写一段C语言程序实现1-100加法
时间: 2023-06-08 10:04:56 浏览: 66
Sure, here is a C program that implements the sum of numbers from 1 to 100:
```
#include <stdio.h>
int main() {
int i, sum = 0;
for (i = 1; i <= 100; i++) {
sum += i;
}
printf("The sum of numbers from 1 to 100 is %d", sum);
return 0;
}
```
Hope that helps!
相关问题
写一段加法运算的C语言程序
当然,下面是一个简单的加法运算的C语言程序示例:
```
#include <stdio.h>
int main() {
int num1, num2, sum;
printf("请输入两个数字:\n");
scanf("%d %d", &num1, &num2);
sum = num1 + num2;
printf("%d + %d = %d\n", num1, num2, sum);
return 0;
}
```
该程序先提示用户输入两个数字,然后通过scanf()函数读取用户输入的数字,并将它们相加保存到sum变量中。最后,程序使用printf()函数输出计算结果。
用c语言写一段随机生成10以内的加法
好的,以下是一段用 C 语言编写的随机生成 10 以内的加法程序:
```c
#include<stdio.h>
#include<stdlib.h>
#include<time.h>
int main(){
int num1, num2, answer, userAnswer;
srand(time(NULL)); // 设置随机数种子
num1 = rand() % 11; // 生成 0 ~ 10 的随机数
num2 = rand() % 11; // 生成 0 ~ 10 的随机数
answer = num1 + num2; // 计算正确答案
printf("请回答以下的问题:\n");
printf("%d + %d = ", num1, num2);
scanf("%d", &userAnswer);
if(userAnswer == answer){
printf("回答正确!\n");
}
else{
printf("回答错误!正确答案是 %d\n", answer);
}
return 0;
}
```
程序中使用了 `rand()` 函数和 `srand()` 函数来生成随机数,使用了 `time(NULL)` 来设置随机数种子,保证每次程序运行时生成的随机数都不同。程序生成两个 0 到 10 的随机数,计算它们的和,并提示用户回答问题。如果用户回答正确,程序输出 "回答正确!",否则输出 "回答错误!" 并显示正确答案。
相关推荐
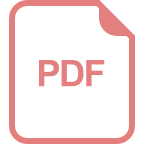
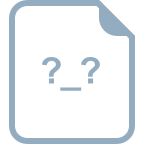
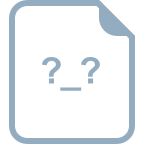












