编写一个java类,实现邮件发送功能
时间: 2023-09-22 16:06:15 浏览: 64
### 回答1:
答:可以使用JavaMail API来实现邮件发送功能,编写的类可以使用以下代码:import javax.mail.*;
import javax.mail.internet.*;public class MailSender {
public void sendMail(String to, String subject, String content) {
try {
// 用于建立邮件会话
Properties props = new Properties();
// 发送邮件协议
props.setProperty("mail.transport.protocol", "smtp");
// SMTP服务器
props.setProperty("mail.smtp.host", "smtp.example.com");
// 设置端口
props.setProperty("mail.smtp.port", "25");
// 是否需要认证
props.setProperty("mail.smtp.auth", "true"); // 构建授权信息,用于进行SMTP进行身份验证
Authenticator auth = new Authenticator() {
public PasswordAuthentication getPasswordAuthentication() {
// 用户名和密码
return new PasswordAuthentication("username", "password");
}
}; // 使用环境属性和授权信息,创建邮件会话
Session mailSession = Session.getInstance(props, auth); // 创建邮件消息
MimeMessage message = new MimeMessage(mailSession);
// 发件人
message.setFrom(new InternetAddress("from@example.com"));
// 收件人
message.setRecipient(Message.RecipientType.TO, new InternetAddress(to));
// 邮件主题
message.setSubject(subject);
// 邮件内容
message.setContent(content, "text/html;charset=utf-8"); // 发送邮件
Transport.send(message);
} catch (MessagingException e) {
e.printStackTrace();
}
}
}
### 回答2:
编写一个Java类来实现邮件发送功能可以使用JavaMail API。以下是一个简单的示例:
```java
import javax.mail.*;
import javax.mail.internet.InternetAddress;
import javax.mail.internet.MimeMessage;
import java.util.Properties;
public class EmailSender {
public void sendEmail(String toEmail, String subject, String content) {
// 配置邮件服务器的属性
Properties props = new Properties();
props.put("mail.smtp.host", "smtp.example.com"); // 邮件服务器主机地址
props.put("mail.smtp.port", "587"); // 邮件服务器端口
props.put("mail.smtp.auth", "true"); // 是否需要身份认证
props.put("mail.smtp.starttls.enable", "true"); // 使用 STARTTLS 加密传输
// 创建会话
Session session = Session.getInstance(props, new Authenticator() {
@Override
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication("your_username", "your_password");
}
});
try {
// 创建邮件对象
Message message = new MimeMessage(session);
message.setFrom(new InternetAddress("your_email@example.com")); // 发件人邮箱
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(toEmail)); // 收件人邮箱
message.setSubject(subject); // 邮件主题
message.setText(content); // 邮件内容
// 发送邮件
Transport.send(message);
System.out.println("邮件发送成功");
} catch (MessagingException e) {
System.out.println("邮件发送失败");
e.printStackTrace();
}
}
public static void main(String[] args) {
EmailSender sender = new EmailSender();
sender.sendEmail("recipient@example.com", "测试邮件", "这是一封来自Java的测试邮件");
}
}
```
在使用上述代码时需要将 `smtp.example.com` 替换为您所使用的邮件服务器主机地址,`your_username` 和 `your_password` 更换为您的邮件账号和密码,以及将 `your_email@example.com` 替换为您的发件人邮箱地址。
这个示例中的 `sendEmail` 方法会使用给定的收件人邮箱、主题和内容发送一封邮件。您可以在 `main` 方法中调用这个方法并传入相应的参数,以测试邮件发送功能。
### 回答3:
实现邮件发送功能的Java类可以使用Java Mail库,以下是一个简单的示例代码:
```java
import java.util.Properties;
import javax.mail.*;
import javax.mail.internet.*;
public class EmailSender {
private String senderEmail;
private String senderPassword;
private String smtpHost;
private String smtpPort;
public EmailSender(String senderEmail, String senderPassword, String smtpHost, String smtpPort) {
this.senderEmail = senderEmail;
this.senderPassword = senderPassword;
this.smtpHost = smtpHost;
this.smtpPort = smtpPort;
}
public void sendEmail(String recipientEmail, String subject, String content) throws MessagingException {
// 设置邮件发送配置
Properties properties = new Properties();
properties.put("mail.smtp.host", smtpHost);
properties.put("mail.smtp.port", smtpPort);
properties.put("mail.smtp.auth", "true");
properties.put("mail.smtp.starttls.enable", "true");
properties.put("mail.smtp.ssl.trust", smtpHost);
// 创建邮件会话
Session session = Session.getInstance(properties, new javax.mail.Authenticator() {
protected PasswordAuthentication getPasswordAuthentication() {
return new PasswordAuthentication(senderEmail, senderPassword);
}
});
// 创建邮件消息
MimeMessage message = new MimeMessage(session);
message.setFrom(new InternetAddress(senderEmail));
message.setRecipients(Message.RecipientType.TO, InternetAddress.parse(recipientEmail));
message.setSubject(subject);
message.setText(content);
// 发送邮件
Transport.send(message);
}
public static void main(String[] args) {
String senderEmail = "your_email@example.com";
String senderPassword = "your_email_password";
String smtpHost = "smtp.example.com";
String smtpPort = "587";
String recipientEmail = "recipient@example.com";
String subject = "Hello";
String content = "Hello, World!";
EmailSender emailSender = new EmailSender(senderEmail, senderPassword, smtpHost, smtpPort);
try {
emailSender.sendEmail(recipientEmail, subject, content);
System.out.println("邮件发送成功!");
} catch (MessagingException e) {
System.out.println("邮件发送失败:" + e.getMessage());
}
}
}
```
在上述代码中,`EmailSender`类使用用户名和密码验证身份,并通过SMTP协议将邮件发送到指定的SMTP服务器。你需要将`senderEmail`、`senderPassword`、`smtpHost`和`smtpPort`替换为你自己的邮箱账户和服务器信息。然后,你可以通过调用`sendEmail`方法发送邮件,传入收件人邮箱、主题和内容作为参数。
相关推荐
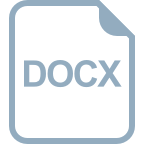














