使用springboot+mybatis+thymeleaf编写代码实现分页查询
时间: 2023-07-31 11:14:08 浏览: 175
首先,需要在pom.xml中引入相应的依赖,这里以MySQL数据库为例:
```xml
<!-- SpringBoot Web模块 -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- SpringBoot Mybatis模块 -->
<dependency>
<groupId>org.mybatis.spring.boot</groupId>
<artifactId>mybatis-spring-boot-starter</artifactId>
<version>2.2.0</version>
</dependency>
<!-- MySQL驱动 -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>8.0.23</version>
</dependency>
```
其次,需要配置Mybatis和数据库连接,可以在application.yml中配置:
```yml
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
url: jdbc:mysql://localhost:3306/testdb?serverTimezone=UTC&useSSL=false&characterEncoding=utf8
username: root
password: root
mybatis:
config-location: classpath:mybatis-config.xml
mapper-locations: classpath:mapper/*.xml
```
其中,`datasource`为数据源的基本信息,`mybatis`则是Mybatis的配置信息,包括配置文件的位置和Mapper文件的位置。
接下来,需要编写Mapper接口和对应的Mapper XML文件。以User表为例:
```java
public interface UserMapper {
List<User> findUserByPage(@Param("start") Integer start, @Param("pageSize") Integer pageSize);
}
```
```xml
<mapper namespace="com.example.demo.mapper.UserMapper">
<select id="findUserByPage" resultType="com.example.demo.entity.User">
select * from user limit #{start},#{pageSize}
</select>
</mapper>
```
其中,`findUserByPage`方法为分页查询方法,`start`为起始位置,`pageSize`为每页数量。
最后,编写Controller层和前端页面。以UserController为例:
```java
@Controller
public class UserController {
@Autowired
private UserMapper userMapper;
@GetMapping("/user")
public String findUserByPage(@RequestParam(value = "pageNum", defaultValue = "1") Integer pageNum,
@RequestParam(value = "pageSize", defaultValue = "5") Integer pageSize,
Model model) {
Integer start = (pageNum - 1) * pageSize;
List<User> userList = userMapper.findUserByPage(start, pageSize);
PageInfo pageInfo = new PageInfo(userList);
model.addAttribute("pageInfo", pageInfo);
return "user";
}
}
```
其中,`findUserByPage`方法接收两个参数:`pageNum`和`pageSize`,表示当前页和每页数量。通过计算获得起始位置,调用Mapper接口进行分页查询,并通过`PageInfo`类将查询结果传递给前端页面。
在前端页面中通过`th:each`循环遍历查询结果,并通过`th:href`生成分页链接。以user.html为例:
```html
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>User List</title>
</head>
<body>
<table border="1">
<thead>
<tr>
<th>ID</th>
<th>Name</th>
<th>Age</th>
<th>Gender</th>
</tr>
</thead>
<tbody>
<tr th:each="user : ${pageInfo.list}">
<td th:text="${user.id}"></td>
<td th:text="${user.name}"></td>
<td th:text="${user.age}"></td>
<td th:text="${user.gender}"></td>
</tr>
</tbody>
</table>
<div>
<a th:href="@{/user?pageNum=1}">首页</a>
<a th:href="@{/user?pageNum=${pageInfo.prePage}}">上一页</a>
<a th:href="@{/user?pageNum=${pageInfo.nextPage}}">下一页</a>
<a th:href="@{/user?pageNum=${pageInfo.pages}}">尾页</a>
</div>
</body>
</html>
```
其中,`pageInfo.list`为查询结果列表,通过`th:each`循环遍历生成表格数据。底部的分页链接则通过`th:href`生成相应的链接。
到这里,一个简单的分页查询就完成了。需要注意的是,以上代码仅为示例,具体实现方式可能会有所不同,需要按照实际需求进行调整。
阅读全文
相关推荐
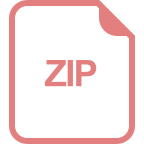
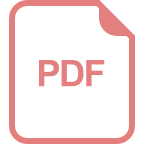
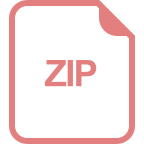
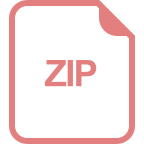
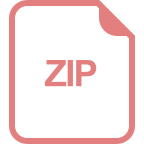
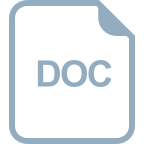
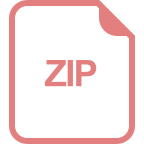
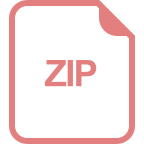
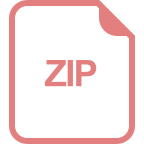
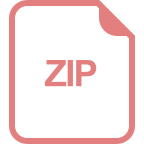
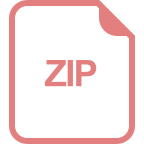
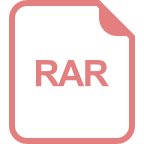
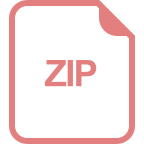
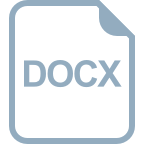
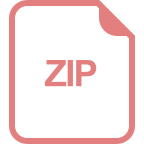
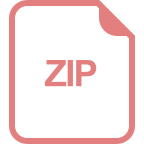
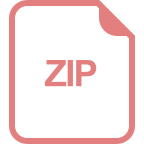
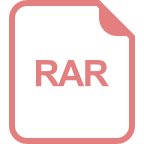