list_for_each_entry含义以及示例
时间: 2023-07-31 07:10:18 浏览: 336
`list_for_each_entry` 是 Linux 内核中用于遍历双向链表的宏,它可以在链表中遍历每个节点,并且可以访问每个节点的成员。
它的语法如下:
```
list_for_each_entry(pos, head, member)
```
其中,`pos` 是遍历链表时当前节点的指针,`head` 是链表头指针,`member` 是链表节点中指向下一个节点的成员指针。
下面是一个示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <stddef.h>
#include "list.h"
struct student {
char name[20];
int age;
struct list_head list;
};
int main()
{
struct student *stu1, *stu2, *stu3;
struct student *pos;
struct list_head *head;
int i;
// 初始化链表头
head = (struct list_head *)malloc(sizeof(struct list_head));
INIT_LIST_HEAD(head);
// 添加节点到链表
stu1 = (struct student *)malloc(sizeof(struct student));
strcpy(stu1->name, "Tom");
stu1->age = 18;
list_add_tail(&stu1->list, head);
stu2 = (struct student *)malloc(sizeof(struct student));
strcpy(stu2->name, "Jerry");
stu2->age = 20;
list_add_tail(&stu2->list, head);
stu3 = (struct student *)malloc(sizeof(struct student));
strcpy(stu3->name, "Mike");
stu3->age = 22;
list_add_tail(&stu3->list, head);
// 遍历链表
printf("遍历链表:\n");
list_for_each_entry(pos, head, list) {
printf("name: %s, age: %d\n", pos->name, pos->age);
}
// 释放节点
list_for_each_entry(pos, head, list) {
list_del(&pos->list);
free(pos);
}
// 释放链表头
free(head);
return 0;
}
```
输出结果:
```
遍历链表:
name: Tom, age: 18
name: Jerry, age: 20
name: Mike, age: 22
```
阅读全文
相关推荐
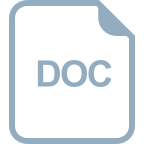
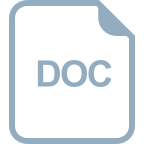
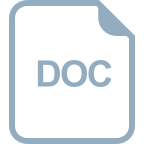






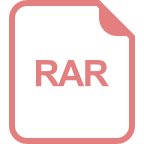
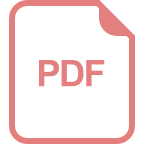
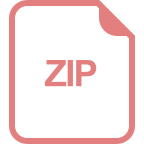
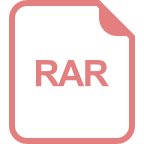
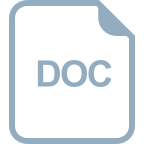
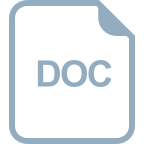
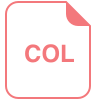
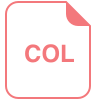
