C语言字符串如何替换单词
时间: 2024-09-30 08:05:43 浏览: 4
在C语言中,字符串的操作通常是字符数组处理,如果需要替换字符串中的某个单词,可以分为以下几个步骤:
1. **定位单词**: 首先找到要替换的单词的位置。通常这涉及到遍历字符串,通过空格或特定分隔符将字符串分割成单词数组。
```c
char str[] = "Hello world, this is a test.";
int pos = 0; // 初始化位置指针
while (str[pos] != '\0') { // 找到单词边界
if (str[pos] == ' ') {
// 如果遇到空格,检查前一个单词是否就是目标
if (prev_word != NULL) {
// 替换操作...
}
prev_word = str + pos; // 更新上一个单词的位置
} else {
prev_word = str + pos;
}
pos++;
}
```
2. **替换操作**: 当找到要替换的单词(`prev_word`)时,你可以创建一个新的字符串,并将新内容插入进去。需要注意的是,如果你打算用新的单词替换整个单词,那么从原单词的起始位置复制到结束位置即可。
```c
char new_word[] = "replaced"; // 新单词
size_t old_length = strlen(prev_word);
size_t new_length = strlen(new_word);
// 移除旧单词并添加新单词
memmove(str + pos + new_length, str + pos, strlen(str) - pos); // 移动后续字符
strcpy(str + pos, new_word); // 插入新单词
```
3. **更新结束标志**: 调整字符串的终止符 `\0` 以匹配新字符串的长度。
```c
str[strlen(str)] = '\0'; // 确保最后一个字符仍为'\0'
```
相关推荐
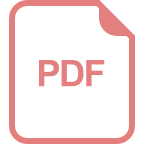
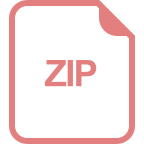
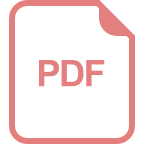














