j++;
} else {
result[(*resultSize)++] = a[i];
i++;
j++;
}
}
return result;
}
// 集合的并集
int* unionSet(int a[], int aSize, int b[], int bSize, int* resultSize) {
int *result = (int*)malloc((aSize + bSize) * sizeof(int));
int i = 0, j = 0, k = 0;
while (i < aSize && j < bSize) {
if (a[i] < b[j]) {
result[k++] = a[i++];
} else if (a[i] > b[j]) {
result[k++] = b[j++];
} else {
result[k++] = a[i++];
j++;
}
}
while (i < aSize) {
result[k++] = a[i++];
}
while (j < bSize) {
result[k++] = b[j++];
}
*resultSize = k;
return result;
}
// 集合的差集
int* difference(int a[], int aSize, int b[], int bSize, int* resultSize) {
*resultSize = 0;
int *result = (int*)malloc(aSize * sizeof(int));
for (int i = 0, j = 0; i < aSize; i++) {
while (j < bSize && b[j] < a[i]) {
j++;
}
if (j == bSize || b[j] > a[i]) {
result[(*resultSize)++] = a[i];
}
}
return result;
}
int main() {
int setA[] = {1, 2, 3, 4, 5};
int setB[] = {4, 5, 6, 7};
int sizeA = sizeof(setA) / sizeof(setA[0]);
int sizeB = sizeof(setB) / sizeof(setB[0]);
int resultSize;
// 交集
int *setInter = intersection(setA, sizeA, setB, sizeB, &resultSize);
printf("Intersection of A and B: ");
printSet(setInter, resultSize);
free(setInter);
// 并集
int *setUnion = unionSet(setA, sizeA, setB, sizeB, &resultSize);
printf("Union of A and B: ");
printSet(setUnion, resultSize);
free(setUnion);
// 差集
int *setDiffA = difference(setA, sizeA, setB, sizeB, &resultSize);
printf("Difference of A and B: ");
printSet(setDiffA, resultSize);
free(setDiffA);
// B和A的差集
int *setDiffB = difference(setB, sizeB, setA, sizeA, &resultSize);
printf("Difference of B and A: ");
printSet(setDiffB, resultSize);
free(setDiffB);
return 0;
}
```
上述代码仅提供了简单的实现,没有考虑所有边界情况,例如内存分配失败等。在实际应用中可能需要更健壮的错误处理和优化。
阅读全文
相关推荐
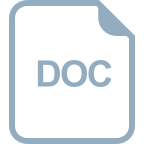
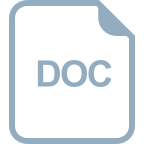
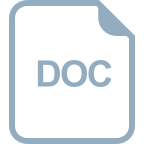
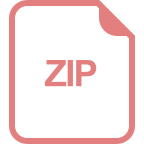
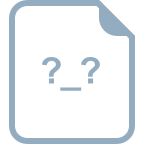
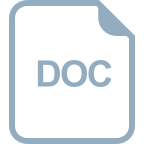
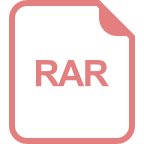
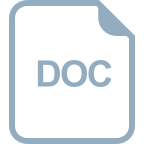
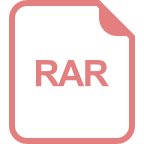
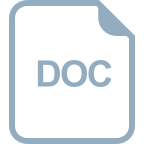
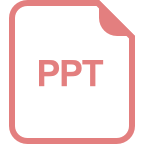
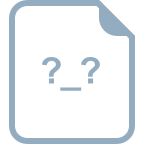
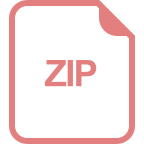
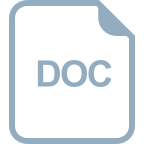