多个机器人协同工作,但又独立工作,当收到其他机器人帮助指令时再去协作的程序用Python写一份
时间: 2023-05-29 16:05:51 浏览: 139
这里给出一个基于Python的简单示例程序,实现多个机器人协同工作,但又独立工作,当收到其他机器人帮助指令时再去协作的功能。具体实现如下:
```python
# 导入相关库
import time
import random
import threading
# 定义机器人类
class Robot:
def __init__(self, name):
self.name = name
self.x = random.randint(0, 100)
self.y = random.randint(0, 100)
self.busy = False
def move(self, x, y):
# 模拟机器人移动
time.sleep(random.uniform(0.5, 1))
self.x = x
self.y = y
def work(self):
# 模拟机器人工作
print(f"{self.name} is working...")
time.sleep(random.uniform(2, 3))
self.busy = False
# 定义协同工作类
class WorkTogether:
def __init__(self, robots):
self.robots = robots
def help(self, robot):
# 随机找一个空闲的机器人来协助
for r in self.robots:
if not r.busy and r != robot:
r.busy = True
print(f"{r.name} is helping {robot.name}...")
t = threading.Thread(target=r.work)
t.start()
return
def run(self):
# 模拟机器人独立工作
for robot in self.robots:
robot.busy = True
print(f"{robot.name} is working...")
t = threading.Thread(target=robot.work)
t.start()
time.sleep(random.uniform(1, 2))
# 有一定概率会请求其他机器人帮助
if random.random() < 0.3:
print(f"{robot.name} needs help!")
self.help(robot)
# 测试程序
if __name__ == "__main__":
robots = [Robot("Robot1"), Robot("Robot2"), Robot("Robot3")]
work_together = WorkTogether(robots)
work_together.run()
```
在上面的示例程序中,我们定义了一个机器人类 `Robot`,其中有一个 `move` 方法用来模拟机器人移动,还有一个 `work` 方法用来模拟机器人工作。另外,我们还定义了一个协同工作类 `WorkTogether`,其中有一个 `help` 方法用来模拟机器人协助其他机器人工作。最后,我们在 `run` 方法中模拟了多个机器人的独立工作,并有一定概率会请求其他机器人帮助。
在程序运行时,我们创建了三个机器人,并将它们放在一个列表中传递给了 `WorkTogether` 类。然后我们调用 `run` 方法来模拟机器人的工作。
在 `run` 方法中,我们先让每个机器人独立工作一段时间,然后有一定概率会请求其他机器人帮助。如果请求帮助,我们就随机找一个空闲的机器人来协助,并启动一个新的线程来执行协助工作。注意,为了避免多个机器人同时协助同一个机器人,我们在协助方法中加入了一个简单的锁机制,确保同一时刻只有一个机器人在协助。
最后,在每个机器人的工作方法中,我们使用 `time.sleep` 函数来模拟机器人的工作时间,同时将 `busy` 标志设置为 False,表示机器人空闲。
阅读全文
相关推荐
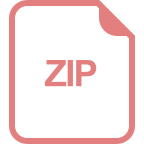

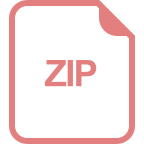
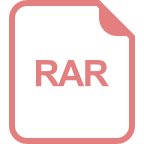
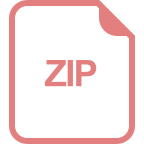
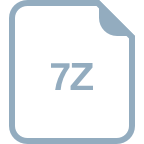
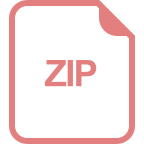
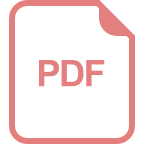
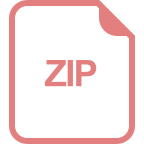
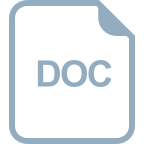
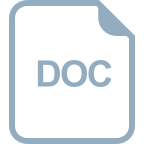
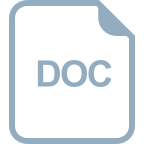
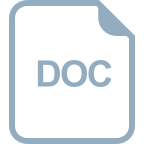
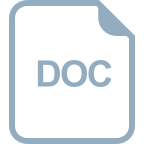
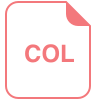
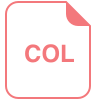
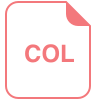