class Block: def __init__(self, index, transaction, previous_hash): self.index = index self.timestamp = time() self.previous_hash = previous_hash self.transaction = transaction def compute_hash(self): concat_str = str(self.index) + str(self.timestamp) + str(self.previous_hash) + str(self.transaction['author']) + str(self.transaction['genre']) hash_result = hasher.sha256(concat_str.encode('utf-8')).hexdigest() return hash_result def serialize(self): return { 'index': self.index, 'timestamp': self.timestamp, 'previous_hash': self.previous_hash, 'transaction': self.transaction }
时间: 2024-04-27 12:25:34 浏览: 9
这段代码定义了一个区块类Block,用于创建区块链上的区块对象。该类包含以下属性:
1. index:区块在区块链中的索引。
2. timestamp:区块生成的时间戳。
3. previous_hash:前一个区块的哈希值。
4. transaction:该区块包含的交易信息。
该类的初始化方法__init__()用于初始化区块对象的属性值。compute_hash()方法用于计算区块的哈希值,通常使用SHA-256算法进行计算。serialize()方法用于将区块序列化为字典格式,以便存储在区块链中。
通过定义区块类,我们可以轻松地在区块链上存储和管理交易信息。每个区块都包含有关交易的信息和前一个区块的哈希值,以确保交易的安全性和完整性。
相关问题
区块源代码_200行代码构建一个区块链
很高兴回答您的问题。下面是200行代码构建一个简单的区块链的示例:
```python
import hashlib
import json
from time import time
class Blockchain(object):
def __init__(self):
self.chain = []
self.current_transactions = []
# Create the genesis block
self.new_block(previous_hash=1, proof=100)
def new_block(self, proof, previous_hash=None):
"""
Create a new Block in the Blockchain
:param proof: <int> The proof given by the Proof of Work algorithm
:param previous_hash: (Optional) <str> Hash of previous Block
:return: <dict> New Block
"""
block = {
'index': len(self.chain) + 1,
'timestamp': time(),
'transactions': self.current_transactions,
'proof': proof,
'previous_hash': previous_hash or self.hash(self.chain[-1]),
}
# Reset the current list of transactions
self.current_transactions = []
self.chain.append(block)
return block
def new_transaction(self, sender, recipient, amount):
"""
Creates a new transaction to go into the next mined Block
:param sender: <str> Address of the Sender
:param recipient: <str> Address of the Recipient
:param amount: <int> Amount
:return: <int> The index of the Block that will hold this transaction
"""
self.current_transactions.append({
'sender': sender,
'recipient': recipient,
'amount': amount,
})
return self.last_block['index'] + 1
@staticmethod
def hash(block):
"""
Creates a SHA-256 hash of a Block
:param block: <dict> Block
:return: <str>
"""
# We must make sure that the Dictionary is Ordered, or we'll have inconsistent hashes
block_string = json.dumps(block, sort_keys=True).encode()
return hashlib.sha256(block_string).hexdigest()
@property
def last_block(self):
return self.chain[-1]
def proof_of_work(self, last_proof):
"""
Simple Proof of Work Algorithm:
- Find a number p' such that hash(pp') contains leading 4 zeroes, where p is the previous p'
- p is the previous proof, and p' is the new proof
:param last_proof: <int>
:return: <int>
"""
proof = 0
while self.valid_proof(last_proof, proof) is False:
proof += 1
return proof
@staticmethod
def valid_proof(last_proof, proof):
"""
Validates the Proof: Does hash(last_proof, proof) contain 4 leading zeroes?
:param last_proof: <int> Previous Proof
:param proof: <int> Current Proof
:return: <bool> True if correct, False if not.
"""
guess = f'{last_proof}{proof}'.encode()
guess_hash = hashlib.sha256(guess).hexdigest()
return guess_hash[:4] == "0000"
```
这是一个区块链的基本数据结构和功能。您可以使用该代码进行测试和开发。
(二)区块链的共识算法:PoS 及其 例子 代码 实现
PoS(Proof of Stake)是一种区块链的共识算法,它通过锁定一定数量的代币来决定区块的生成权,相比于 PoW(Proof of Work)更加节能且不容易被攻击。下面给出一个简单的 PoS 示例代码实现:
```
import hashlib
import random
class Block:
def __init__(self, index, timestamp, data, previous_hash, validator):
self.index = index
self.timestamp = timestamp
self.data = data
self.previous_hash = previous_hash
self.validator = validator
self.hash = self.calculate_hash()
def calculate_hash(self):
return hashlib.sha256(str(self.index).encode('utf-8') +
str(self.timestamp).encode('utf-8') +
str(self.data).encode('utf-8') +
str(self.previous_hash).encode('utf-8') +
str(self.validator).encode('utf-8')).hexdigest()
class Blockchain:
def __init__(self):
self.chain = [self.create_genesis_block()]
self.nodes = []
def create_genesis_block(self):
return Block(0, '01/01/2020', 'Genesis Block', '0', None)
def add_node(self, node):
self.nodes.append(node)
def get_validator(self):
total_stake = sum([node.stake for node in self.nodes])
winning_num = random.uniform(0, total_stake)
current_num = 0
for node in self.nodes:
current_num += node.stake
if current_num > winning_num:
return node
def add_block(self, data):
previous_block = self.chain[-1]
validator = self.get_validator()
new_block = Block(len(self.chain),
str(datetime.datetime.now()),
data,
previous_block.hash,
validator)
self.chain.append(new_block)
print(f"Block {new_block.index} has been added to the chain by {validator.name}.\n")
class Node:
def __init__(self, name, stake):
self.name = name
self.stake = stake
if __name__ == '__main__':
blockchain = Blockchain()
node1 = Node('Alice', 100)
node2 = Node('Bob', 50)
node3 = Node('Charlie', 150)
blockchain.add_node(node1)
blockchain.add_node(node2)
blockchain.add_node(node3)
blockchain.add_block('Transaction A')
blockchain.add_block('Transaction B')
blockchain.add_block('Transaction C')
```
在上面的代码中,我们定义了一个 Block 类来表示区块,包含索引、时间戳、数据、前一个区块的哈希值和验证者等属性,以及一个 calculate_hash 方法来计算区块的哈希值。同时,我们还定义了一个 Blockchain 类来表示区块链,包含链和参与 PoS 共识的节点列表两个属性,以及 create_genesis_block、add_node、get_validator、add_block 等方法。其中,get_validator 方法用于随机选择一个验证者节点,add_block 方法用于添加新的区块并指定验证者。最后,在主程序中我们创建了三个节点,并添加了三个区块,每个区块的验证者都是随机选择的。
相关推荐
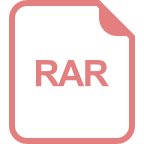









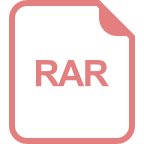
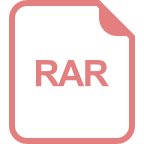