Epoch 20/20 35/35 [==============================] - 2s 50ms/step - loss: 3.3574e-04 - accuracy: 9.1870e-04 - val_loss: 8.7506e-04
时间: 2023-09-09 13:06:18 浏览: 32
这是一个深度学习模型的训练日志,其中Epoch 20/20表示模型已经训练了20个epoch(迭代轮数),35/35 [==============================]表示当前epoch的训练进度,loss: 3.3574e-04表示当前epoch的训练损失值,accuracy: 9.1870e-04表示当前epoch的训练准确率,val_loss: 8.7506e-04表示当前epoch的验证集上的损失值。训练损失值和验证集损失值都越小,表示模型的性能越好。
相关问题
config configs/culane.py
这是一个CULane数据集的配置文件,其中包含了训练、验证和测试的相关参数设置。
```
_base_ = [
'../_base_/models/fcn_r50-d8.py', '../_base_/datasets/culane.py',
'../_base_/default_runtime.py', '../_base_/schedules/schedule_80k.py'
]
# model settings
model = dict(
decode_head=dict(num_classes=5), auxiliary_head=dict(num_classes=5))
# dataset settings
data = dict(
samples_per_gpu=4,
workers_per_gpu=4,
train=dict(type='CULane', split='train'),
val=dict(type='CULane', split='val'),
test=dict(type='CULane', split='test'))
# optimizer
optimizer = dict(type='SGD', lr=0.02, momentum=0.9, weight_decay=0.0001)
optimizer_config = dict(grad_clip=dict(max_norm=35, norm_type=2))
# learning policy
lr_config = dict(policy='poly', power=0.9, by_epoch=False)
# runtime settings
runner = dict(type='EpochBasedRunner', max_epochs=160)
checkpoint_config = dict(by_epoch=False, interval=20)
evaluation = dict(interval=20, metric='mIoU')
```
其中:
- `_base_` 表示使用的基础配置文件,这里使用了模型、数据集、运行时设置和学习率调度等基础配置文件。
- `model` 表示模型相关的设置,这里使用 FCN-R50-d8 作为基础模型,decode_head 和 auxiliary_head 都设置为 5 类别(即 5 条车道线)。
- `data` 表示数据集相关的设置,包括每个 GPU 用来训练的样本数、数据集划分方式等。
- `optimizer` 和 `optimizer_config` 表示优化器相关的设置,这里使用 SGD 优化器,设置了学习率、动量和权重衰减等参数。
- `lr_config` 表示学习率调度的设置,这里使用了 Poly 调度,设置了幂次和是否按 epoch 计算。
- `runner` 表示训练器相关的设置,这里使用了 EpochBasedRunner,并且设置了最大训练轮数。
- `checkpoint_config` 表示保存模型参数的设置,这里设置了每 20 轮保存一次模型,并且不按 epoch 计算。
- `evaluation` 表示验证时的评估设置,这里设置了每 20 轮进行一次验证,并且使用 mIoU 作为评估指标。
import torchimport torch.nn as nnimport torch.optim as optimimport numpy as np# 定义视频特征提取模型class VideoFeatureExtractor(nn.Module): def __init__(self): super(VideoFeatureExtractor, self).__init__() self.conv1 = nn.Conv2d(3, 16, kernel_size=3, stride=1, padding=1) self.conv2 = nn.Conv2d(16, 32, kernel_size=3, stride=1, padding=1) self.pool = nn.MaxPool2d(kernel_size=2, stride=2) def forward(self, x): x = self.pool(torch.relu(self.conv1(x))) x = self.pool(torch.relu(self.conv2(x))) x = x.view(-1, 32 * 8 * 8) return x# 定义推荐模型class VideoRecommendationModel(nn.Module): def __init__(self, num_videos, embedding_dim): super(VideoRecommendationModel, self).__init__() self.video_embedding = nn.Embedding(num_videos, embedding_dim) self.user_embedding = nn.Embedding(num_users, embedding_dim) self.fc1 = nn.Linear(2 * embedding_dim, 64) self.fc2 = nn.Linear(64, 1) def forward(self, user_ids, video_ids): user_embed = self.user_embedding(user_ids) video_embed = self.video_embedding(video_ids) x = torch.cat([user_embed, video_embed], dim=1) x = torch.relu(self.fc1(x)) x = self.fc2(x) return torch.sigmoid(x)# 加载数据data = np.load('video_data.npy')num_users, num_videos, embedding_dim = data.shapetrain_data = torch.tensor(data[:int(0.8 * num_users)])test_data = torch.tensor(data[int(0.8 * num_users):])# 定义模型和优化器feature_extractor = VideoFeatureExtractor()recommendation_model = VideoRecommendationModel(num_videos, embedding_dim)optimizer = optim.Adam(recommendation_model.parameters())# 训练模型for epoch in range(10): for user_ids, video_ids, ratings in train_data: optimizer.zero_grad() video_features = feature_extractor(video_ids) ratings_pred = recommendation_model(user_ids, video_ids) loss = nn.BCELoss()(ratings_pred, ratings) loss.backward() optimizer.step() # 计算测试集准确率 test_ratings_pred = recommendation_model(test_data[:, 0], test_data[:, 1]) test_loss = nn.BCELoss()(test_ratings_pred, test_data[:, 2]) test_accuracy = ((test_ratings_pred > 0.5).float() == test_data[:, 2]).float().mean() print('Epoch %d: Test Loss %.4f, Test Accuracy %.4f' % (epoch, test_loss.item(), test_accuracy.item()))解释每一行代码
1. `import torch`: 导入 PyTorch 模块
2. `import torch.nn as nn`: 导入 PyTorch 中的神经网络模块
3. `import torch.optim as optim`: 导入 PyTorch 中的优化器模块
4. `import numpy as np`: 导入 NumPy 模块,并将其重命名为 np
5. `class VideoFeatureExtractor(nn.Module):`:定义视频特征提取模型,继承自 nn.Module
6. `def __init__(self):`:定义初始化函数,初始化视频特征提取模型中的卷积层和池化层
7. `super(VideoFeatureExtractor, self).__init__()`: 调用父类的初始化函数
8. `self.conv1 = nn.Conv2d(3, 16, kernel_size=3, stride=1, padding=1)`: 定义一个 3 x 3 的卷积层,输入通道数为 3 ,输出通道数为 16,卷积核大小为 3,步长为 1,填充为 1
9. `self.conv2 = nn.Conv2d(16, 32, kernel_size=3, stride=1, padding=1)`: 定义一个 3 x 3 的卷积层,输入通道数为 16 ,输出通道数为 32,卷积核大小为 3,步长为 1,填充为 1
10. `self.pool = nn.MaxPool2d(kernel_size=2, stride=2)`: 定义一个大小为 2x2 的最大池化层
11. `def forward(self, x):`: 定义前向传播函数,将输入 x 经过卷积层和池化层后展平输出
12. `x = self.pool(torch.relu(self.conv1(x)))`: 将输入 x 经过第一层卷积层、ReLU 激活函数和最大池化层
13. `x = self.pool(torch.relu(self.conv2(x)))`: 将输入 x 经过第二层卷积层、ReLU 激活函数和最大池化层
14. `x = x.view(-1, 32 * 8 * 8)`: 将输出结果展平为一维向量,大小为 32*8*8
15. `return x`: 返回输出结果 x
16. `class VideoRecommendationModel(nn.Module):`:定义推荐模型,继承自 nn.Module
17. `def __init__(self, num_videos, embedding_dim):`:定义初始化函数,初始化推荐模型中的用户嵌入层、视频嵌入层和全连接层
18. `super(VideoRecommendationModel, self).__init__()`: 调用父类的初始化函数
19. `self.video_embedding = nn.Embedding(num_videos, embedding_dim)`: 定义视频嵌入层,输入维度为 num_videos,输出维度为 embedding_dim
20. `self.user_embedding = nn.Embedding(num_users, embedding_dim)`: 定义用户嵌入层,输入维度为 num_users,输出维度为 embedding_dim
21. `self.fc1 = nn.Linear(2 * embedding_dim, 64)`: 定义一个全连接层,输入维度为 2*embedding_dim,输出维度为 64
22. `self.fc2 = nn.Linear(64, 1)`: 定义一个全连接层,输入维度为 64,输出维度为 1
23. `def forward(self, user_ids, video_ids):`: 定义前向传播函数,将用户和视频 id 经过嵌入层和全连接层计算得到推荐评分
24. `user_embed = self.user_embedding(user_ids)`: 将用户 id 经过用户嵌入层得到用户嵌入
25. `video_embed = self.video_embedding(video_ids)`: 将视频 id 经过视频嵌入层得到视频嵌入
26. `x = torch.cat([user_embed, video_embed], dim=1)`: 将用户嵌入和视频嵌入拼接起来
27. `x = torch.relu(self.fc1(x))`: 将拼接后的结果经过激活函数和全连接层
28. `x = self.fc2(x)`: 将全连接层的输出作为推荐评分
29. `return torch.sigmoid(x)`: 将推荐评分经过 sigmoid 函数转换到 [0,1] 区间内
30. `data = np.load('video_data.npy')`: 从文件中读取数据
31. `num_users, num_videos, embedding_dim = data.shape`: 获取数据的形状,即用户数、视频数和嵌入维度
32. `train_data = torch.tensor(data[:int(0.8 * num_users)])`: 将前 80% 的数据作为训练集,并转换为 PyTorch 的 tensor 格式
33. `test_data = torch.tensor(data[int(0.8 * num_users):])`: 将后 20% 的数据作为测试集,并转换为 PyTorch 的 tensor 格式
34. `feature_extractor = VideoFeatureExtractor()`: 创建视频特征提取模型的实例
35. `recommendation_model = VideoRecommendationModel(num_videos, embedding_dim)`: 创建推荐模型的实例
36. `optimizer = optim.Adam(recommendation_model.parameters())`: 创建优化器,使用 Adam 算法优化推荐模型的参数
37. `for epoch in range(10):`: 开始训练,进行 10 轮迭代
38. `for user_ids, video_ids, ratings in train_data:`: 对训练集中的每个样本进行训练
39. `optimizer.zero_grad()`: 将梯度清零
40. `video_features = feature_extractor(video_ids)`: 提取视频特征
41. `ratings_pred = recommendation_model(user_ids, video_ids)`: 通过推荐模型得到预测评分
42. `loss = nn.BCELoss()(ratings_pred, ratings)`: 计算二分类交叉熵损失
43. `loss.backward()`: 反向传播求梯度
44. `optimizer.step()`: 更新模型参数
45. `test_ratings_pred = recommendation_model(test_data[:, 0], test_data[:, 1])`: 对测试集进行评分预测
46. `test_loss = nn.BCELoss()(test_ratings_pred, test_data[:, 2])`: 计算测试集上的损失
47. `test_accuracy = ((test_ratings_pred > 0.5).float() == test_data[:, 2]).float().mean()`: 计算测试集上的准确率
48. `print('Epoch %d: Test Loss %.4f, Test Accuracy %.4f' % (epoch, test_loss.item(), test_accuracy.item()))`: 输出每轮迭代的测试集损失和准确率
相关推荐
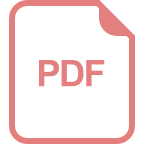
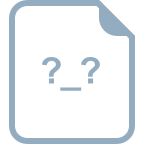
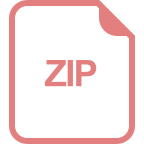












