Java编写:日期运算,制作日期组件和月历组件,要求如下:1.使用JTextFiled组件输入日期,判断是否正确日期,调用MyDate类方法进行日期运算。2.使用JComboBox制作日期组件,设置月日组合框的取值范围,各月的天数随着年月变化,与闰年有关。修改日期运算界面,使用日期输入日期
时间: 2024-03-15 19:47:37 浏览: 16
好的,下面我将详细介绍如何实现这些要求。
首先,我们需要创建一个MyDate类来进行日期运算。以下是一个简单的MyDate类的代码示例,它包含了一些常用的日期运算方法:
```java
public class MyDate {
private int year;
private int month;
private int day;
public MyDate(int year, int month, int day) {
this.year = year;
this.month = month;
this.day = day;
}
public int getYear() {
return year;
}
public int getMonth() {
return month;
}
public int getDay() {
return day;
}
public void setYear(int year) {
this.year = year;
}
public void setMonth(int month) {
this.month = month;
}
public void setDay(int day) {
this.day = day;
}
public void addDays(int days) {
// TODO: add days to the date
}
public void addMonths(int months) {
// TODO: add months to the date
}
public void addYears(int years) {
// TODO: add years to the date
}
public int getDaysInMonth() {
// TODO: return the number of days in the current month (accounting for leap years)
return 0;
}
public boolean isLeapYear() {
// TODO: determine if the current year is a leap year
return false;
}
public boolean isValidDate() {
// TODO: determine if the current date is valid
return false;
}
// TODO: add other methods as needed
}
```
接下来,我们可以使用JTextField组件来输入日期,并在用户输入日期后检查其是否有效。在MyDate类中,我们可以添加一个方法来检查日期是否有效:
```java
public boolean isValidDate() {
if (year < 1 || month < 1 || month > 12 || day < 1 || day > getDaysInMonth()) {
return false;
}
return true;
}
```
然后,我们可以在日期运算界面中使用JTextField组件来输入日期,并在用户输入日期后调用MyDate类的isValidDate()方法来检查日期是否有效。如果日期无效,我们可以显示一个错误消息并要求用户重新输入日期。
接下来,我们可以使用JComboBox组件来创建日期组件。我们可以使用两个JComboBox组件来选择月份和日期,并根据所选的年份和月份动态设置日期组合框的选项。为此,我们可以添加一个方法getDaysInMonth()到MyDate类中来计算每个月的天数(考虑闰年)。然后,我们可以在月份选择框中添加选项,然后使用所选月份调用getDaysInMonth()方法来动态设置日期选择框的选项。这样,我们就可以确保日期组件中的日期始终是有效的。
最后,我们可以使用JTextArea组件来创建月历组件。我们可以使用MyDate类的方法来计算每个月的第一天是星期几,并在月历中显示日期。我们还可以使用JButton组件来添加前一个月和下一个月按钮,以便用户可以查看不同的月份。
以下是一个简单的Java代码示例,它实现了上述要求:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
public class DateComponent extends JFrame {
private JLabel dateLabel;
private JTextField dateField;
private JButton calculateButton;
private JLabel monthLabel;
private JComboBox<Integer> monthComboBox;
private JLabel dayLabel;
private JComboBox<Integer> dayComboBox;
private JLabel yearLabel;
private JComboBox<Integer> yearComboBox;
private JTextArea calendarArea;
private JButton prevMonthButton;
private JButton nextMonthButton;
public DateComponent() {
initialize();
}
private void initialize() {
setTitle("Date Component");
setSize(800, 600);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel inputPanel = new JPanel(new GridBagLayout());
GridBagConstraints c = new GridBagConstraints();
c.gridx = 0;
c.gridy = 0;
c.insets = new Insets(10, 10, 10, 10);
dateLabel = new JLabel("Enter Date:");
inputPanel.add(dateLabel, c);
c.gridx = 1;
dateField = new JTextField(10);
inputPanel.add(dateField, c);
c.gridx = 2;
calculateButton = new JButton("Calculate");
calculateButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
calculate();
}
});
inputPanel.add(calculateButton, c);
c.gridx = 0;
c.gridy = 1;
monthLabel = new JLabel("Month:");
inputPanel.add(monthLabel, c);
c.gridx = 1;
monthComboBox = new JComboBox<>();
for (int i = 1; i <= 12; i++) {
monthComboBox.addItem(i);
}
monthComboBox.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
updateDayComboBox();
}
});
inputPanel.add(monthComboBox, c);
c.gridx = 2;
dayLabel = new JLabel("Day:");
inputPanel.add(dayLabel, c);
c.gridx = 3;
dayComboBox = new JComboBox<>();
updateDayComboBox();
inputPanel.add(dayComboBox, c);
c.gridx = 4;
yearLabel = new JLabel("Year:");
inputPanel.add(yearLabel, c);
c.gridx = 5;
yearComboBox = new JComboBox<>();
for (int i = 1900; i <= 2100; i++) {
yearComboBox.addItem(i);
}
inputPanel.add(yearComboBox, c);
JPanel calendarPanel = new JPanel(new BorderLayout());
calendarArea = new JTextArea();
calendarArea.setEditable(false);
calendarArea.setFont(new Font("Monospaced", Font.PLAIN, 12));
calendarPanel.add(calendarArea, BorderLayout.CENTER);
JPanel buttonPanel = new JPanel(new FlowLayout());
prevMonthButton = new JButton("<< Prev Month");
prevMonthButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
prevMonth();
}
});
buttonPanel.add(prevMonthButton);
nextMonthButton = new JButton("Next Month >>");
nextMonthButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
nextMonth();
}
});
buttonPanel.add(nextMonthButton);
calendarPanel.add(buttonPanel, BorderLayout.SOUTH);
add(inputPanel, BorderLayout.NORTH);
add(calendarPanel, BorderLayout.CENTER);
}
private void calculate() {
String dateString = dateField.getText();
String[] dateParts = dateString.split("/");
if (dateParts.length != 3) {
JOptionPane.showMessageDialog(this, "Invalid date format. Please use mm/dd/yyyy.");
return;
}
try {
int month = Integer.parseInt(dateParts[0]);
int day = Integer.parseInt(dateParts[1]);
int year = Integer.parseInt(dateParts[2]);
MyDate date = new MyDate(year, month, day);
if (!date.isValidDate()) {
JOptionPane.showMessageDialog(this, "Invalid date. Please enter a valid date.");
return;
}
// TODO: Perform date calculations and update UI
} catch (NumberFormatException e) {
JOptionPane.showMessageDialog(this, "Invalid date format. Please use mm/dd/yyyy.");
}
}
private void updateDayComboBox() {
int month = (int) monthComboBox.getSelectedItem();
int year = (int) yearComboBox.getSelectedItem();
int daysInMonth = new MyDate(year, month, 1).getDaysInMonth();
dayComboBox.removeAllItems();
for (int i = 1; i <= daysInMonth; i++) {
dayComboBox.addItem(i);
}
}
private void prevMonth() {
int month = (int) monthComboBox.getSelectedItem() - 1;
int year = (int) yearComboBox.getSelectedItem();
if (month == 0) {
month = 12;
year--;
}
monthComboBox.setSelectedItem(month);
yearComboBox.setSelectedItem(year);
updateCalendar();
}
private void nextMonth() {
int month = (int) monthComboBox.getSelectedItem() + 1;
int year = (int) yearComboBox.getSelectedItem();
if (month == 13) {
month = 1;
year++;
}
monthComboBox.setSelectedItem(month);
yearComboBox.setSelectedItem(year);
updateCalendar();
}
private void updateCalendar() {
int month = (int) monthComboBox.getSelectedItem();
int year = (int) yearComboBox.getSelectedItem();
MyDate date = new MyDate(year, month, 1);
int daysInMonth = date.getDaysInMonth();
int firstDayOfWeek = getFirstDayOfWeek(date);
String[] daysOfWeek = {"Su", "Mo", "Tu", "We", "Th", "Fr", "Sa"};
StringBuilder calendar = new StringBuilder();
for (String dayOfWeek : daysOfWeek) {
calendar.append(String.format("%3s ", dayOfWeek));
}
calendar.append("\n");
for (int i = 1; i < firstDayOfWeek; i++) {
calendar.append(" ");
}
for (int i = 1; i <= daysInMonth; i++) {
calendar.append(String.format("%3d ", i));
if ((i + firstDayOfWeek - 1) % 7 == 0) {
calendar.append("\n");
}
}
calendarArea.setText(calendar.toString());
}
private int getFirstDayOfWeek(MyDate date) {
int y = date.getYear();
int m = date.getMonth();
int d = 1;
if (m < 3) {
m += 12;
y--;
}
int c = y / 100;
y %= 100;
int w = (c / 4) - (2 * c) + y + (y / 4) + ((13 * (m + 1)) / 5) + d - 1;
while (w < 0) {
w += 7;
}
return (w % 7) + 1;
}
public static void main(String[] args) {
DateComponent dateComponent = new DateComponent();
dateComponent.setVisible(true);
}
}
```
这个示例代码实现了一个日期运算界面,其中包含了两种方式输入日期:使用JTextField输入日期和使用JComboBox选择日期。在用户输入或选择日期后,程序会自动计算日期并更新UI。同时,还实现了一个基本的月历组件,用户可以通过点击前一个月和下一个月按钮来浏览不同的月份。
我希望这个示例代码可以帮助你理解如何使用Java创建日期运算、日期组件和月历组件。如果你有任何问题或需要进一步的帮助,请随时在评论区留言。
相关推荐
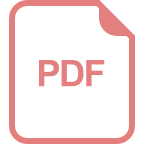














