Ping::Ping(QObject* parent) : QObject(parent), failCount(0) { process = new QProcess(this); timer = new QTimer(this); connect(timer, SIGNAL(timeout()), this, SLOT(onTimeout())); connect(process, SIGNAL(readyReadStandardOutput()), this, SLOT(onReadyReadStandardOutput())); connect(process, SIGNAL(readyReadStandardError()), this, SLOT(onReadyReadStandardError())); } void Ping::startPing(QString ipAddress) { // Stop the ping command if it's running stopPing(); // Clear fail counter failCount = 0; // Start the ping command with appropriate arguments this->ipAddress = ipAddress; QStringList arguments; qDebug()<<"ip"<<ipAddress<<this->ipAddress; arguments << "-n" << "1" << "-w" << "1000" << ipAddress; process->start("ping", arguments); // arguments<< "-a" << ipAddress; // process->start("arp", arguments); // Start the timer to repeatedly send the ping command timer->start(1000); // ping every 1 second } void Ping::stopPing() { // Stop the ping command process->kill(); process->waitForFinished(); // Stop the timer timer->stop(); } void Ping::onTimeout() { failCount++; if (failCount >= 3) { QString macAddress = ""; emit deviceDisconnected(ipAddress, macAddress); stopPing(); } else { onPing(); } } void Ping::onPing() { // Write a newline to the ping process to send another ping //process->write("\n"); QStringList arguments; arguments << "-n" << "1" << "-w" << "1000" << ipAddress; process->start("ping", arguments); } void Ping::onReadyReadStandardOutput() { // Extract the MAC address from the standard output of the ping command process->waitForFinished(); QByteArray output(process->readAllStandardOutput()); QString str = QString::fromLocal8Bit(output); //qDebug()<<"???????"<<str<<failCount; // QRegularExpression macRegexp(R"(([\da-fA-F]{2}[:-]){5}([\da-fA-F]{2}))"); // QRegularExpressionMatch match = macRegexp.match(output); if (str.contains("丢失 = 0")) { //qDebug()<<"!!!!!"<<str<<failCount; //QString macAddress = match.captured(0).toUpper(); // Check if MAC address has changed // if (macAddress != lastMacAddress) { // lastMacAddress = macAddress; emit deviceConnected(ipAddress, ""); // } // Reset fail counter failCount = 0; } } void Ping::onReadyReadStandardError() { // Output the standard error of the ping command to the console QString output(process->readAllStandardError()); qDebug()<<"errormessage" << output; }优化这段代码
时间: 2024-04-01 19:32:55 浏览: 96
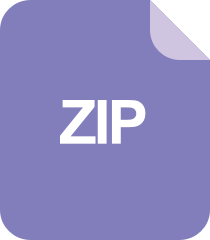
vlcplayer02.zip,vlc库函数的使用(一):单视频文件播放
1. 建议将 Ping 类中的变量和方法进行私有化,并添加相应的访问函数。
2. 在 startPing 函数中,每次启动进程时都创建了新的 QStringList 对象,可以将其定义为成员变量,避免重复创建。
3. 在 onTimeout 函数中,failCount 的更新应该是 failCount++ 而不是 failCount。同时建议将 magic number 3 定义为常量。
4. 在 onPing 函数中,每次启动进程时都创建了新的 QStringList 对象,可以将其定义为成员变量,避免重复创建。
5. 在 onReadyReadStandardOutput 函数中,建议使用正则表达式来提取 MAC 地址,而不是简单的字符串匹配。
6. 在 onReadyReadStandardOutput 函数中,由于 process->waitForFinished() 会阻塞应用程序的执行,建议将其移动到函数的开头。
7. 在 onReadyReadStandardOutput 函数中,由于输出可能跨越多行,建议使用 process->readLine() 逐行读取输出,以便更好地匹配正则表达式。
8. 在 onReadyReadStandardOutput 函数中,建议使用 QString 的 contains 函数来判断是否能够成功 ping 通,而不是简单的字符串匹配。
阅读全文
相关推荐
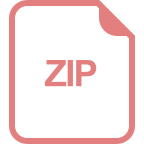
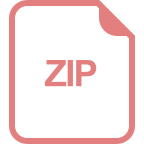
















