unordered set 查找
时间: 2023-11-10 18:06:37 浏览: 35
unordered_set是C++标准库中的容器之一,用于存储唯一的元素集合,并且支持快速的插入、删除和查找操作。在unordered_set中,元素的存储顺序是无序的。
要在unordered_set中进行查找操作,可以使用find()函数或者count()函数。find()函数返回一个迭代器,指向目标元素,如果元素不存在,则返回unordered_set的end()迭代器。count()函数返回目标元素在unordered_set中的出现次数,由于unordered_set中的元素是唯一的,所以count()函数最多返回1。
下面是一个示例代码,演示了如何在unordered_set中进行查找:
```cpp
#include <iostream>
#include <unordered_set>
int main() {
std::unordered_set<int> mySet = {1, 2, 3, 4, 5};
// 使用find()函数进行查找
auto it = mySet.find(3);
if (it != mySet.end()) {
std::cout << "Element found: " << *it << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
// 使用count()函数进行查找
int count = mySet.count(6);
if (count > 0) {
std::cout << "Element found" << std::endl;
} else {
std::cout << "Element not found" << std::endl;
}
return 0;
}
```
在上述代码中,我们首先创建了一个包含整数的unordered_set。然后使用find()函数查找元素3,如果找到,则打印出找到的元素;否则,打印出未找到的提示。接下来,使用count()函数查找元素6,如果返回的count大于0,则表示找到了元素;否则,表示未找到。
希望这个示例能够帮助你理解如何使用unordered_set进行查找操作。如果有任何疑问,请随时提问。
相关推荐
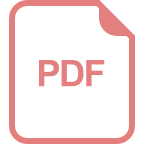
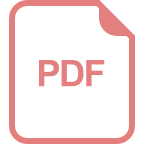
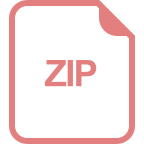
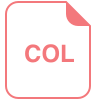
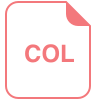












