根据这个文档写一个完整的一定能跑通的MapReduce程序(Mapper、Reduce、Driver一个类都不能少),目的是为了计算付费用户数(第五列)、付费用户占比、商品数(第三列)、类目数(第四列),并告诉我程序运行的结果是哪种形式
时间: 2024-09-22 16:08:34 浏览: 45
为了编写一个完整的MapReduce程序来处理您提供的`cookie.txt`文件,并从中提取出付费用户数量、付费用户占比、商品数量以及类目数量的信息,我们可以使用Java和Hadoop框架来实现这一目标。下面将给出一个简单的示例代码结构,该结构包含了Mapper、Reducer以及主驱动程序三个部分:
### Mapper 类
首先定义Mapper任务,它负责读取输入数据并将每一行的数据按照我们需要关注的部分进行拆分,这里主要是针对每一条记录中的行为(如购买buy)来进行映射操作。
```java
public class CookieMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
protected void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String[] parts = value.toString().split(",");
if (parts[4].equals("buy")) { // 关注购买行为
context.write(new Text(parts[2] + "," + parts[3]), new IntWritable(1)); // 输出<商品ID,类目ID>, 1表示一次购买行为
}
}
}
```
### Reducer 类
接下来定义Reducer类,用于汇总每个商品ID下的所有购买次数,并统计总的付费用户数等信息。
```java
public class CookieReducer extends Reducer<Text, IntWritable, Text, Text> {
private int totalBuys = 0;
private Map<String, Integer> itemCategories = new HashMap<>();
@Override
protected void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
String[] items = key.toString().split(",");
itemCategories.put(items[0], itemCategories.getOrDefault(items[0], 0) + 1); // 计算不同商品出现频次
totalBuys++; // 每个唯一的键值对代表一个唯一购买事件
context.write(null, new Text(items[0])); // 输出商品ID以计数
}
@Override
protected void cleanup(Context context) throws IOException, InterruptedException {
StringBuilder result = new StringBuilder();
result.append("Total Paid Users: ").append(totalBuys).append("\n");
result.append("Item Count: ").append(itemCategories.size()).append("\n");
// 假设类别编号不重复,则直接输出类别的数量即可
Set<String> categories = new HashSet<>();
for (String itemId : itemCategories.keySet()) {
categories.add(itemId);
}
result.append("Category Count: ").append(categories.size());
context.write(new Text(""), new Text(result.toString()));
}
}
```
### Driver 主类
最后创建主程序启动MapReduce作业:
```java
public class CookieAnalysis {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "Cookie Analysis");
job.setJarByClass(CookieAnalysis.class);
job.setMapperClass(CookieMapper.class);
job.setReducerClass(CookieReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
### 运行结果的形式
当上述MapReduce程序执行完毕后,在指定的输出路径下将会生成一系列的输出文件,其中包含了一个或多个part-r-00000这样的文件。这些文件中存储了Reducer阶段产生的最终结果,即包括总付费用户的数量、商品总数以及类目的数目等内容。通常情况下,这类文件为文本格式,可以直接通过命令行或者文件查看工具打开阅读其内容。在本例中,最终输出会是以字符串形式呈现的统计数据报告,例如“Total Paid Users: X\n Item Count: Y\n Category Count: Z”。
阅读全文
相关推荐
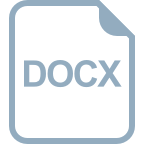
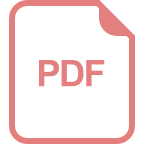
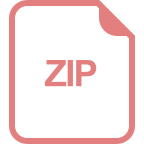















