实现在angular 中递归 router.config
时间: 2023-09-15 08:05:26 浏览: 148
### 回答1:
在 Angular 中,你可以使用递归的方式来配置路由。
首先,你需要在路由配置数组中定义一个路由,其中包含一个 `children` 属性,该属性是一个由子路由配置对象组成的数组。例如:
```
const routes: Routes = [
{
path: '',
component: ParentComponent,
children: [
{
path: 'child1',
component: Child1Component
},
{
path: 'child2',
component: Child2Component
}
]
}
];
```
接下来,你可以在父组件的模板中使用 `<router-outlet>` 标签来渲染子组件,并且在子组件的模板中再次使用 `<router-outlet>` 标签来渲染更深层的子组件。例如:
```
<!-- 父组件模板 -->
<router-outlet></router-outlet>
<!-- 子组件模板 -->
<router-outlet></router-outlet>
```
这样,你就可以在 Angular 中使用递归的方式配置路由了。
### 回答2:
在Angular中实现递归路由配置可以通过创建一个递归的函数来完成。下面是一个示例:
首先,我们需要在路由模块中创建一个递归方法。这个方法会根据传入的参数构建并返回一个路由配置数组。
```typescript
import { Route } from '@angular/router';
function createRouteConfig(path: string): Route[] {
return [
{
path: path,
component: YourComponent,
children: createRouteConfig(path + '/child') // 递归调用方法以创建子路由配置
}
];
}
const routes: Route[] = createRouteConfig('your/path'); // 传入根路径,开始递归创建
```
在这个示例中,`createRouteConfig`方法接收一个`path`参数,该参数用于指定当前路由的路径。然后,我们返回一个包含当前路由配置的数组。
在路由配置中,我们在`children`属性里调用`createRouteConfig`方法,并传入带有子路由路径的新`path`,这样就能够递归地创建子路由配置。
最后,我们将返回的路由配置数组赋值给`routes`常量,以便在路由模块中使用。
请注意,上述示例是一个简单的示例,只是为了说明递归路由配置的原理。根据实际需求,你可能需要根据具体的场景进行更加复杂的逻辑处理。
### 回答3:
在Angular中实现递归的router.config,可以通过创建一个递归函数来实现。首先,我们需要在项目的根组件中导入Router和RouterModule,并将其作为构造函数的参数。
然后,我们可以创建一个递归函数,该函数的参数是一个路由数组和一个父级路由路径。在函数内部,我们首先遍历路由数组,然后判断每个路由对象是否有children属性。
如果有children属性,我们可以通过调用递归函数,并将children数组和当前路由路径作为参数传递给它,来处理它的子路由。递归函数将返回一个包含子路由配置的路由数组。
接下来,我们可以使用forEach()方法来遍历这个返回的子路由数组,并将每个子路由对象的路径修改为父级路径加上子路由路径的形式。然后,我们可以通过调用router.config()方法来配置这些路由。
最后,我们可以在根组件的ngOnInit()函数中调用递归函数,并传递顶层路由数组和空字符串作为参数,来递归地配置路由。
这样,我们就实现了在Angular中递归地配置路由。通过这种方式,我们可以轻松地处理具有多层嵌套的路由配置,提供更灵活和可扩展的应用程序结构。
以下是一个示例代码:
```typescript
import { Component, OnInit } from '@angular/core';
import { Router, RouterModule, Routes } from '@angular/router';
@Component({
selector: 'app-root',
templateUrl: './app.component.html',
styleUrls: ['./app.component.css']
})
export class AppComponent implements OnInit {
constructor(private router: Router) {}
ngOnInit() {
const routes: Routes = [
{
path: '',
component: HomeComponent
},
{
path: 'about',
component: AboutComponent,
children: [
{
path: 'overview',
component: OverviewComponent
},
{
path: 'features',
component: FeaturesComponent
},
{
path: 'pricing',
component: PricingComponent
}
]
}
];
const recursiveConfig = (routes: Routes, parentPath: string = '') => {
routes.forEach(route => {
if (route.children) {
const childRoutes = recursiveConfig(route.children, parentPath + route.path + '/');
route.children = childRoutes;
}
});
return routes.map(route => ({
...route,
path: parentPath + route.path
}));
};
const configuredRoutes = recursiveConfig(routes);
this.router.config = configuredRoutes;
}
}
```
这是一个简单的示例,但你可以根据你的实际需求进行更复杂的路由配置。希望这能帮助到你!
相关推荐
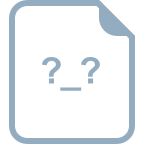
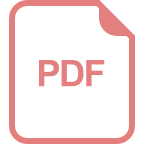
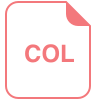













