unity 图片循环切换,中间放大,两边收小程序代码
时间: 2023-07-20 14:34:21 浏览: 48
以下是一个示例代码,展示如何在Unity中实现图片循环切换、中间放大和两边收小的效果:
```csharp
using UnityEngine;
using System.Collections;
public class ImageCycle : MonoBehaviour {
public Texture[] textures; // 存储图片的数组
public float cycleTime = 2.0f; // 图片循环切换的时间间隔
public float zoomTime = 0.5f; // 图片缩放的时间
public float zoomScale = 2.0f; // 图片放大的比例
public float moveSpeed = 0.5f; // 图片移动的速度
private int currentTextureIndex = 0; // 当前显示的图片索引
private float cycleTimer = 0.0f; // 图片循环切换的计时器
private bool isZooming = false; // 是否正在缩放图片
private Vector3 originalScale; // 图片原始的缩放比例
private Vector3 targetScale; // 图片目标的缩放比例
void Start () {
// 初始化图片缩放的参数
originalScale = transform.localScale;
targetScale = originalScale * zoomScale;
}
void Update () {
// 更新图片循环切换的计时器
cycleTimer += Time.deltaTime;
if (cycleTimer >= cycleTime) {
cycleTimer = 0.0f;
SwitchTexture();
}
// 如果正在缩放图片,则更新图片的缩放
if (isZooming) {
float zoomProgress = Mathf.PingPong(Time.time, zoomTime) / zoomTime;
transform.localScale = Vector3.Lerp(originalScale, targetScale, zoomProgress);
}
else {
transform.localScale = originalScale;
}
}
// 切换图片
void SwitchTexture() {
currentTextureIndex++;
if (currentTextureIndex >= textures.Length) {
currentTextureIndex = 0;
}
// 计算图片移动目标位置
Vector3 targetPosition = transform.position;
if (textures.Length > 1) {
if (currentTextureIndex == 0) {
targetPosition.x += moveSpeed * textures.Length;
}
else if (currentTextureIndex == textures.Length - 1) {
targetPosition.x -= moveSpeed * textures.Length;
}
else if (currentTextureIndex > 0 && currentTextureIndex < textures.Length - 1) {
if (currentTextureIndex % 2 == 0) {
targetPosition.x -= moveSpeed;
}
else {
targetPosition.x += moveSpeed;
}
}
}
// 改变图片的贴图
renderer.material.mainTexture = textures[currentTextureIndex];
// 启动图片缩放的动画
StartCoroutine(ZoomImage(targetPosition));
}
// 图片缩放的动画
IEnumerator ZoomImage(Vector3 targetPosition) {
isZooming = true;
Vector3 originalPosition = transform.position;
float zoomTimer = 0.0f;
while (zoomTimer < zoomTime) {
zoomTimer += Time.deltaTime;
float zoomProgress = zoomTimer / zoomTime;
transform.position = Vector3.Lerp(originalPosition, targetPosition, zoomProgress);
yield return null;
}
transform.position = targetPosition;
isZooming = false;
}
}
```
上面的代码中,使用了一个`Texture`数组来存储要循环切换的图片,使用了一个计时器来控制图片循环切换的时间间隔,使用了`Lerp`函数来实现图片的缩放和移动。在`SwitchTexture`方法中,先计算出图片移动的目标位置,然后通过`renderer.material.mainTexture`来切换图片,最后启动一个协程来实现图片缩放的动画。在`ZoomImage`协程中,使用了`Lerp`函数来实现图片的缩放和移动,并使用了`yield return null`语句来使协程等待一帧时间,以便让Unity执行其他的操作。
相关推荐
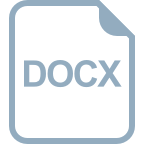














