用python生成一个温标转换的代码
时间: 2023-09-15 13:05:46 浏览: 400
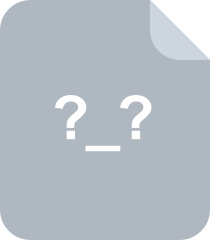
用python实现华氏温度何摄氏温度转换

### 回答1:
下面是一个将摄氏温度转换为华氏温度的 Python 代码:
```
def celsius_to_fahrenheit(celsius):
fahrenheit = (celsius * 9/5) + 32
return fahrenheit
```
使用方法:
```
temperature_in_celsius = 37.5
temperature_in_fahrenheit = celsius_to_fahrenheit(temperature_in_celsius)
print("Temperature in Fahrenheit:", temperature_in_fahrenheit)
```
输出:
```
Temperature in Fahrenheit: 99.5
```
### 回答2:
温标转换是指将摄氏度(Celsius)、华氏度(Fahrenheit)和开氏度(Kelvin)之间进行转换的计算过程。
下面是一个用Python编写的温标转换程序:
```python
# 定义温标转换函数
def temperature_conversion():
# 提示用户输入转换类型
print("请选择转换类型:")
print("1. 摄氏度 -> 华氏度")
print("2. 华氏度 -> 摄氏度")
print("3. 摄氏度 -> 开氏度")
print("4. 开氏度 -> 摄氏度")
print("5. 华氏度 -> 开氏度")
print("6. 开氏度 -> 华氏度")
choice = int(input("请输入选择的转换类型(输入相应的数字):"))
if choice == 1:
celsius = float(input("请输入摄氏度:"))
fahrenheit = (celsius * 9/5) + 32
print("摄氏度 %.2f 度转换为华氏度为 %.2f 度" % (celsius, fahrenheit))
elif choice == 2:
fahrenheit = float(input("请输入华氏度:"))
celsius = (fahrenheit - 32) * 5/9
print("华氏度 %.2f 度转换为摄氏度为 %.2f 度" % (fahrenheit, celsius))
elif choice == 3:
celsius = float(input("请输入摄氏度:"))
kelvin = celsius + 273.15
print("摄氏度 %.2f 度转换为开氏度为 %.2f 度" % (celsius, kelvin))
elif choice == 4:
kelvin = float(input("请输入开氏度:"))
celsius = kelvin - 273.15
print("开氏度 %.2f 度转换为摄氏度为 %.2f 度" % (kelvin, celsius))
elif choice == 5:
fahrenheit = float(input("请输入华氏度:"))
kelvin = (fahrenheit + 459.67) * 5/9
print("华氏度 %.2f 度转换为开氏度为 %.2f 度" % (fahrenheit, kelvin))
elif choice == 6:
kelvin = float(input("请输入开氏度:"))
fahrenheit = kelvin * 9/5 - 459.67
print("开氏度 %.2f 度转换为华氏度为 %.2f 度" % (kelvin, fahrenheit))
else:
print("无效选择!")
# 调用温标转换函数
temperature_conversion()
```
使用该程序,用户可以根据提示选择需要进行的温标转换类型,然后输入相应的温度值,程序会自动将输入的温度进行转换并输出结果。
### 回答3:
温标转换是指将温度从一种温标单位转换到另一种温标单位。下面是一个使用Python编写的温标转换代码:
```python
def celsius_to_fahrenheit(celsius):
fahrenheit = (celsius * 9/5) + 32
return fahrenheit
def fahrenheit_to_celsius(fahrenheit):
celsius = (fahrenheit - 32) * 5/9
return celsius
def celsius_to_kelvin(celsius):
kelvin = celsius + 273.15
return kelvin
def kelvin_to_celsius(kelvin):
celsius = kelvin - 273.15
return celsius
def fahrenheit_to_kelvin(fahrenheit):
kelvin = (fahrenheit + 459.67) * 5/9
return kelvin
def kelvin_to_fahrenheit(kelvin):
fahrenheit = (kelvin * 9/5) - 459.67
return fahrenheit
# 测试代码
celsius = 30
print(celsius, "摄氏度转换为华氏度:", celsius_to_fahrenheit(celsius), "华氏度")
fahrenheit = 86
print(fahrenheit, "华氏度转换为摄氏度:", fahrenheit_to_celsius(fahrenheit), "摄氏度")
celsius = 30
print(celsius, "摄氏度转换为开尔文:", celsius_to_kelvin(celsius), "开尔文")
kelvin = 303.15
print(kelvin, "开尔文转换为摄氏度:", kelvin_to_celsius(kelvin), "摄氏度")
fahrenheit = 86
print(fahrenheit, "华氏度转换为开尔文:", fahrenheit_to_kelvin(fahrenheit), "开尔文")
kelvin = 300
print(kelvin, "开尔文转换为华氏度:", kelvin_to_fahrenheit(kelvin), "华氏度")
```
以上代码定义了六个函数分别用于各种温标转换,并附带了一些测试代码。你可以根据自己的需要传入不同的温度值进行测试。
阅读全文
相关推荐
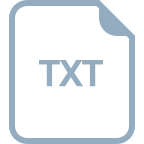
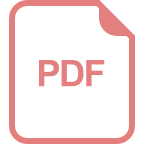















