Mapper 类Reducer 类 Driver 类怎么写
时间: 2024-03-22 15:36:56 浏览: 23
Mapper 类、Reducer 类和 Driver 类是 Hadoop MapReduce 中的三个重要组件。下面是它们的基本代码实现:
Mapper 类:
```java
public class MyMapper extends Mapper<LongWritable, Text, Text, IntWritable> {
private final static IntWritable one = new IntWritable(1);
private Text word = new Text();
public void map(LongWritable key, Text value, Context context) throws IOException, InterruptedException {
String line = value.toString();
String[] words = line.split(" ");
for (String w : words) {
word.set(w);
context.write(word, one);
}
}
}
```
Reducer 类:
```java
public class MyReducer extends Reducer<Text, IntWritable, Text, IntWritable> {
public void reduce(Text key, Iterable<IntWritable> values, Context context) throws IOException, InterruptedException {
int sum = 0;
for (IntWritable val : values) {
sum += val.get();
}
context.write(key, new IntWritable(sum));
}
}
```
Driver 类:
```java
public class MyDriver {
public static void main(String[] args) throws Exception {
Configuration conf = new Configuration();
Job job = Job.getInstance(conf, "word count");
job.setJarByClass(MyDriver.class);
job.setMapperClass(MyMapper.class);
job.setCombinerClass(MyReducer.class);
job.setReducerClass(MyReducer.class);
job.setOutputKeyClass(Text.class);
job.setOutputValueClass(IntWritable.class);
FileInputFormat.addInputPath(job, new Path(args[0]));
FileOutputFormat.setOutputPath(job, new Path(args[1]));
System.exit(job.waitForCompletion(true) ? 0 : 1);
}
}
```
以上代码实现了一个简单的 WordCount 示例,其中 MyMapper 类和 MyReducer 类分别实现了 Mapper 和 Reducer 接口,并重写了 map 和 reduce 方法。MyDriver 类则是程序的入口,它配置了 Job 的各种参数,并提交运行任务。
相关推荐
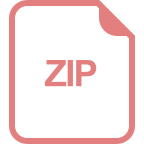
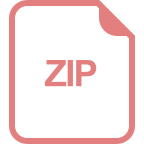
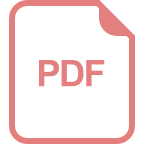














