error: incompatible types: no instance(s) of type variable(s) T exist so that List<T> conforms to Integer
时间: 2024-04-16 22:26:39 浏览: 293
这个错误是由于将一个泛型类型的列表(List<T>)赋值给一个整数类型(Integer)引起的。在Java中,泛型类型是类型安全的,不能将一个泛型类型的列表直接赋值给一个非泛型类型。
要解决这个问题,你需要确保将列表分配给正确的类型。如果你要将列表中的元素转换为整数类型,可以使用循环遍历列表并逐个转换每个元素。例如:
```java
List<Integer> integerList = new ArrayList<>();
List<T> genericList = new ArrayList<>();
// 假设 genericList 中的元素都是整数类型
for (T item : genericList) {
Integer number = (Integer) item; // 将每个元素转换为整数类型
integerList.add(number); // 将转换后的元素添加到整数类型的列表中
}
```
请注意,在进行类型转换时,确保列表中的每个元素都是整数类型,否则可能会抛出ClassCastException异常。
相关问题
Required type: Map <Long, List<Node>> Provided: Map <Integer, List<Node>> no instance(s) of type variable(s) exist so that Integer conforms to Long inference variable K has incompatible bounds: equality constraints: Long
这个错误提示表明在代码中使用了类型不匹配的 `Map` 类型。具体而言,代码期望一个 `Map<Long, List<Node>>` 类型的对象,但是实际传入的是一个 `Map<Integer, List<Node>>` 类型的对象,导致类型不匹配。
根据错误提示,可以看出是因为使用了类型推断时出现了问题。在 Java 中,类型推断是通过编译器自动根据上下文推断出表达式的类型,从而省略类型参数的过程。但是在这个例子中,编译器无法自动推断出正确的类型参数,因此出现了错误。
要解决这个问题,可以明确指定 `Map` 的类型参数,比如使用 `Map<Long, List<Node>>` 替代原来的 `Map` 类型。例如:
```
Map<Long, List<Node>> map = new HashMap<>();
```
或者在方法签名中指定泛型类型参数,比如:
```
public void myMethod(Map<Long, List<Node>> map) {
// ...
}
```
这样可以避免类型不匹配的问题。
Line 4: error: incompatible types: inference variable T has incompatible bounds set.addAll(Arrays.asList(arr)); ^ lower bounds: Integer,Object lower bounds: int[] where T is a type-variable: T extends Object declared in method <T>asList(T...)
这个错误是由于 `Arrays.asList` 方法的参数类型不匹配导致的。
`Arrays.asList` 方法期望接收一个泛型参数 `T` 的可变参数,但在你的代码中,传入的是一个整型数组 `arr`,而不是整型对象。
为了解决这个问题,你可以将整型数组 `arr` 转换为整型对象数组,然后再将其传递给 `Arrays.asList` 方法。这样,就可以保证参数类型与方法的期望类型一致。
修改后的代码如下所示:
```java
set.addAll(Arrays.stream(arr).boxed().collect(Collectors.toList()));
```
在这个修改后的代码中,我们使用 `Arrays.stream(arr)` 将整型数组 `arr` 转换为流,并调用 `boxed()` 将流中的每个元素包装为对应的整型对象。然后,我们使用 `collect(Collectors.toList())` 将整型对象流收集为列表。最后,我们使用 `set.addAll` 将列表中的元素添加到集合中。
这样,你就可以解决“incompatible types: inference variable T has incompatible bounds”的错误。
阅读全文
相关推荐
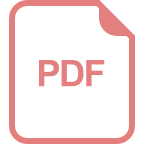
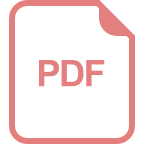
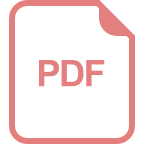













