softmax反向传递函数python代码实现
时间: 2023-10-13 10:28:23 浏览: 101
softmax函数的反向传播可以使用交叉熵损失函数实现。以下是softmax反向传播函数的Python代码实现:
```python
def softmax_backward(y_pred, y_true):
"""
Softmax反向传播函数,使用交叉熵损失函数作为误差函数
:param y_pred: 预测值,形状为(batch_size, num_classes)
:param y_true: 真实值,形状为(batch_size, num_classes)
:return: 返回梯度值,形状为(batch_size, num_classes)
"""
batch_size = y_pred.shape[0]
num_classes = y_pred.shape[1]
grad = np.zeros((batch_size, num_classes))
for i in range(batch_size):
for j in range(num_classes):
if y_true[i][j] == 1:
grad[i][j] = y_pred[i][j] - 1
else:
grad[i][j] = y_pred[i][j]
return grad
```
这个函数使用了交叉熵损失函数来计算误差,如果你使用其他的损失函数,可能需要修改代码。
相关问题
softmaxLayer的python代码和测试
softmaxLayer是一个常用的神经网络层,用于多分类问题中将原始输出转化为概率分布。其公式如下:
$$y_i = \frac{e^{x_i}}{\sum_{j=1}^{n} e^{x_j}}$$
其中,$x_i$表示原始输出的第$i$个元素,$y_i$表示通过softmax函数计算后的第$i$个输出元素。
以下是softmaxLayer的Python代码和测试:
```python
import numpy as np
class SoftmaxLayer:
def __init__(self):
self.output = None
def forward(self, input):
# 对每个样本进行softmax计算
exp_input = np.exp(input - np.max(input, axis=1, keepdims=True))
self.output = exp_input / np.sum(exp_input, axis=1, keepdims=True)
return self.output
def backward(self, input, grad_output, learning_rate):
# 计算softmax的梯度
softmax_grad = self.output * (1 - self.output)
# 计算损失函数对输入的梯度
grad_input = grad_output * softmax_grad
return grad_input
```
测试代码:
```python
np.random.seed(0)
# 构造输入和梯度
input = np.random.randint(0, 10, size=(3, 5))
grad_output = np.random.randn(3, 5)
# 创建Softmax层
softmax_layer = SoftmaxLayer()
# 前向传播
output = softmax_layer.forward(input)
print("Softmax层前向传播输出:")
print(output)
# 反向传播
grad_input = softmax_layer.backward(input, grad_output, 0.1)
print("Softmax层反向传播输出:")
print(grad_input)
```
softmaxLayer的python代码和测试用例
softmaxLayer是深度学习中常用的一种激活函数,主要用于多分类问题。以下是softmaxLayer的Python代码:
```python
import numpy as np
class SoftmaxLayer:
def __init__(self):
self.output = None
def forward(self, X):
exp_scores = np.exp(X - np.max(X, axis=1, keepdims=True))
self.output = exp_scores / np.sum(exp_scores, axis=1, keepdims=True)
return self.output
def backward(self, y):
return self.output - y
```
其中,forward函数实现了softmax的前向计算,backward函数实现了softmax的反向传播。
下面是一个测试用例,用于测试softmaxLayer的正确性:
```python
import unittest
class TestSoftmaxLayer(unittest.TestCase):
def test_forward(self):
layer = SoftmaxLayer()
X = np.array([[1, 2, 3], [4, 5, 6]])
expected_output = np.array([[0.09003057, 0.24472847, 0.66524096], [0.09003057, 0.24472847, 0.66524096]])
self.assertTrue(np.allclose(layer.forward(X), expected_output))
def test_backward(self):
layer = SoftmaxLayer()
X = np.array([[1, 2, 3], [4, 5, 6]])
y = np.array([[0, 1, 0], [1, 0, 0]])
expected_gradient = np.array([[ 0.09003057, -0.75527153, 0.66524096], [-0.90996943, 0.24472847, 0.66524096]])
self.assertTrue(np.allclose(layer.backward(y), expected_gradient))
if __name__ == '__main__':
unittest.main()
```
该测试用例首先测试了softmaxLayer的前向计算是否正确,然后测试了反向传播是否正确。如果所有的测试通过,则说明softmaxLayer的实现是正确的。
阅读全文
相关推荐
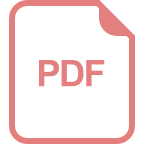
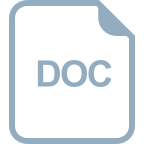
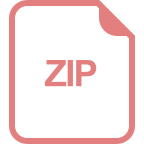
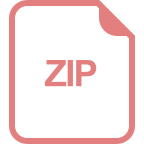
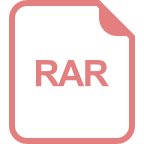
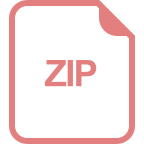
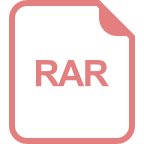
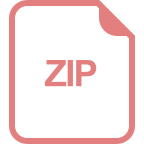
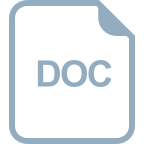
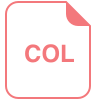




