C++对vector对象数组进行排序的同时自定义排序规则
时间: 2023-12-23 16:04:08 浏览: 80
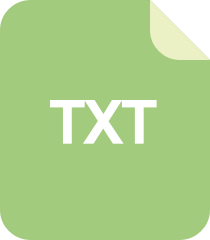
c++语言实现排序
可以使用STL库中的sort函数对vector对象数组进行排序并自定义排序规则。示例代码如下:
```cpp
#include <iostream>
#include <vector>
#include <algorithm>
using namespace std;
bool myCompare(int a, int b) {
return a > b; // 降序排序
}
int main()
{
vector<int> vec {5, 1, 3, 2, 4};
// 使用sort函数进行自定义排序
sort(vec.begin(), vec.end(), myCompare);
// 输出排序后的结果
for (auto num : vec) {
cout << num << " ";
}
return 0;
}
```
输出结果为:5 4 3 2 1。
在代码中,我们定义了一个自定义函数myCompare,该函数的返回值类型为bool型,接受两个int型参数a和b,用于自定义排序规则。在主函数中,我们首先定义了一个vector对象数组vec,其中包含了5个整型元素。然后我们使用sort函数对vec进行排序,并传入自定义函数myCompare作为第三个参数,即用于自定义排序规则。最后使用for循环输出排序后的结果。
本例中自定义函数myCompare为降序排序,如果需要进行升序排序,则返回值应为a < b。
阅读全文
相关推荐















