#include <QObject> #include <QTcpServer> #include <QTcpSocket> class TcpProxy : public QObject { Q_OBJECT public: explicit TcpProxy(QObject *parent = nullptr); signals: public slots: void onNewClientConnection(); void onClientDataReady(); void onServerDataReady(); private: QTcpServer *m_server; QTcpSocket *m_client1; QTcpSocket *m_client2; QTcpSocket *m_serverSocket; }; TcpProxy::TcpProxy(QObject *parent) : QObject(parent) { m_server = new QTcpServer(this); m_server->listen(QHostAddress::Any, 1234); connect(m_server, SIGNAL(newConnection()), this, SLOT(onNewClientConnection())); } void TcpProxy::onNewClientConnection() { if (!m_client1) { m_client1 = m_server->nextPendingConnection(); connect(m_client1, SIGNAL(readyRead()), this, SLOT(onClientDataReady())); qDebug() << "Client 1 connected"; } else if (!m_client2) { m_client2 = m_server->nextPendingConnection(); connect(m_client2, SIGNAL(readyRead()), this, SLOT(onClientDataReady())); qDebug() << "Client 2 connected"; } if (m_client1 && m_client2 && !m_serverSocket) { m_serverSocket = new QTcpSocket(this); connect(m_serverSocket, SIGNAL(readyRead()), this, SLOT(onServerDataReady())); m_serverSocket->connectToHost("localhost", 4321); // replace with your down-level machine's IP address and port number qDebug() << "Server connected"; } } void TcpProxy::onClientDataReady() { QTcpSocket *senderSocket = qobject_cast<QTcpSocket*>(sender()); QTcpSocket *destSocket = (senderSocket == m_client1) ? m_client2 : m_client1; QByteArray data = senderSocket->readAll(); destSocket->write(data); if (m_serverSocket) { m_serverSocket->write(data); } } void TcpProxy::onServerDataReady() { QByteArray data = m_serverSocket->readAll(); if (m_client1) { m_client1->write(data); } if (m_client2) { m_client2->write(data); } } int main(int argc, char *argv[]) { QCoreApplication a(argc, argv); TcpProxy tcpProxy; return a.exec(); }为什么两台电脑无法互通数据
时间: 2024-04-01 16:36:35 浏览: 141
这段代码是一个 TCP 代理程序,主要实现了两个客户端与一个服务器的数据交互。如果两台电脑无法互通数据,可能是以下几个原因导致的:
1. 网络配置问题:确保两台电脑都处于同一个局域网内,并且 IP 地址和子网掩码设置正确。
2. 防火墙问题:确保防火墙没有阻止程序的网络通信。可以尝试关闭防火墙或添加程序的网络访问权限。
3. 端口号问题:确保代理程序监听的端口号(1234)和服务器连接的端口号(4321)都没有被其他程序占用。
如果以上问题都没有解决,还需要进一步排查程序代码是否存在问题。
相关问题
多线程下tcp发数据,将发送的数据通过Qvetor<T>发送数据出去,其中T是自定义类型。,主线程收数据,收到的数据放入QMap<QString,QVctor<T>>中,根据不同的QString和T类型分类数据,比较同一个Map中数据的一致性,请用qt写一段代码,代码包含.h和.cpp
以下是一个简单的多线程TCP通信的例子,其中发送的数据类型为自定义类型MyData,接收到的数据存储在QMap<QString, QVector<MyData>>中,主线程进行数据分类和一致性比较。
在头文件中定义以下内容:
```cpp
#ifndef TCPCOMMUNICATION_H
#define TCPCOMMUNICATION_H
#include <QObject>
#include <QTcpServer>
#include <QTcpSocket>
#include <QThread>
#include <QVector>
#include <QMap>
struct MyData {
QString name;
int value;
};
class TcpCommunication : public QObject
{
Q_OBJECT
public:
explicit TcpCommunication(QObject *parent = nullptr);
void start();
signals:
public slots:
void newConnection();
void readData();
void sendData();
private:
QTcpServer *m_server;
QTcpSocket *m_socket;
QVector<MyData> m_dataToSend;
QMap<QString, QVector<MyData>> m_dataReceived;
};
#endif // TCPCOMMUNICATION_H
```
在源文件中实现以下内容:
```cpp
#include "tcpcommunication.h"
TcpCommunication::TcpCommunication(QObject *parent) : QObject(parent)
{
m_server = new QTcpServer(this);
m_socket = new QTcpSocket(this);
connect(m_server, SIGNAL(newConnection()), this, SLOT(newConnection()));
connect(m_socket, SIGNAL(readyRead()), this, SLOT(readData()));
connect(m_socket, SIGNAL(disconnected()), m_socket, SLOT(deleteLater()));
}
void TcpCommunication::start()
{
if (m_server->listen(QHostAddress::Any, 1234)) {
qDebug() << "Server started";
} else {
qDebug() << "Server failed to start";
}
}
void TcpCommunication::newConnection()
{
m_socket = m_server->nextPendingConnection();
}
void TcpCommunication::readData()
{
QByteArray data = m_socket->readAll();
QDataStream stream(&data, QIODevice::ReadOnly);
while (!stream.atEnd()) {
MyData newData;
stream >> newData.name >> newData.value;
m_dataReceived[newData.name].append(newData);
}
}
void TcpCommunication::sendData()
{
QByteArray data;
QDataStream stream(&data, QIODevice::WriteOnly);
for (const auto& newData : m_dataToSend) {
stream << newData.name << newData.value;
}
m_socket->write(data);
}
```
在主函数中创建一个TcpCommunication对象,使用QThread将其移动到子线程中运行:
```cpp
#include <QCoreApplication>
#include <QThread>
#include "tcpcommunication.h"
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
TcpCommunication tcpCommunication;
QThread thread;
tcpCommunication.moveToThread(&thread);
QObject::connect(&thread, SIGNAL(started()), &tcpCommunication, SLOT(start()));
QObject::connect(&tcpCommunication, SIGNAL(finished()), &thread, SLOT(quit()));
QObject::connect(&tcpCommunication, SIGNAL(finished()), &tcpCommunication, SLOT(deleteLater()));
QObject::connect(&thread, SIGNAL(finished()), &thread, SLOT(deleteLater()));
thread.start();
return a.exec();
}
```
多线程下tcp收数据,将收到的数据通过Qvetor<T>发送给主线程,其中T是自定义类型。主线程收数据,收到的数据放入QMap<QString,QVctor<T>>中,根据不同的QString和T类型分类数据,比较同一个Map中数据的一致性,思考一下,请用qt写一段代码,代码包含.h和.cpp。
以下是一个多线程TCP通信的例子,其中接收到的数据类型为自定义类型MyData,收到的数据存储在QMap<QString, QVector<MyData>>中,主线程进行数据分类和一致性比较。发送的数据通过QVector<MyData>发送给子线程。
在头文件中定义以下内容:
```cpp
#ifndef TCPCOMMUNICATION_H
#define TCPCOMMUNICATION_H
#include <QObject>
#include <QTcpServer>
#include <QTcpSocket>
#include <QThread>
#include <QVector>
#include <QMap>
struct MyData {
QString name;
int value;
};
class TcpCommunication : public QObject
{
Q_OBJECT
public:
explicit TcpCommunication(QObject *parent = nullptr);
void start();
signals:
void newDataReceived(const QString& name, const QVector<MyData>& data);
public slots:
void newConnection();
void readData();
void sendData(const QVector<MyData>& data);
private:
QTcpServer *m_server;
QTcpSocket *m_socket;
QMap<QString, QVector<MyData>> m_dataReceived;
};
#endif // TCPCOMMUNICATION_H
```
在源文件中实现以下内容:
```cpp
#include "tcpcommunication.h"
TcpCommunication::TcpCommunication(QObject *parent) : QObject(parent)
{
m_server = new QTcpServer(this);
m_socket = new QTcpSocket(this);
connect(m_server, SIGNAL(newConnection()), this, SLOT(newConnection()));
connect(m_socket, SIGNAL(readyRead()), this, SLOT(readData()));
connect(m_socket, SIGNAL(disconnected()), m_socket, SLOT(deleteLater()));
}
void TcpCommunication::start()
{
if (m_server->listen(QHostAddress::Any, 1234)) {
qDebug() << "Server started";
} else {
qDebug() << "Server failed to start";
}
}
void TcpCommunication::newConnection()
{
m_socket = m_server->nextPendingConnection();
}
void TcpCommunication::readData()
{
QByteArray data = m_socket->readAll();
QDataStream stream(&data, QIODevice::ReadOnly);
QVector<MyData> newDataVector;
while (!stream.atEnd()) {
MyData newData;
stream >> newData.name >> newData.value;
newDataVector.append(newData);
}
emit newDataReceived(newDataVector);
}
void TcpCommunication::sendData(const QVector<MyData>& data)
{
QByteArray dataToSend;
QDataStream stream(&dataToSend, QIODevice::WriteOnly);
for (const auto& newData : data) {
stream << newData.name << newData.value;
}
m_socket->write(dataToSend);
}
```
在主函数中创建一个TcpCommunication对象,使用QThread将其移动到子线程中运行,并且将接收到的数据放入QMap<QString, QVector<MyData>>中:
```cpp
#include <QCoreApplication>
#include <QThread>
#include "tcpcommunication.h"
int main(int argc, char *argv[])
{
QCoreApplication a(argc, argv);
QMap<QString, QVector<MyData>> dataMap;
TcpCommunication tcpCommunication;
QThread thread;
tcpCommunication.moveToThread(&thread);
QObject::connect(&thread, SIGNAL(started()), &tcpCommunication, SLOT(start()));
QObject::connect(&tcpCommunication, &TcpCommunication::newDataReceived, [&](const QString& name, const QVector<MyData>& data){
dataMap[name] = data;
});
QObject::connect(&tcpCommunication, SIGNAL(finished()), &thread, SLOT(quit()));
QObject::connect(&tcpCommunication, SIGNAL(finished()), &tcpCommunication, SLOT(deleteLater()));
QObject::connect(&thread, SIGNAL(finished()), &thread, SLOT(deleteLater()));
thread.start();
return a.exec();
}
```
阅读全文
相关推荐
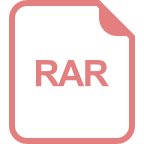
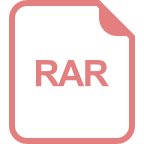
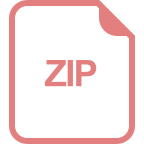













