写一个完整代码,使用using namwspacs std,编写一个二维小数数组类,采用动态内存分配方式,采用分开new的方式编写。通过成员函数计算该数组的方差,返回并打印输出
时间: 2024-12-24 13:28:09 浏览: 7
在C++中,我们可以创建一个二维小数数组类,名为`DecimalMatrix`,采用动态内存分配,并使用命名空间std。以下是一个完整的代码示例:
```cpp
#include <iostream>
#include <vector>
#include <cmath> // 包含计算方差所需的数学库
// 使用std命名空间
using namespace std;
class DecimalMatrix {
private:
int rows;
int cols;
vector<vector<double>>* matrix; // 动态存储二维数组
public:
// 构造函数,接收行数和列数,动态初始化矩阵
DecimalMatrix(int rows_, int cols_) {
rows = rows_;
cols = cols_;
matrix = new vector<vector<double>>(rows, vector<double>(cols, 0.0));
}
// 分开new的方式动态分配元素
double** allocateMemory() {
double** result = new double*[rows];
for (int i = 0; i < rows; ++i) {
result[i] = new double[cols];
}
return result;
}
// 释放动态内存
void deallocateMemory() {
for (int i = 0; i < rows; ++i) {
delete[] matrix->at(i).data(); // 对每个内部向量释放内存
}
delete[] matrix->data(); // 对整个二维向量释放内存
}
// 计算方差
double calculateVariance() {
double sum_of_squares = 0.0;
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
sum_of_squares += pow(matrix->at(i)[j], 2);
}
}
double mean = accumulate(matrix->begin(), matrix->end(), 0.0) / (rows * cols);
return sum_of_squares / (rows * cols) - mean * mean;
}
// 打印矩阵
void printMatrix() {
for (int i = 0; i < rows; ++i) {
for (int j = 0; j < cols; ++j) {
cout << matrix->at(i)[j] << " ";
}
cout << endl;
}
}
// 析构函数,自动调用deallocateMemory释放内存
~DecimalMatrix() {
deallocateMemory();
}
// 主函数,演示如何使用
static void main() {
DecimalMatrix mat(3, 4);
// 初始化矩阵元素...
mat.printMatrix();
double variance = mat.calculateVariance();
cout << "Variance: " << variance << endl;
}
};
```
在这个代码中,我们首先创建了一个`DecimalMatrix`类,包含行数、列数和指向动态分配二维数组的指针。`calculateVariance`函数用于计算方差,`printMatrix`函数用于打印矩阵。主函数展示了如何创建、使用和销毁这个类的对象。
注意:在实际应用中,应该避免全局静态函数`main()`,这里只是为了演示。如果你在其他地方使用这个类,会更合适地在另一个对象上下文中调用`main()`。
阅读全文
相关推荐
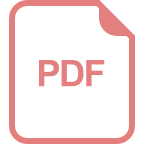
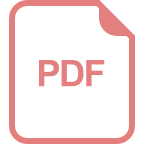
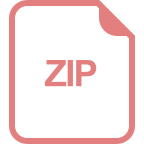
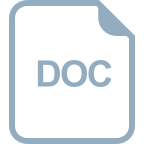
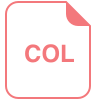














