一元线性回归的回归参数和迭代次数变化曲线
时间: 2024-04-20 21:04:13 浏览: 9
一元线性回归的回归参数是指模型中的斜率和截距,它们是通过最小化残差平方和来确定的。迭代次数是指用梯度下降等优化算法来求解回归参数时,进行更新的次数。
回归参数和迭代次数的变化曲线可以通过训练数据来得到。在每一次迭代中,根据当前的回归参数,计算出预测值与真实值之间的误差,然后根据梯度下降等优化算法更新回归参数。可以记录下每一次迭代后的回归参数和误差,然后绘制出它们随迭代次数变化的曲线。
下面是一个简单的示例代码,展示了如何绘制一元线性回归的回归参数和迭代次数变化曲线(假设使用梯度下降算法):
```python
import numpy as np
import matplotlib.pyplot as plt
# 定义线性回归模型
def linear_regression(x, y, alpha=0.01, num_iters=100):
m = len(y)
theta0 = 0
theta1 = 0
J_history = []
for i in range(num_iters):
h = theta0 + theta1 * x
theta0 -= alpha * (1/m) * np.sum(h-y)
theta1 -= alpha * (1/m) * np.sum((h-y)*x)
J_history.append(compute_cost(x, y, theta0, theta1))
return theta0, theta1, J_history
# 计算代价函数
def compute_cost(x, y, theta0, theta1):
m = len(y)
h = theta0 + theta1 * x
J = (1/(2*m)) * np.sum(np.square(h-y))
return J
# 生成随机数据
np.random.seed(0)
x = np.random.rand(100)
y = 2 * x + 1 + 0.2 * np.random.randn(100)
# 训练模型
theta0, theta1, J_history = linear_regression(x, y, alpha=0.01, num_iters=100)
# 绘制代价函数随迭代次数变化的曲线
plt.plot(J_history)
plt.xlabel('Number of iterations')
plt.ylabel('Cost J')
plt.show()
# 绘制回归线和散点图
plt.scatter(x, y)
plt.plot(x, theta0 + theta1 * x, 'r')
plt.xlabel('x')
plt.ylabel('y')
plt.show()
```
上述代码中,首先定义了一个线性回归模型,并使用梯度下降算法进行训练。在每一次迭代中,记录下当前的代价函数值,最终绘制出代价函数随迭代次数变化的曲线。同时,也绘制出回归线和散点图,以展示模型的拟合效果。
相关推荐
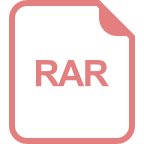














