如何用c++对两个数组进行从大到小的排序,且原数组不变
时间: 2024-09-18 19:02:03 浏览: 73
在C++中,如果你想对两个数组进行降序排列而不改变原始数组,可以使用`std::sort`函数结合自定义比较函数。这里提供一种常见的方法,即使用迭代器和`greater<int>`这个标准库对象:
```cpp
#include <algorithm> // 包含sort和greater头文件
#include <vector>
// 自定义比较函数,用于降序排列
bool compare(const int& a, const int& b) {
return a > b;
}
void sortDescending(std::vector<int>& arr1, std::vector<int>& arr2) {
// 使用sort(arr1.begin(), arr1.end(), greater<int>()) 对arr1进行降序排列
std::sort(arr1.begin(), arr1.end(), compare);
// 对应地,arr2也按照同样的规则排列
std::sort(arr2.begin(), arr2.end(), compare);
}
// 示例用法
int main() {
std::vector<int> array1 = {5, 2, 9, 1};
std::vector<int> array2 = {4, 7, 3, 8};
sortDescending(array1, array2);
// 输出结果(注意:原数组array1和array2已经按降序排列)
for (const auto& num : array1) {
std::cout << num << " ";
}
for (const auto& num : array2) {
std::cout << num << " ";
}
阅读全文
相关推荐
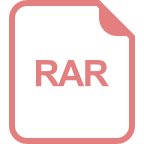
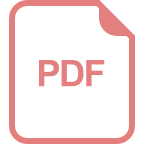
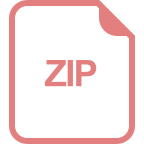




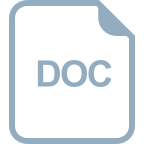
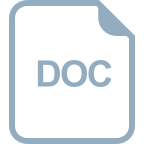
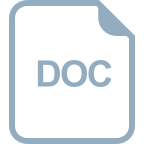
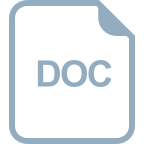
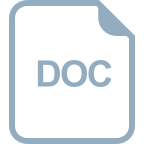
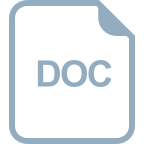
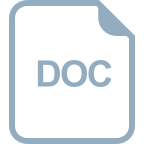
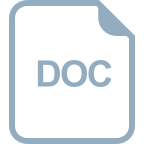
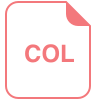
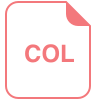

