如何设置Text文本内容?:self.text_box = tk.Text(root, width=30, height=10, font=('宋体', 20))
时间: 2023-06-18 12:06:01 浏览: 46
你可以使用 `insert` 方法向 `Text` 组件中添加文本内容,如下所示:
```python
self.text_box.insert(tk.END, "这是要添加的文本内容")
```
其中,`tk.END` 表示文本框中的末尾位置。如果你想要设置文本框中的内容,可以使用 `delete` 方法清空原有的内容,再使用 `insert` 方法添加新的内容,如下所示:
```python
self.text_box.delete('1.0', tk.END) # 清空文本框中的内容
self.text_box.insert(tk.END, "这是要设置的新内容")
```
其中,`'1.0'` 表示文本框中的第一行第一列位置。
相关问题
from tkinter import * import time class ChatInterface(Frame): def __init__(self, master=None): Frame.__init__(self, master) self.master = master self.initialise() def initialise(self): self.grid() self.entry = Entry(self) self.entry.bind("<Return>", self.send) self.entry.grid(row=0, column=0, sticky=NSEW) self.submit_button = Button(self, text="Send", command=self.send) self.submit_button.grid(row=0, column=1) self.message_box = Text(self, state=DISABLED) self.message_box.grid(row=1, column=0, columnspan=2, sticky=NSEW) class ChatBot: def __init__(self): self.chat_history = [] self.current_question = "" self.response = "" def get_response(self, user_input): # Your code for generating a response goes here return response def send(self, event=None): message = self.entry.get() chat_bot.chat_history.append("You: " + message) self.message_box.configure(state=NORMAL) self.message_box.insert(END, "You: " + message + "\n") self.entry.delete(0, END) self.message_box.configure(state=DISABLED) time.sleep(0.5) self.message_box.configure(state=NORMAL) chat_bot_response = chat_bot.get_response(message) self.message_box.insert(END, "ChatBot: " + chat_bot_response + "\n") chat_bot.chat_history.append("ChatBot: " + chat_bot_response) self.message_box.configure(state=DISABLED) root = Tk() root.title("ChatBot") chat_bot = ChatBot() ChatInterface(root) root.mainloop()这个代码是否有bug
是的,这个代码有一些bug:
1. ChatBot类没有被实例化,需要在ChatInterface类中实例化ChatBot类。
2. get_response方法中的response变量没有被定义,应该将其替换为self.response。
3. send方法中的time.sleep(0.5)会阻塞GUI线程,应该避免使用。
4. ChatInterface类的initialise方法中没有设置self.message_box的高度和宽度,需要指定。
下面是修改后的代码:
```python
from tkinter import *
import time
class ChatInterface(Frame):
def __init__(self, master=None):
Frame.__init__(self, master)
self.master = master
self.chat_bot = ChatBot()
self.initialise()
def initialise(self):
self.grid()
self.entry = Entry(self)
self.entry.bind("<Return>", self.send)
self.entry.grid(row=0, column=0, sticky=NSEW)
self.submit_button = Button(self, text="Send", command=self.send)
self.submit_button.grid(row=0, column=1)
self.message_box = Text(self, state=DISABLED, height=20, width=50)
self.message_box.grid(row=1, column=0, columnspan=2, sticky=NSEW)
def send(self, event=None):
message = self.entry.get()
self.chat_bot.chat_history.append("You: " + message)
self.message_box.configure(state=NORMAL)
self.message_box.insert(END, "You: " + message + "\n")
self.entry.delete(0, END)
self.message_box.configure(state=DISABLED)
chat_bot_response = self.chat_bot.get_response(message)
self.message_box.configure(state=NORMAL)
self.message_box.insert(END, "ChatBot: " + chat_bot_response + "\n")
self.chat_bot.chat_history.append("ChatBot: " + chat_bot_response)
self.message_box.configure(state=DISABLED)
class ChatBot:
def __init__(self):
self.chat_history = []
self.current_question = ""
self.response = ""
def get_response(self, user_input):
# Your code for generating a response goes here
return self.response
root = Tk()
root.title("ChatBot")
ChatInterface(root)
root.mainloop()
```
from tkinter import * import time class ChatInterface(Frame): def __init__(self, master=None): Frame.__init__(self, master) self.master = master self.chat_bot = ChatBot() self.initialise() def initialise(self): self.grid() self.entry = Entry(self) self.entry.bind("<Return>", self.send) self.entry.grid(row=0, column=0, sticky=NSEW) self.submit_button = Button(self, text="Send", command=self.send) self.submit_button.grid(row=0, column=1) self.message_box = Text(self, state=DISABLED, height=20, width=50) self.message_box.grid(row=1, column=0, columnspan=2, sticky=NSEW) def send(self, event=None): message = self.entry.get() self.chat_bot.chat_history.append("You: " + message) self.message_box.configure(state=NORMAL) self.message_box.insert(END, "You: " + message + "\n") self.entry.delete(0, END) self.message_box.configure(state=DISABLED) chat_bot_response = self.chat_bot.get_response(message) self.message_box.configure(state=NORMAL) self.message_box.insert(END, "ChatBot: " + chat_bot_response + "\n") self.chat_bot.chat_history.append("ChatBot: " + chat_bot_response) self.message_box.configure(state=DISABLED) class ChatBot: def __init__(self): self.chat_history = [] self.current_question = "" self.response = "" def get_response(self, user_input): # Your code for generating a response goes here return self.response root = Tk() root.title("ChatBot") ChatInterface(root) root.mainloop()在这个代码上加上注释
# 导入 tkinter 模块
from tkinter import *
# 导入 time 模块 (未被使用)
import time
# 创建一个 ChatInterface 类,继承自 Frame 类
class ChatInterface(Frame):
# 初始化方法
def __init__(self, master=None):
# 调用父类的初始化方法
Frame.__init__(self, master)
# 设置主窗口
self.master = master
# 创建一个 ChatBot 类的实例
self.chat_bot = ChatBot()
# 调用初始化界面的方法
self.initialise()
# 初始化界面的方法
def initialise(self):
# 将当前 ChatInterface 实例网格化
self.grid()
# 创建一个输入框
self.entry = Entry(self)
# 绑定输入框的回车事件为 send 方法
self.entry.bind("<Return>", self.send)
# 将输入框放在界面中的第一行第一列,并且填满整个列
self.entry.grid(row=0, column=0, sticky=NSEW)
# 创建一个发送按钮
self.submit_button = Button(self, text="Send", command=self.send)
# 将发送按钮放在界面中的第一行第二列
self.submit_button.grid(row=0, column=1)
# 创建一个文本框
self.message_box = Text(self, state=DISABLED, height=20, width=50)
# 将文本框放在界面中的第二行第一列和第二列,并且填满整个行
self.message_box.grid(row=1, column=0, columnspan=2, sticky=NSEW)
# 发送消息的方法
def send(self, event=None):
# 获取用户输入的消息
message = self.entry.get()
# 将用户输入的消息添加到 ChatBot 实例的 chat_history 属性中
self.chat_bot.chat_history.append("You: " + message)
# 将用户输入的消息添加到文本框中
self.message_box.configure(state=NORMAL)
self.message_box.insert(END, "You: " + message + "\n")
self.entry.delete(0, END)
self.message_box.configure(state=DISABLED)
# 获取 ChatBot 对用户输入的消息的回复
chat_bot_response = self.chat_bot.get_response(message)
# 将 ChatBot 对用户输入的消息的回复添加到文本框中
self.message_box.configure(state=NORMAL)
self.message_box.insert(END, "ChatBot: " + chat_bot_response + "\n")
# 将 ChatBot 对用户输入的消息的回复添加到 ChatBot 实例的 chat_history 属性中
self.chat_bot.chat_history.append("ChatBot: " + chat_bot_response)
self.message_box.configure(state=DISABLED)
# 创建一个 ChatBot 类
class ChatBot:
# 初始化方法
def __init__(self):
# 创建一个 chat_history 属性,用于存储聊天记录
self.chat_history = []
# 创建一个 current_question 属性,用于存储当前正在回答的问题
self.current_question = ""
# 创建一个 response 属性,用于存储 ChatBot 的回复
self.response = ""
# 获取 ChatBot 对用户输入的消息的回复的方法
def get_response(self, user_input):
# 在这里编写生成回复的代码
return self.response
# 创建一个 tkinter 的根窗口
root = Tk()
# 设置根窗口的标题为 "ChatBot"
root.title("ChatBot")
# 创建一个 ChatInterface 类的实例
ChatInterface(root)
# 进入 tkinter 的事件循环(等待用户操作)
root.mainloop()
相关推荐
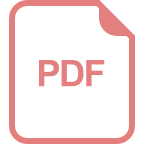
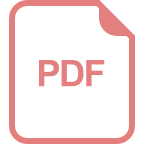
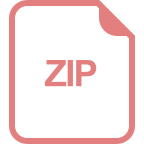












