Java中的method里有一个循环,循环里有个return,请问这个代码有错误吗
时间: 2024-02-19 07:00:15 浏览: 26
这个问题的答案取决于具体的代码实现。在Java中,方法中可以包含循环和return语句,这是完全合法的语法。在循环中使用return语句可以提前结束方法的执行并返回一个值。如果循环中的return语句满足方法的返回值类型,那么这个代码是没有错误的。但是,如果循环中的return语句不满足方法的返回值类型,或者循环中的某些逻辑错误导致返回的值不符合预期,那么这个代码就是有错误的。因此,在编写代码时,需要仔细检查循环中的return语句是否符合预期,并确保代码逻辑正确。
相关问题
Java的一个返回整数类型的method中有一个for循环语句,其中又有一个if语句,if语句中return一个整数,该代码有什么错误吗
这个代码没有语法上的错误,但是需要根据具体的业务逻辑来判断是否符合预期。在Java中,方法中可以包含循环和if语句,并且可以在if语句中使用return语句提前结束方法的执行并返回一个值。因此,从语法上来说,这个代码是没有错误的。
但是,需要根据具体的业务逻辑来判断是否符合预期。如果该方法的语义是需要在循环内部进行一些操作并返回一个结果,那么在if语句中使用return语句可能会导致方法的执行提前结束,导致方法无法完整地执行循环内部的逻辑。此外,如果if语句的条件不满足,那么该方法将不会返回任何结果,这可能会导致一些未知的错误。因此,需要仔细检查代码的逻辑是否符合预期,并确保代码能够正确地执行。
JAVA实现双向循环链表代码
Sure! Here's an example of Java code to implement a doubly circular linked list:
```java
public class DoublyCircularLinkedList {
private Node head;
private int size;
private class Node {
private int data;
private Node previous;
private Node next;
public Node(int data) {
this.data = data;
}
}
// Constructor
public DoublyCircularLinkedList() {
head = null;
size = 0;
}
// Check if the list is empty
public boolean isEmpty() {
return head == null;
}
// Get the size of the list
public int getSize() {
return size;
}
// Add an element to the beginning of the list
public void addFirst(int data) {
Node newNode = new Node(data);
if (isEmpty()) {
newNode.next = newNode;
newNode.previous = newNode;
head = newNode;
} else {
newNode.next = head;
newNode.previous = head.previous;
head.previous.next = newNode;
head.previous = newNode;
head = newNode;
}
size++;
}
// Add an element to the end of the list
public void addLast(int data) {
Node newNode = new Node(data);
if (isEmpty()) {
newNode.next = newNode;
newNode.previous = newNode;
head = newNode;
} else {
newNode.next = head;
newNode.previous = head.previous;
head.previous.next = newNode;
head.previous = newNode;
}
size++;
}
// Remove the first element from the list
public void removeFirst() {
if (isEmpty()) {
System.out.println("List is empty!");
return;
}
if (size == 1) {
head = null;
} else {
head.next.previous = head.previous;
head.previous.next = head.next;
head = head.next;
}
size--;
}
// Remove the last element from the list
public void removeLast() {
if (isEmpty()) {
System.out.println("List is empty!");
return;
}
if (size == 1) {
head = null;
} else {
head.previous.previous.next = head;
head.previous = head.previous.previous;
}
size--;
}
// Display the elements of the list
public void display() {
if (isEmpty()) {
System.out.println("List is empty!");
return;
}
Node current = head;
do {
System.out.print(current.data + " ");
current = current.next;
} while (current != head);
System.out.println();
}
// Main method to test the implementation
public static void main(String[] args) {
DoublyCircularLinkedList list = new DoublyCircularLinkedList();
list.addFirst(3);
list.addFirst(2);
list.addFirst(1);
list.addLast(4);
list.addLast(5);
System.out.println("Elements of the list: ");
list.display();
list.removeFirst();
list.removeLast();
System.out.println("Elements after removing first and last: ");
list.display();
}
}
```
This code creates a DoublyCircularLinkedList class with methods to add elements to the beginning and end, remove elements from the beginning and end, check if the list is empty, get the size of the list, and display the elements of the list. The main method is used to test the implementation by adding elements and then removing the first and last elements.
相关推荐
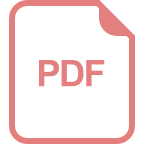
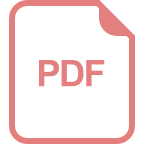
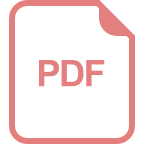












