def tableRowCounter(s): soup = BeautifulSoup(s, 'html.parser') table = soup.find('table') rows = table.find_all('tr') header_row = rows[0] non_header_rows = rows[1:] return len(non_header_rows)输入s ="<body><table><tr><td>1</td><td>1</td></tr><tr><td>1</td><td>1</td></tr><tr><td>1</td><td>1</td></tr></table><body>" assert tableRowCounter(s)==3时会出错
时间: 2023-06-16 09:03:06 浏览: 496
在你提供的代码中,出现了一个错误,就是在返回非表头行数之前,没有对表是否存在进行检查。如果表不存在,代码将会抛出异常。为了解决这个问题,可以使用以下代码:
```python
def tableRowCounter(s):
soup = BeautifulSoup(s, 'html.parser')
table = soup.find('table')
if not table:
return 0
rows = table.find_all('tr')
header_row = rows[0]
non_header_rows = rows[1:]
return len(non_header_rows)
```
在这个版本的代码中,我们在查找表格之后进行了检查。如果表格不存在,我们就返回0,否则我们按照之前的方式计算非表头行数。这个版本的代码应该可以正常运行,而且在没有表格的情况下,也不会抛出异常。
相关问题
帮我改错import bs4 def tableRowCounter(s): soup = BeautifulSoup(s, 'html.parser') table = soup.find('table') if not table: return 0 rows = table.find_all('tr') count = 0 for i in len(rows): if len(rows) > 0 and rows[i].find('th'): break count+=1 return count
import requests
from bs4 import BeautifulSoup
def tableRowCounter(s):
soup = BeautifulSoup(s, 'html.parser')
table = soup.find('table')
if not table:
return 0
rows = table.find_all('tr')
count = 0
for i in range(len(rows)):
if len(rows[i]) > 0 and rows[i].find('th'):
break
count += 1
return count
# 这里的 import bs4 是多余的,因为 bs4 已经在第二行中被导入了,可以直接使用 bs4 的模块
# 另外,for 循环中应该使用 range(len(rows)) 来获取索引值,而不是使用 len(rows) 来获取元素数量
帮我改错:import bs4 def tableRowCounter(s): soup = BeautifulSoup(s, 'html.parser') table = soup.find('table') if not table: return 0 rows = table.find_all('tr') count = 0 for i in len(rows): if len(rows) > 0 and rows[i].find('th'): break count+=1 return count
代码中的错误在于 `for i in len(rows)` 这一行,应该改为 `for i in range(len(rows))`。
修改后的代码如下:
```python
import bs4
from bs4 import BeautifulSoup
def tableRowCounter(s):
soup = BeautifulSoup(s, 'html.parser')
table = soup.find('table')
if not table:
return 0
rows = table.find_all('tr')
count = 0
for i in range(len(rows)):
if len(rows) > 0 and rows[i].find('th'):
break
count += 1
return count
```
这样就可以正确地计算出表格的行数了。
阅读全文
相关推荐
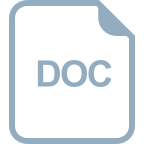
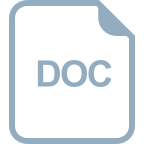
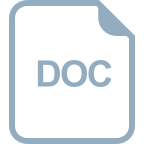













