生成一份ai贪吃蛇的代码
时间: 2023-07-10 21:33:03 浏览: 35
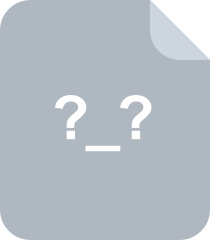
贪吃蛇的代码
好的,以下是一个简单的AI贪吃蛇代码,使用了基本的深度优先搜索算法:
```python
import pygame
import random
import time
# 初始化pygame
pygame.init()
# 设置窗口大小和标题
win_size = (500, 500)
win_title = "AI Snake"
window = pygame.display.set_mode(win_size)
pygame.display.set_caption(win_title)
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
GREEN = (0, 255, 0)
RED = (255, 0, 0)
# 定义贪吃蛇类
class Snake:
def __init__(self):
self.x = 10
self.y = 10
self.direction = "right"
self.length = 1
self.body = [(self.x, self.y)]
def move(self):
if self.direction == "right":
self.x += 10
elif self.direction == "left":
self.x -= 10
elif self.direction == "up":
self.y -= 10
elif self.direction == "down":
self.y += 10
self.body.insert(0, (self.x, self.y))
if len(self.body) > self.length:
self.body.pop()
def change_direction(self, direction):
if direction == "right" and self.direction != "left":
self.direction = "right"
elif direction == "left" and self.direction != "right":
self.direction = "left"
elif direction == "up" and self.direction != "down":
self.direction = "up"
elif direction == "down" and self.direction != "up":
self.direction = "down"
def draw(self, surface):
for x, y in self.body:
pygame.draw.rect(surface, GREEN, [x, y, 10, 10])
def check_collision(self, food):
if self.body[0] == food:
self.length += 1
return True
return False
# 定义食物类
class Food:
def __init__(self):
self.x = random.randrange(0, win_size[0], 10)
self.y = random.randrange(0, win_size[1], 10)
def draw(self, surface):
pygame.draw.rect(surface, RED, [self.x, self.y, 10, 10])
# 定义游戏主循环函数
def game_loop():
# 初始化贪吃蛇和食物
snake = Snake()
food = Food()
# 设置循环标志和计时器
game_over = False
clock = pygame.time.Clock()
# 循环开始
while not game_over:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
game_over = True
# 获取当前贪吃蛇头的位置和方向
head_x, head_y = snake.body[0]
direction = snake.direction
# 计算下一步的位置
if direction == "right":
next_x, next_y = head_x + 10, head_y
elif direction == "left":
next_x, next_y = head_x - 10, head_y
elif direction == "up":
next_x, next_y = head_x, head_y - 10
elif direction == "down":
next_x, next_y = head_x, head_y + 10
# 深度优先搜索算法寻找下一步要走的方向
visited = set(snake.body)
stack = [(next_x, next_y, direction)]
found = False
while stack and not found:
x, y, d = stack.pop()
if (x, y) == food:
found = True
break
for dx, dy, nd in [(10, 0, "right"), (-10, 0, "left"), (0, 10, "down"), (0, -10, "up")]:
nx, ny = x + dx, y + dy
if nx < 0 or nx >= win_size[0] or ny < 0 or ny >= win_size[1] or (nx, ny) in visited:
continue
stack.append((nx, ny, nd))
visited.add((nx, ny))
if found:
snake.change_direction(d)
else:
# 如果找不到路径,随机选择一个方向
possible_directions = ["right", "left", "up", "down"]
possible_directions.remove(snake.direction)
random.shuffle(possible_directions)
for d in possible_directions:
snake.change_direction(d)
nx, ny = snake.body[0]
if nx < 0 or nx >= win_size[0] or ny < 0 or ny >= win_size[1] or (nx, ny) in visited:
continue
break
# 移动贪吃蛇
snake.move()
# 检测碰撞
if snake.check_collision(food=(food.x, food.y)):
food = Food()
# 绘制界面
window.fill(BLACK)
snake.draw(surface=window)
food.draw(surface=window)
pygame.display.update()
# 控制帧率
clock.tick(10)
# 游戏结束,退出pygame
pygame.quit()
# 运行游戏主循环函数
game_loop()
```
这份代码使用了pygame库来绘制游戏界面和处理事件,实现了一个基本的AI贪吃蛇游戏,并使用深度优先搜索算法来控制贪吃蛇的移动。
阅读全文
相关推荐


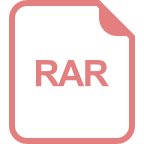
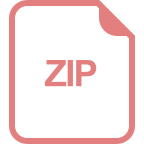
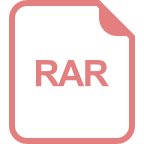
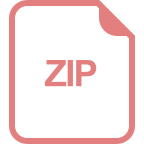
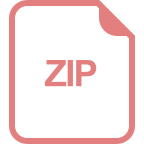
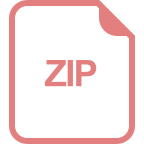
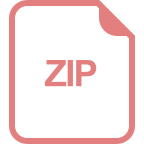
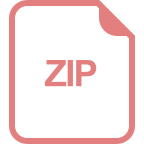
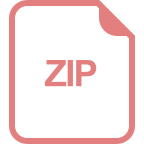
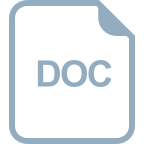
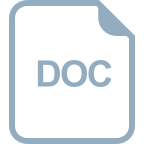
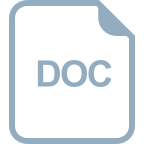
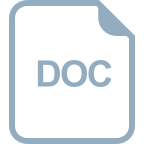
