一个vector数组根据某个数把数组的数排序C++
时间: 2024-12-23 19:14:31 浏览: 2
在C++中,你可以使用`std::sort`函数配合自定义比较函数来自定义排序规则,包括按特定数值对`std::vector`进行排序。这里假设你想按照一个整数元素对整个向量进行升序排列,可以这样做:
```cpp
#include <iostream>
#include <vector>
#include <algorithm> // 包含sort函数
// 自定义比较函数模板,用于根据给定值sort
template <typename T>
bool compareByValue(const std::pair<int, T>& a, const std::pair<int, T>& b, int value) {
return a.first < b.first || (a.first == b.first && a.second < value); // 按照第一个元素升序,如果相等再按第二个元素是否小于目标值
}
int main() {
std::vector<std::pair<int, int>> vec = { {4, 5}, {2, 10}, {6, 1} }; // 示例向量,包含元组(int, int)
int sortValue = 7; // 要排序的特定数值
// 使用自定义比较函数和sort对向量进行排序
std::sort(vec.begin(), vec.end(), [sortValue](const std::pair<int, int>& a, const std::pair<int, int>& b) {
return compareByValue(a, b, sortValue);
});
// 输出排序后的向量
for (const auto& pair : vec) {
std::cout << "Value: " << pair.second << ", Index: " << pair.first << " with target " << sortValue << std::endl;
}
return 0;
}
```
在这个例子中,我们首先定义了一个比较函数`compareByValue`,然后在`main`函数中使用这个函数作为`std::sort`的第三个参数,根据`sortValue`来决定元素的顺序。
阅读全文
相关推荐
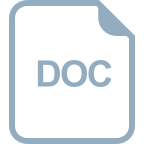
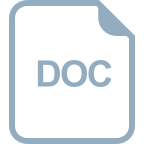
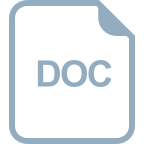








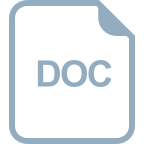





