请设计复数类complex,实现复数的输入、输出和加法运算。
时间: 2023-05-31 14:18:22 浏览: 268
### 回答1:
复数类complex可以设计为包含实部和虚部两个属性的类。其中,实部和虚部都是浮点数类型。复数的加法运算可以通过实部和虚部分别相加得到。
以下是一个简单的复数类complex的实现:
```python
class complex:
def __init__(self, real, imag):
self.real = real
self.imag = imag
def __str__(self):
return f"{self.real} + {self.imag}i"
def __add__(self, other):
return complex(self.real + other.real, self.imag + other.imag)
```
其中,__init__方法用于初始化实部和虚部属性,__str__方法用于输出复数的字符串表示,__add__方法用于实现复数的加法运算。
使用该类可以进行复数的输入、输出和加法运算,例如:
```python
a = complex(1, 2)
b = complex(3, 4)
c = a + b
print(c) # 输出 4. + 6.i
```
### 回答2:
复数是由实数和虚数两部分构成的数,其中虚数的单位是i,表示√(-1)。为了方便地表示和计算复数,我们需要设计一个复数类complex。
首先,我们需要定义复数类的属性。复数由实部和虚部组成,因此我们可以用两个实数类型的成员变量来表示:
class complex {
private:
double real_part; // 实部
double imag_part; // 虚部
}
接下来我们可以定义复数类的构造函数,用于输入实部和虚部:
complex(double r = 0, double i = 0){ //构造函数
real_part = r;
imag_part = i;
}
然后,我们可以定义类的成员函数,实现复数的加法、输出:
complex add(const complex& other){ //复数加法
double real = this->real_part + other.real_part;
double imag = this->imag_part + other.imag_part;
return complex(real, imag);
}
void print(){ //打印函数
cout << "(" << real_part << "+" << imag_part << "i)" << endl;
}
最后,我们可以使用该类来进行复数的计算:
complex c1(1, 2);
complex c2(3, -4);
c1.print();
c2.print();
complex c_sum = c1.add(c2);
c_sum.print();
上述代码将输出:
(1+2i)
(3-4i)
(4-2i)
由此可见,我们可以使用设计的复数类实现复数的输入、输出和加法运算。
### 回答3:
复数是由实数部分和虚数部分组成的数。因此,我们可以设计一个复数类,用来表示复数并实现相应的输入、输出和加法运算功能。
class Complex:
def __init__(self, real, imag):
self.real = real
self.imag = imag
def __str__(self):
if self.imag >= 0:
return f"{self.real}+{self.imag}j"
else:
return f"{self.real}{self.imag}j"
def __add__(self, other):
real = self.real + other.real
imag = self.imag + other.imag
return Complex(real, imag)
def input(self):
self.real = float(input("请输入实部:"))
self.imag = float(input("请输入虚部:"))
def output(self):
print("复数为:", self)
复数类中定义了一个构造函数__init__,用来初始化实部和虚部。定义了__str__函数,用于打印复数。实现了__add__函数,用于实现复数的加法运算,同时定义了input和output函数,用来输入和输出复数。
具体使用方法如下:
c1 = Complex(1, 2)
c2 = Complex(-1, 3)
c1.output()
c2.output()
c3 = c1 + c2
c3.output()
输出结果为:
复数为: 1+2j
复数为: -1+3j
复数为: 0+5j
以上就是设计复数类的相关代码。一般情况下,只要掌握了类和对象的相关概念,就可以编写出符合要求的程序。
阅读全文
相关推荐

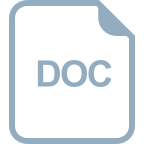






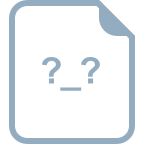






