用DFS实现AI贪吃蛇
时间: 2024-05-16 09:14:27 浏览: 165
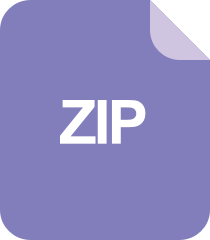
基于Java实现贪吃蛇AI【100011962】

好的,下面是一份基于深度优先搜索算法实现的AI贪吃蛇代码:
```python
import pygame
import random
# 设置游戏场地大小
SIZE = 20
WIDTH = 800
HEIGHT = 600
ROWS = HEIGHT // SIZE
COLUMNS = WIDTH // SIZE
# 定义颜色
WHITE = (255, 255, 255)
BLACK = (0, 0, 0)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义方向
UP = (0, -1)
DOWN = (0, 1)
LEFT = (-1, 0)
RIGHT = (1, 0)
# 初始化pygame
pygame.init()
# 创建游戏窗口
screen = pygame.display.set_mode((WIDTH, HEIGHT))
pygame.display.set_caption('AI贪吃蛇')
# 设置游戏时钟
clock = pygame.time.Clock()
class Snake:
def __init__(self):
self.head = [COLUMNS // 2, ROWS // 2]
self.body = [self.head, [self.head[0], self.head[1] + 1], [self.head[0], self.head[1] + 2]]
self.direction = UP
self.score = 0
def move(self, apple):
# 判断新的蛇头位置是否合法
new_head = [self.head[0] + self.direction[0], self.head[1] + self.direction[1]]
if new_head[0] < 0 or new_head[0] >= COLUMNS or new_head[1] < 0 or new_head[1] >= ROWS or new_head in self.body:
return False
self.head = new_head
self.body.insert(0, self.head)
# 如果吃到苹果,增加分数并生成新的苹果
if self.head == apple:
self.score += 1
return True
else:
self.body.pop()
return False
class Apple:
def __init__(self):
self.position = [random.randint(0, COLUMNS - 1), random.randint(0, ROWS - 1)]
def draw(self):
pygame.draw.rect(screen, RED, (self.position[0] * SIZE, self.position[1] * SIZE, SIZE, SIZE))
def dfs(snake, apple, visited):
# 如果找到苹果,返回路径
if snake.head == apple.position:
return []
# 遍历所有可能的方向
for direction in [UP, DOWN, LEFT, RIGHT]:
new_head = [snake.head[0] + direction[0], snake.head[1] + direction[1]]
if new_head[0] < 0 or new_head[0] >= COLUMNS or new_head[1] < 0 or new_head[1] >= ROWS or new_head in snake.body or new_head in visited:
continue
# 尝试以该方向继续搜索
new_snake = Snake()
new_snake.head = new_head
new_snake.body = [new_head] + snake.body[:-1]
new_snake.direction = direction
visited.append(new_head)
path = dfs(new_snake, apple, visited)
# 如果找到了一条路径,返回路径
if path is not None:
return [new_head] + path
# 没有找到路径,返回空
return None
def update_screen(snake, apple):
# 绘制背景
screen.fill(BLACK)
# 绘制苹果和蛇
apple.draw()
for body_part in snake.body:
pygame.draw.rect(screen, GREEN, (body_part[0] * SIZE, body_part[1] * SIZE, SIZE, SIZE))
# 绘制分数
font = pygame.font.SysFont('arial', 30)
text = font.render('Score: ' + str(snake.score), True, WHITE)
screen.blit(text, (10, 10))
# 更新屏幕
pygame.display.update()
def main():
snake = Snake()
apple = Apple()
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
# 计算下一步方向
visited = []
path = dfs(snake, apple, visited)
if path is not None:
next_direction = [path[0][0] - snake.head[0], path[0][1] - snake.head[1]]
snake.direction = next_direction
# 移动蛇
if not snake.move(apple.position):
break
# 生成新的苹果
apple = Apple()
# 更新屏幕
update_screen(snake, apple)
# 设置游戏时钟
clock.tick(10)
# 显示游戏结束信息
font = pygame.font.SysFont('arial', 50)
text = font.render('Game Over!', True, WHITE)
screen.blit(text, (WIDTH // 2 - text.get_width() // 2, HEIGHT // 2 - text.get_height() // 2))
pygame.display.update()
pygame.time.wait(2000)
# 退出游戏
pygame.quit()
quit()
if __name__ == '__main__':
main()
```
该代码使用深度优先搜索算法计算下一步的方向,以使蛇能够吃到苹果。搜索过程中,如果找到了一条路径,就返回该路径,并将蛇的方向设置为该路径的第一个方向。如果找不到路径,就继续尝试其他方向,直到所有方向都尝试完毕。
阅读全文
相关推荐

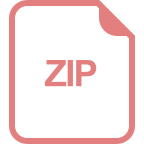
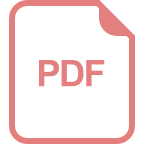
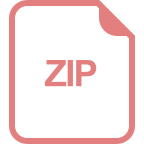
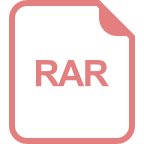
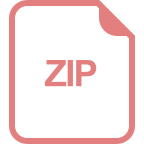
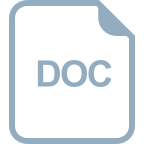
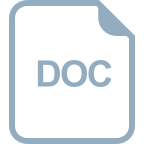
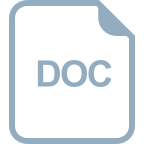
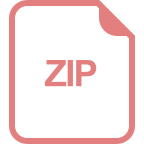
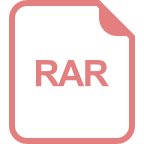
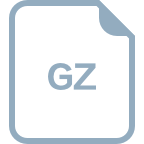
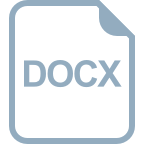
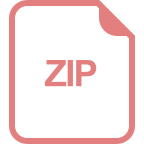
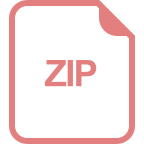
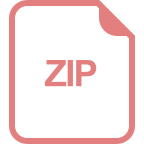