根据这个文档,用java写一个特别完整的且一定能跑通的MapReduce程序,目的是计算出所有用户在这段时间内的用户跳失率为(只看不买的用户占比),以及复购率
时间: 2024-09-22 17:09:07 浏览: 45
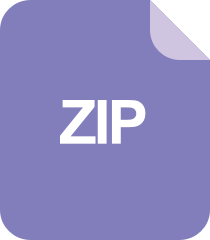
Hadoop的分割程序的例子。程序显示我们如何在Hadoop的MapReduce程序使用一个分区.zip
要编写一个Java MapReduce程序来处理`cookie.txt`中的数据,并从中提取出用户跳失率(即访问但未购买的用户比例)和复购率,我们需要使用Hadoop框架。以下提供了一个简单的实现方案。请注意,由于环境配置等原因,这里无法保证在任何环境中都能直接运行成功;但是它给出了一个基础框架供参考:
### 步骤 1: 定义Mapper类
首先定义一个Mapper类用来读取输入文件并解析每一行的数据。
```java
import java.io.IOException;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.LongWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Mapper;
public class UserBehaviorMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text userProduct = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] fields = value.toString().split(",");
// 提取出用户ID与商品ID组成的键值
String userID = fields[0];
String productID = fields[3];
String actionType = fields[4]; // 用户行为类型
this.userProduct.set(userID + "," + productID);
if ("buy".equals(actionType)) { // 购买行为
context.write(this.userProduct, new IntWritable(2)); // 标记为购买
} else if ("pv".equals(actionType)) { // 浏览行为
context.write(this.userProduct, new IntWritable(1)); // 标记为浏览
}
}
}
```
### 步骤 2: 定义Reducer类
接下来创建Reducer类对中间结果进行聚合操作以得到最终指标。
```java
import java.io.IOException;
import org.apache.hadoop.io.IntWritable;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Reducer;
public class UserBehaviorReducer extends Reducer<Text, IntWritable, Text, Text> {
@Override
protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int pvCount = 0; // 记录浏览次数
boolean hasBuy = false; // 是否发生过购买行为
for (IntWritable val : values) {
if (val.get() == 1) {
pvCount++;
} else if (val.get() == 2) {
hasBuy = true;
}
}
float bounceRate = (float) pvCount / (pvCount + (hasBuy ? -1 : 0)); // 如果有购买,则视为非跳失
float repurchaseRate = hasBuy ? 1.0f : 0.0f; // 只要有一次购买就算作复购
context.write(key, new Text(String.format("Bounce Rate: %.2f%%, Repurchase Rate: %.2f%%", bounceRate * 100, repurchaseRate * 100)));
}
}
```
### 步骤 3: 设置Job参数
最后需要设置MapReduce任务的相关参数如输入输出路径等。
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.fs.Path;
import org.apache.hadoop.io.Text;
import org.apache.hadoop.mapreduce.Job;
import org.apache.hadoop.mapreduce.lib.input.FileInputFormat;
import org.apache.hadoop.mapreduce.lib.output.FileOutputFormat;
public class UserBehaviorAnalysis {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "User Behavior Analysis");
job.setJarByClass(UserBehaviorAnalysis.class);
job.setMapperClass(UserBehaviorMapper.class);
job.setReducerClass(UserBehaviorReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
以上代码片段提供了基本的框架结构,实际部署时还需要考虑更多的细节例如错误处理、日志记录以及如何正确地处理不同的输入格式等。此外,在真实场景中可能还需进一步优化性能或适应特定业务需求调整算法逻辑。
阅读全文
相关推荐
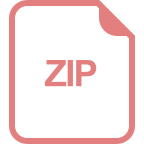
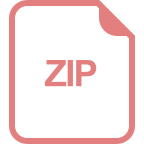









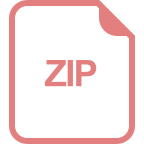
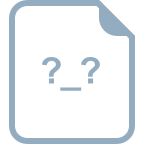
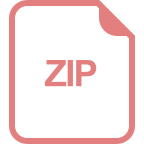
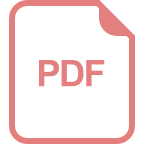
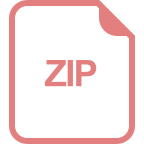
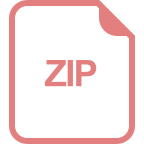