实现一个方法,遍历angular,router模块里的config对象
时间: 2023-09-06 13:04:03 浏览: 235
### 回答1:
你可以使用 Angular 的 Dependency Injection (DI) 来注入路由器服务,然后在你的方法里获取路由器的配置对象。
首先,你需要在你的组件或服务的构造函数里注入路由器服务:
```
constructor(private router: Router) {}
```
然后,你可以使用路由器服务的 `config` 属性来访问路由器的配置对象:
```
let config = this.router.config;
```
你可以使用 JavaScript 的 `for...of` 循环或者 `Array.forEach()` 方法来遍历 `config` 数组:
```
for (let route of config) {
console.log(route.path);
}
config.forEach(route => {
console.log(route.path);
});
```
你也可以使用 JavaScript 的 `map()` 方法来对 `config` 数组中的每个元素进行操作:
```
let paths = config.map(route => route.path);
```
### 回答2:
要实现遍历Angular Router模块里的config对象,可以采用递归的方式进行遍历。
首先,我们可以在Angular应用的根模块中导入Router模块,并获取其配置对象。代码如下所示:
```typescript
import { RouterModule, Routes, Router } from '@angular/router';
export class AppModule {
constructor(private router: Router) {
// 获取Router模块的配置对象
const config = router.config;
// 调用遍历方法,从根路由进行递归遍历
this.traverseRoutes(config);
}
// 遍历路由配置方法
private traverseRoutes(routes: Routes) {
for (const route of routes) {
// 打印当前路由配置对象
console.log(route);
// 判断当前路由是否有子路由配置
if (route.children) {
// 递归调用遍历方法,继续遍历子路由
this.traverseRoutes(route.children);
}
}
}
}
```
在以上代码中,首先通过注入Router对象来获取Router模块的配置对象,然后调用遍历方法traverseRoutes来遍历路由配置。遍历方法采用递归的方式,首先对传入的routes进行迭代处理,打印当前路由配置对象,并判断是否存在子路由配置,若存在则递归调用遍历方法,继续遍历子路由。
通过以上方法,我们可以遍历Angular Router模块里的config对象,并对每个路由配置进行处理。
### 回答3:
在Angular中,遍历`router`模块中的`config`对象可以使用如下方式实现:
```typescript
import { Routes, RouterModule } from '@angular/router';
function traverseRouterConfig(config: Routes) {
for (const route of config) {
// 处理当前路由配置
console.log(route);
// 递归遍历子路由配置
if (route.children) {
traverseRouterConfig(route.children);
}
}
}
// 假设已经定义了路由配置
const routes: Routes = [
// 路由配置项
{
path: 'example',
component: ExampleComponent,
children: [
// 子路由配置项
{ path: 'child', component: ChildComponent }
]
}
];
// 调用方法遍历路由配置
traverseRouterConfig(routes);
```
以上代码中,我们定义了一个`traverseRouterConfig`方法,该方法接受一个`config`对象作为参数,我们可以将`router`模块中的`config`对象传递给该方法进行遍历。在遍历过程中,我们首先处理当前路由配置,然后递归处理子路由配置(如果存在)。可以根据具体需要,修改方法内部的处理逻辑来满足不同的需求。
以上就是实现一个方法,遍历Angular `router`模块中的`config`对象的简单示例,根据实际需求可以进一步定制该方法。
阅读全文
相关推荐
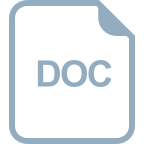
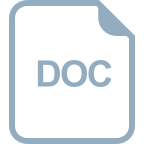
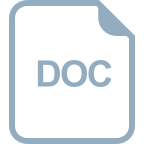

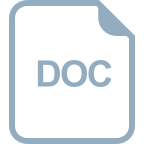
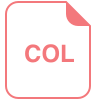


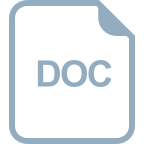
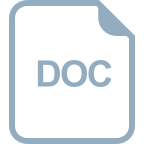
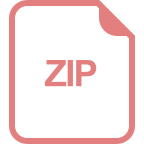
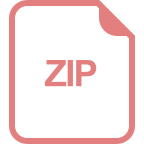
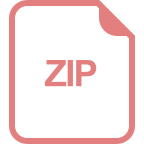
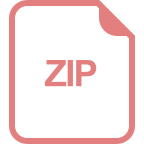
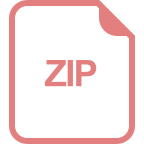
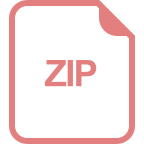
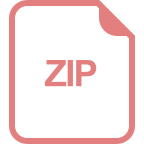