c++对vector结构体数组进行排序
时间: 2023-10-26 12:05:44 浏览: 290
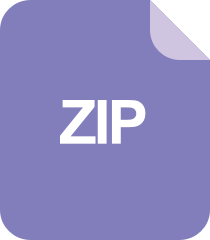
一个结构体的构希望大家看看
可以使用`std::sort()`函数对`vector`结构体数组进行排序。以下是一个示例代码:
```c++
#include <iostream>
#include <vector>
#include <algorithm>
struct MyStruct {
int value;
// 其他成员变量...
};
bool compare(const MyStruct& a, const MyStruct& b) {
return a.value < b.value;
}
int main() {
std::vector<MyStruct> myVector;
// 将需要排序的结构体对象添加到vector中
std::sort(myVector.begin(), myVector.end(), compare);
for (const auto& item : myVector) {
// 输出排序后的结果
std::cout << item.value << " ";
}
return 0;
}
```
阅读全文
相关推荐
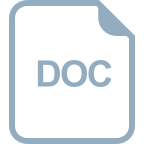
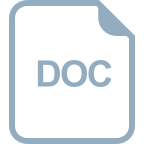















