用C语言写一个简单的代码,要求为:编写一个读入一个字符串,把它顺序读入队列,并输出队列 的程序。
时间: 2024-02-01 09:14:11 浏览: 32
以下是用C语言实现读入字符串并顺序读入队列,最后输出队列的代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#define MAX_LEN 100
typedef struct node {
char data;
struct node *next;
} Node;
typedef struct {
Node *front;
Node *rear;
} Queue;
void initQueue(Queue *q) {
q->front = q->rear = NULL;
}
int isEmpty(Queue *q) {
return q->front == NULL;
}
void enQueue(Queue *q, char data) {
Node *p = (Node *) malloc(sizeof(Node));
p->data = data;
p->next = NULL;
if (isEmpty(q)) {
q->front = q->rear = p;
} else {
q->rear->next = p;
q->rear = p;
}
}
void deQueue(Queue *q, char *data) {
if (isEmpty(q)) {
printf("Queue is empty\n");
exit(1);
}
Node *p = q->front;
*data = p->data;
q->front = p->next;
free(p);
if (q->front == NULL) {
q->rear = NULL;
}
}
void printQueue(Queue *q) {
char data;
while (!isEmpty(q)) {
deQueue(q, &data);
printf("%c", data);
}
}
int main() {
char str[MAX_LEN];
printf("Enter a string: ");
fgets(str, MAX_LEN, stdin);
Queue q;
initQueue(&q);
for (int i = 0; str[i] != '\0'; i++) {
enQueue(&q, str[i]);
}
printf("Queue: ");
printQueue(&q);
return 0;
}
```
首先,我们定义了一个队列结构体类型,其中包含一个指向队首和队尾的指针。然后,我们实现了初始化队列、判断队列是否为空、入队、出队、输出队列的函数。在主函数中,我们先读入一个字符串,然后将其顺序入队,并最后输出队列。
相关推荐
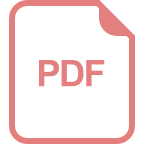
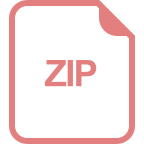
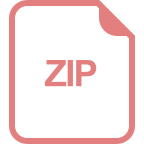














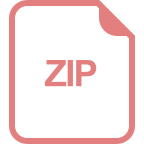